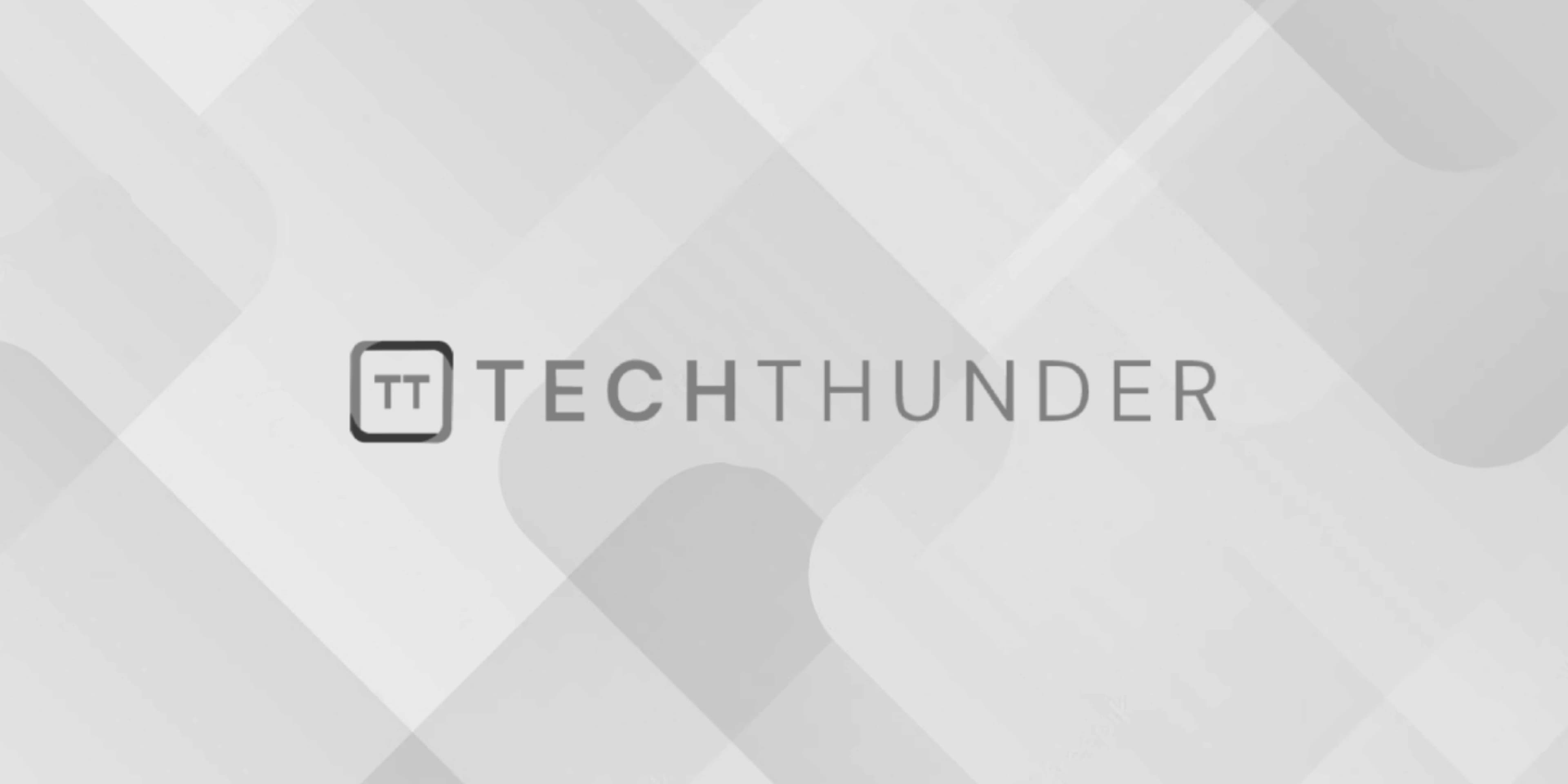
224 views
PHP Array Functions
PHP provides a variety of built-in array functions that allow you to perform various operations on arrays. Here are some of the commonly used PHP array functions:
count()
:
This function is used to count the number of elements in an array.
$numbers = [1, 2, 3, 4, 5];
echo count($numbers); // Output: 5
array_push()
andarray_pop()
:array_push()
is used to add one or more elements to the end of an array, andarray_pop()
is used to remove the last element from an array.
$fruits = ["Apple", "Banana"];
array_push($fruits, "Orange", "Mango");
print_r($fruits); // Output: Array ( [0] => Apple [1] => Banana [2] => Orange [3] => Mango )
$lastFruit = array_pop($fruits);
echo $lastFruit; // Output: Mango
array_shift()
andarray_unshift()
:array_shift()
is used to remove the first element from an array and return it, whilearray_unshift()
is used to add one or more elements to the beginning of an array.
$numbers = [1, 2, 3, 4, 5];
$firstNumber = array_shift($numbers);
echo $firstNumber; // Output: 1
array_unshift($numbers, 0);
print_r($numbers); // Output: Array ( [0] => 0 [1] => 2 [2] => 3 [3] => 4 [4] => 5 )
array_merge()
:
This function is used to merge two or more arrays into a single array.
$fruits1 = ["Apple", "Banana"];
$fruits2 = ["Orange", "Mango"];
$allFruits = array_merge($fruits1, $fruits2);
print_r($allFruits); // Output: Array ( [0] => Apple [1] => Banana [2] => Orange [3] => Mango )
array_search()
:
This function is used to search for a value in an array and return its corresponding key if found.
$fruits = ["Apple", "Banana", "Orange"];
$key = array_search("Banana", $fruits);
echo $key; // Output: 1
in_array()
:
This function checks if a value exists in an array and returnstrue
if found, orfalse
otherwise.
$fruits = ["Apple", "Banana", "Orange"];
if (in_array("Orange", $fruits)) {
echo "Orange is in the array.";
} else {
echo "Orange is not in the array.";
}
These are just a few examples of the many useful array functions available in PHP. Other array functions like array_slice()
, array_filter()
, array_map()
, and array_reduce()
are also commonly used for array manipulation and data processing tasks. You can refer to the official PHP documentation for a comprehensive list of array functions and their usages.