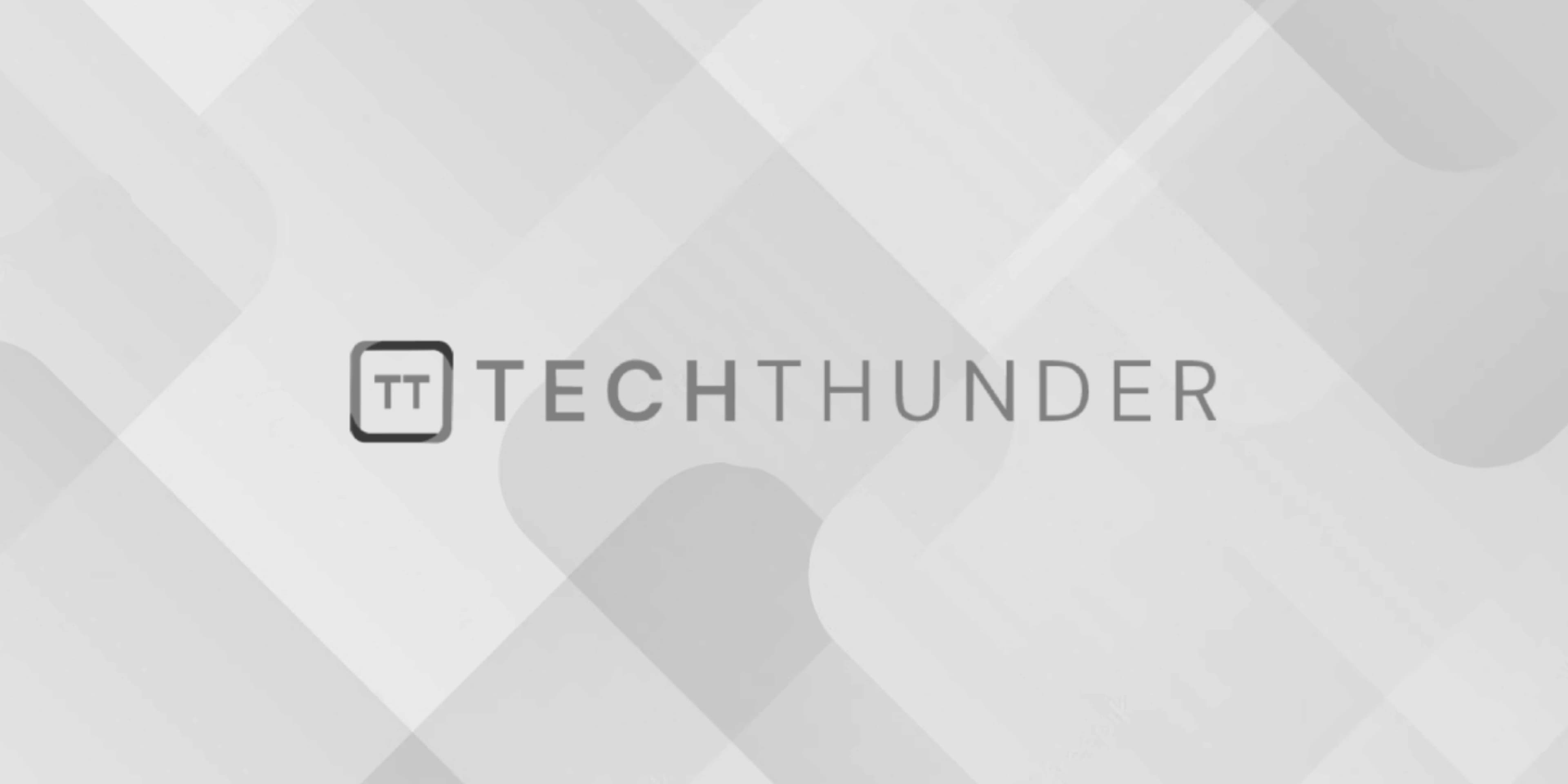
Multiple Inheritance in PHP
As of my last update in September 2021, PHP does not support multiple inheritance in the traditional sense, where a class can directly inherit from multiple parent classes. PHP uses single inheritance, which means a class can only inherit from one parent class. This is a design decision made by the PHP language to avoid ambiguity and complications that can arise from multiple inheritance.
However, PHP does provide a way to achieve similar functionality using interfaces and traits, which allow for code reuse and the sharing of behavior among multiple classes.
- Interfaces: An interface defines a contract that a class must adhere to by implementing its methods. A class can implement multiple interfaces, effectively allowing it to inherit behavior from multiple sources.
interface Animal {
public function makeSound();
}
interface Flyable {
public function fly();
}
class Bird implements Animal, Flyable {
public function makeSound() {
echo "Chirp, chirp!" . PHP_EOL;
}
public function fly() {
echo "Flying high!" . PHP_EOL;
}
}
In this example, the Bird
class implements both the Animal
and Flyable
interfaces, allowing it to inherit behavior from both interfaces.
- Traits: A trait is a group of methods that can be reused in multiple classes. A class can use multiple traits, effectively inheriting behavior from multiple sources.
trait CanRun {
public function run() {
echo "Running fast!" . PHP_EOL;
}
}
trait CanSwim {
public function swim() {
echo "Swimming gracefully!" . PHP_EOL;
}
}
class Dog {
use CanRun, CanSwim;
}
$dog = new Dog();
$dog->run(); // Output: Running fast!
$dog->swim(); // Output: Swimming gracefully!
In this example, the Dog
class uses both the CanRun
and CanSwim
traits, effectively inheriting behavior from both traits.
By using interfaces and traits, you can achieve a form of multiple inheritance in PHP while avoiding some of the complexities that can arise with traditional multiple inheritance. It allows for a more flexible and modular approach to code reuse and sharing behavior among classes.