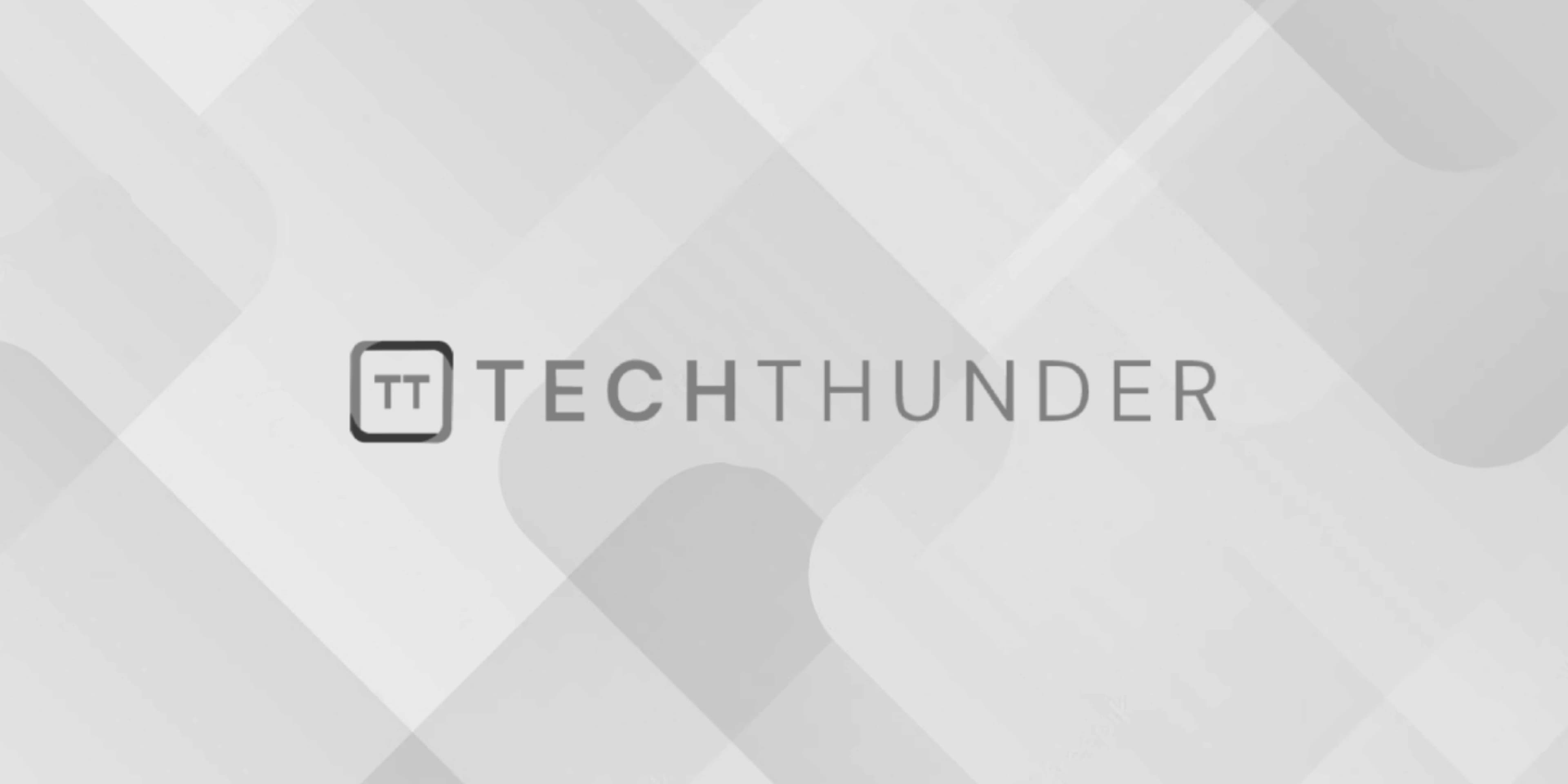
PHP Write File
In PHP, you can use the file_put_contents()
function to write data to a file. The file_put_contents()
function is a convenient way to write content to a file in a single line of code. It can create a new file if it doesn’t exist or overwrite the content of an existing file. Here’s how to use file_put_contents()
:
<?php
$file = 'path/to/your/file.txt'; // Replace this with the actual path and filename of the file you want to write.
$data = "This is the content that will be written to the file.\n";
$data .= "You can include multiple lines of text using the concatenation operator.\n";
// Write data to the file
if (file_put_contents($file, $data) !== false) {
echo "Data written to the file successfully.";
} else {
echo "Error writing to the file.";
}
?>
In this example, replace 'path/to/your/file.txt'
with the actual path and filename where you want to write the data. The $data
variable contains the content that will be written to the file. The \n
characters are used to add line breaks between the lines of text.
The file_put_contents()
function returns the number of bytes written to the file on success or false
on failure. If the file doesn’t exist, file_put_contents()
will create a new file and write the data to it. If the file already exists, it will overwrite the existing content with the new data.
Remember to ensure that the directory where you want to write the file has the appropriate permissions to allow PHP to write to it. Additionally, be cautious when using user input to write to files to prevent security vulnerabilities like path traversal attacks. Always validate and sanitize user input before writing it to a file.