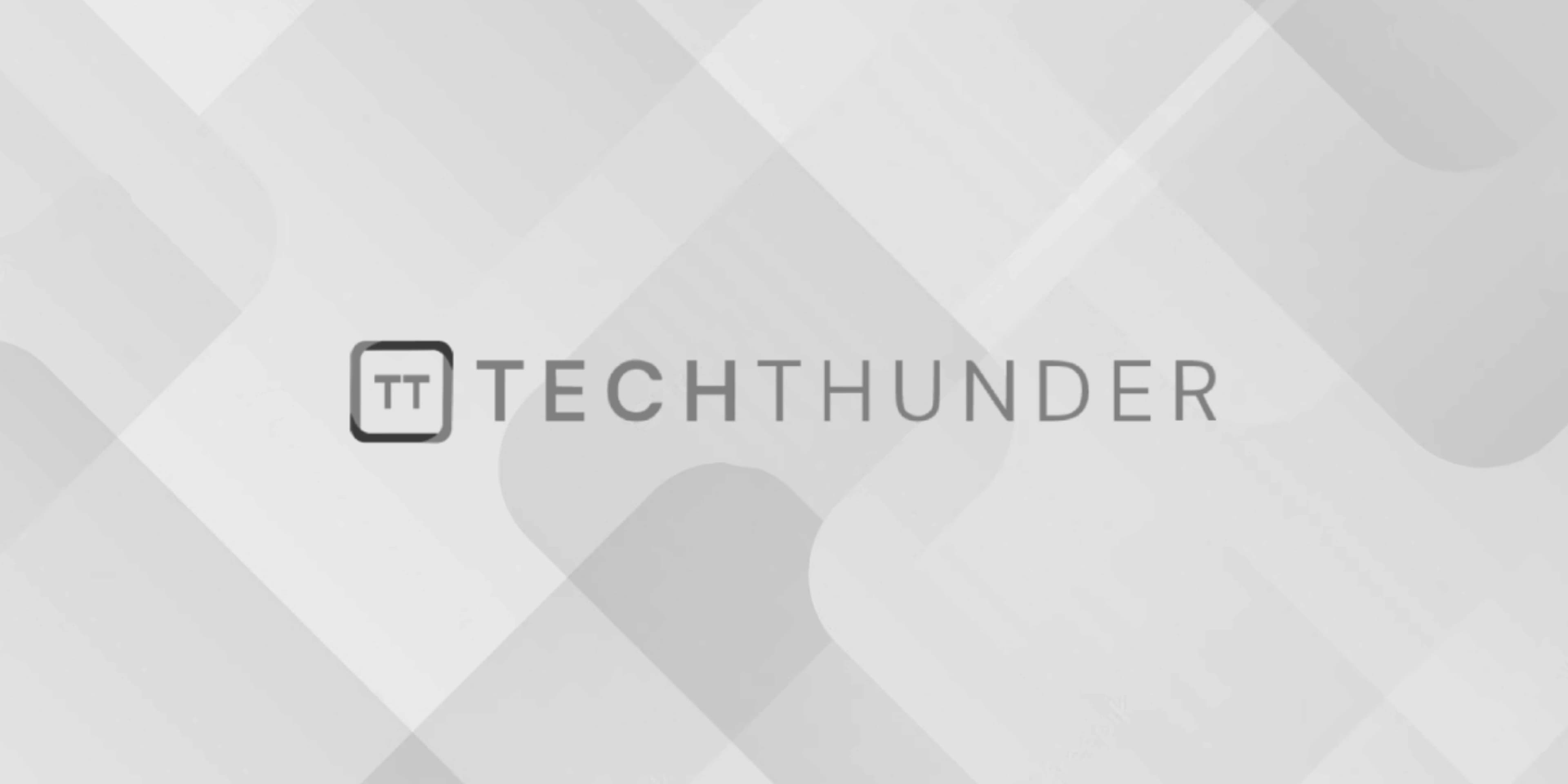
PHP Call By Value
In PHP, function parameters are typically passed by value by default, which means that a copy of the variable’s value is passed to the function. When you pass a variable as an argument to a function, any changes made to the parameter within the function do not affect the original variable outside the function.
Let’s see how call by value works in PHP:
function increment($num) {
$num++;
return $num;
}
$number = 5;
$result = increment($number);
echo $number; // Output: 5
echo $result; // Output: 6
In the example above, we define a function increment
that takes a parameter $num
. Inside the function, we increment the value of $num
by one using the $num++
notation. However, when we call the increment
function with $number
as an argument, the original value of $number
remains unchanged. The function works on a copy of the value of $number
, and the changes made within the function do not affect the variable outside the function.
In PHP, if you want to modify the original variable from within the function, you need to use the “call by reference” technique. This is done by using the ampersand (&
) symbol before the parameter name in both the function declaration and the function call. This way, you are passing a reference to the original variable instead of a copy, allowing the function to directly modify the original variable.
Example of PHP Call By Reference:
function incrementByReference(&$num) {
$num++;
}
$number = 5;
incrementByReference($number);
echo $number; // Output: 6
In this example, the incrementByReference
function is defined with the parameter $num
passed by reference using the &$num
notation. When we call the function with $number
as an argument, it directly modifies the value of $number
, and the change is reflected outside the function.
Keep in mind that using call by reference should be done carefully, as it can lead to unexpected side effects and make code harder to maintain. In most cases, call by value is sufficient for working with function parameters in PHP.