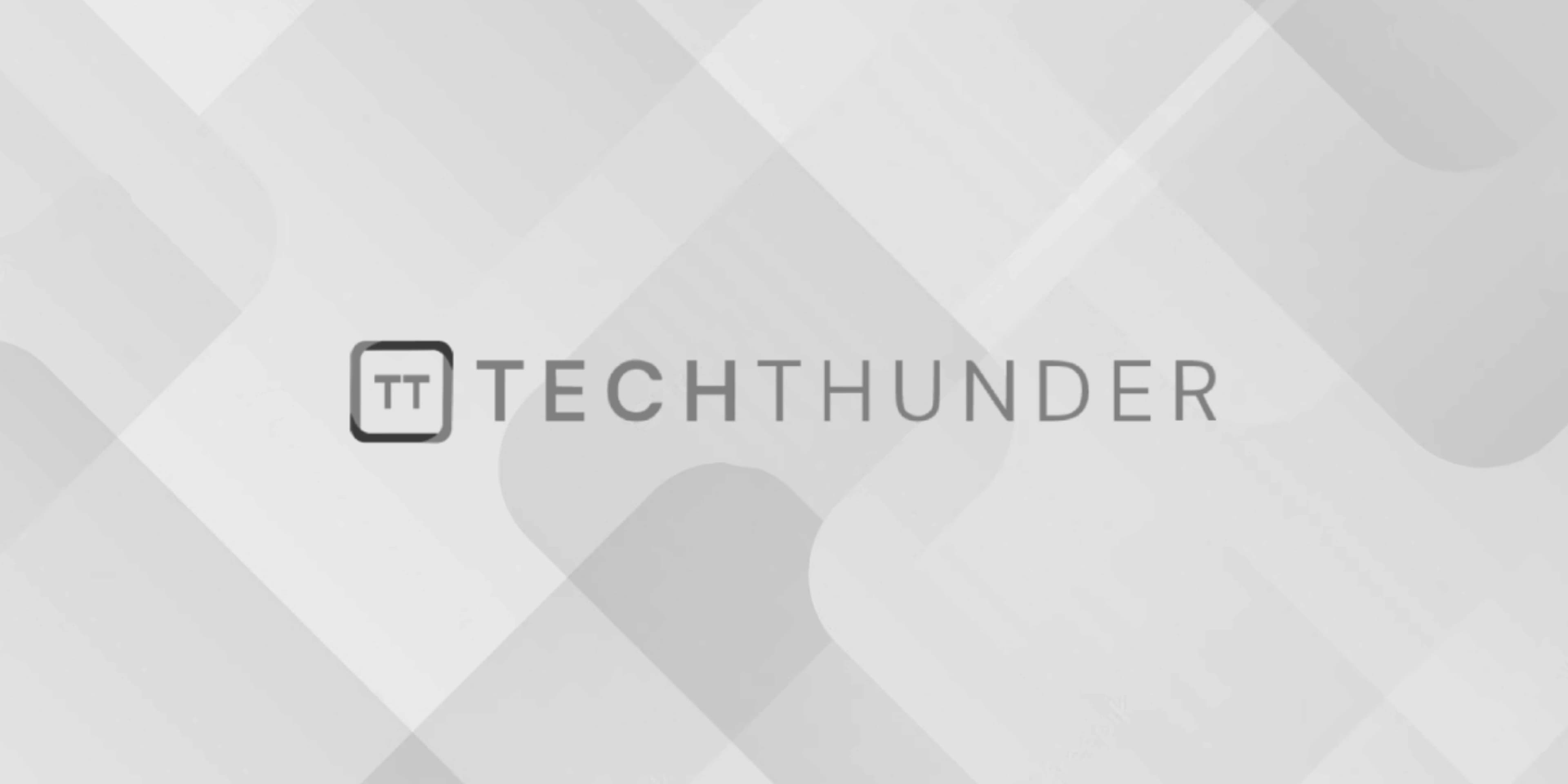
OOPs Type Hinting
In object-oriented programming (OOP), type hinting is a feature that allows you to specify the data type of a parameter in a method or function declaration. It helps enforce type safety by ensuring that the passed arguments match the expected data types. Type hinting is especially useful when working with objects and class instances, as it allows you to define the expected data types for method parameters or function arguments.
In PHP, type hinting is used with classes, interfaces, and some built-in data types. There are several types of type hinting available:
- Class Type Hinting:
You can specify that a parameter should be an instance of a particular class or one of its subclasses. This is done by using the class name as the type hint.
class Car {
// Method with class type hinting
public function startEngine(Car $car) {
// $car must be an instance of the Car class or its subclasses
// Method implementation...
}
}
- Interface Type Hinting:
You can also use type hinting with interfaces. This allows you to specify that a parameter should be an object that implements a specific interface.
interface LoggerInterface {
// Interface methods...
}
class FileLogger implements LoggerInterface {
// Implementation of LoggerInterface...
}
class DatabaseLogger implements LoggerInterface {
// Implementation of LoggerInterface...
}
class LogManager {
// Method with interface type hinting
public function logMessage(LoggerInterface $logger, $message) {
// $logger must be an object that implements LoggerInterface
// Method implementation...
}
}
- Array Type Hinting (PHP 5.1 and later):
You can specify that a parameter should be an array using thearray
type hint.
class ArrayProcessor {
// Method with array type hinting
public function processArray(array $data) {
// $data must be an array
// Method implementation...
}
}
- Scalar Type Hinting (PHP 7 and later):
With PHP 7, you can also use scalar type hinting for basic data types likestring
,int
,float
, andbool
.
class MathOperations {
// Method with scalar type hinting
public function addNumbers(int $num1, int $num2) {
// $num1 and $num2 must be integers
// Method implementation...
}
}
Using type hinting helps improve code readability, maintainability, and safety. It provides clear expectations for the types of data that methods or functions can accept, reducing the likelihood of errors and making code more self-documenting. If a mismatched type is passed as an argument, PHP will issue a warning or throw an error, depending on your PHP error reporting settings.