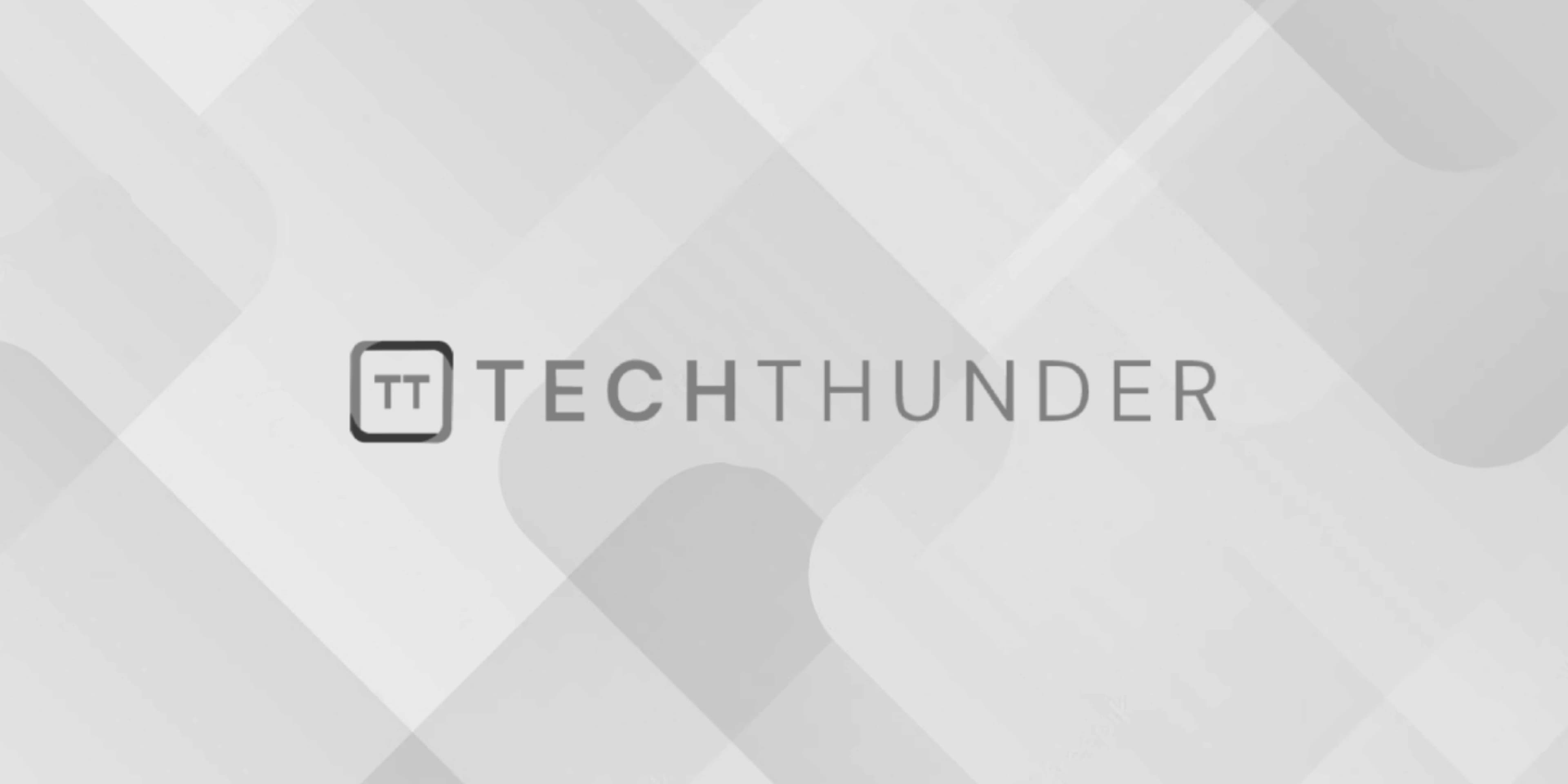
291 views
PHP Tutorial
Sure, I can provide you with a basic PHP tutorial to get started with the language. This tutorial assumes you have some basic programming knowledge. If you’re new to programming, it’s helpful to learn some fundamentals before diving into PHP.
- What is PHP?
- PHP (Hypertext Preprocessor) is a server-side scripting language widely used for web development.
- It’s embedded within HTML and executed on the web server, generating dynamic content for the web page.
- Setting Up a Local Development Environment
- To run PHP code locally, you need a web server (e.g., Apache) and PHP installed on your computer.
- Alternatively, you can use a package like XAMPP or WAMP, which includes Apache, PHP, MySQL, and more.
- Basic PHP Syntax
- PHP code is enclosed within
<?php
and?>
tags. - PHP statements end with a semicolon
;
.
- Outputting Content
- To display content in PHP, use the
echo
orprint
statement. - Example:
php <?php echo "Hello, PHP!"; // Output: Hello, PHP! ?>
- Variables and Data Types
- Declare variables using the
$
sign followed by the variable name. - PHP supports various data types: strings, integers, floats, booleans, arrays, etc.
- Example:
php <?php $name = "John"; $age = 30; $isStudent = true; ?>
- Comments
- As mentioned earlier, use
//
for single-line comments and/* */
for multi-line comments.
- Basic Operators
- PHP supports arithmetic, assignment, comparison, logical, and other operators.
- Refer to the “PHP Operators” section in this conversation for more details.
- Control Structures
- Use control structures like
if
,else
,elseif
,while
,for
,switch
, etc., to make decisions and control program flow. - Example:
<?php $score = 85; if ($score >= 90) { echo "A"; } elseif ($score >= 80) { echo "B"; } else { echo "C"; } ?>
- Arrays
- Arrays are used to store multiple values in a single variable.
- PHP supports indexed, associative, and multidimensional arrays.
- Example:
<?php $fruits = array("Apple", "Banana", "Orange"); echo $fruits[0]; // Output: Apple $person = array("name" => "John", "age" => 30, "isStudent" => true); echo $person["name"]; // Output: John ?>
- Functions
- Functions are blocks of code that can be reused throughout the program.
- They help modularize code and make it easier to manage.
- Example:
<?php function greet($name) { echo "Hello, $name!"; } greet("Alice"); // Output: Hello, Alice! greet("Bob"); // Output: Hello, Bob! ?>
This tutorial covers the basics of PHP, but there’s much more to learn to become proficient in web development with PHP. You can explore topics like handling forms, working with databases (MySQL, etc.), object-oriented programming, and popular frameworks like Laravel or CodeIgniter for more advanced projects. Happy coding!