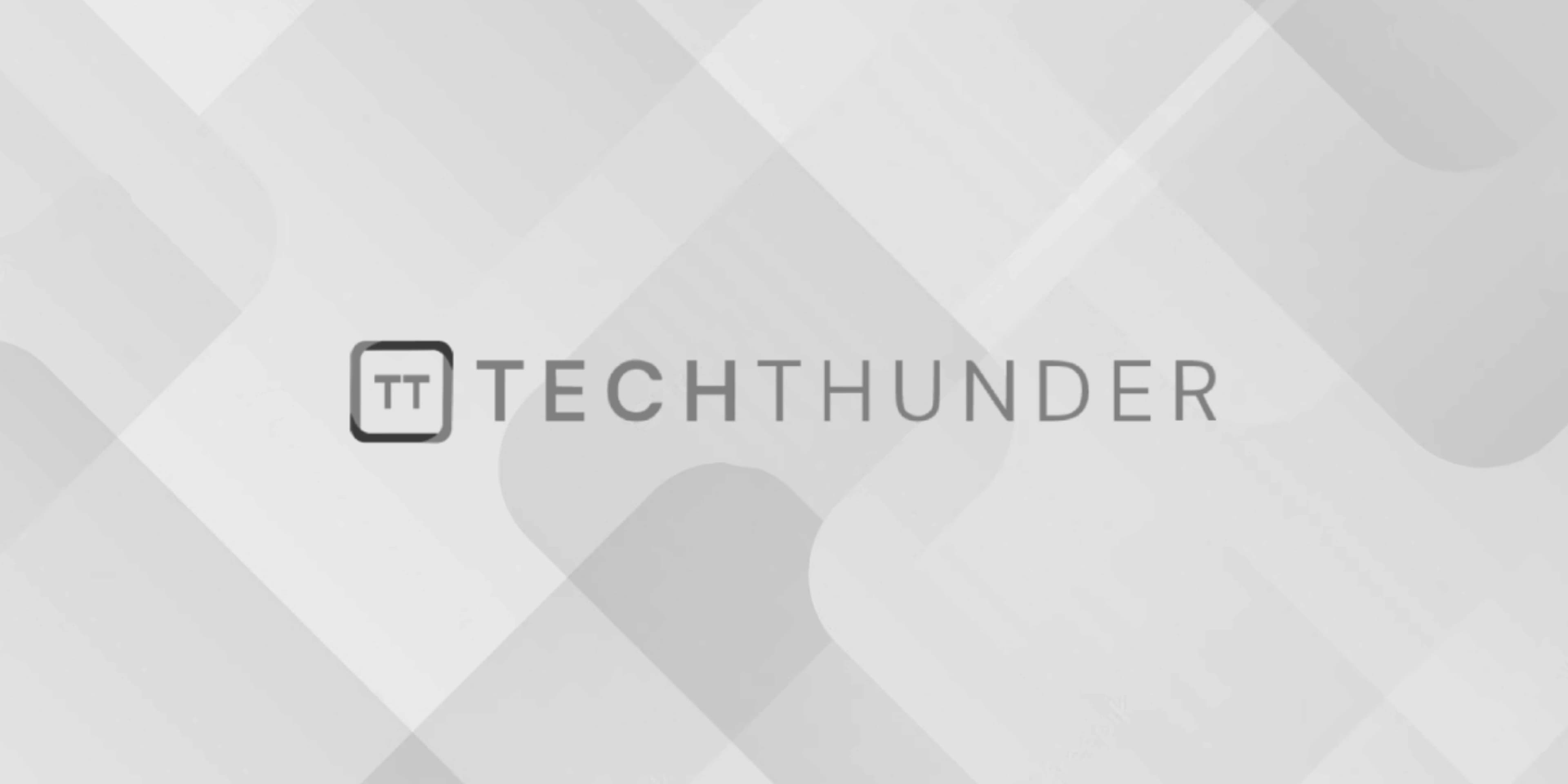
PHP Recursive Function
A recursive function is a function in PHP that calls itself during its execution. Recursive functions are used to solve problems that can be broken down into smaller, similar subproblems. Each recursive call typically operates on a smaller subset of the original problem until it reaches a base case, where the function no longer calls itself and the recursion stops.
Here’s an example of a simple recursive function to calculate the factorial of a number:
function factorial($n) {
if ($n == 0 || $n == 1) {
return 1; // Base case: factorial of 0 and 1 is 1
} else {
return $n * factorial($n - 1); // Recursive call
}
}
echo factorial(5); // Output: 120 (5! = 5 * 4 * 3 * 2 * 1 = 120)
In the example above, the factorial
function calculates the factorial of a given number $n
. If $n
is 0 or 1, it returns 1 (base case). Otherwise, it makes a recursive call to calculate the factorial of $n - 1
and multiplies it with $n
. This process continues until the base case is reached, and the function returns the final result.
Here’s another example of a recursive function to calculate the nth number in the Fibonacci sequence:
function fibonacci($n) {
if ($n == 0) {
return 0; // Base case: Fibonacci(0) = 0
} elseif ($n == 1) {
return 1; // Base case: Fibonacci(1) = 1
} else {
return fibonacci($n - 1) + fibonacci($n - 2); // Recursive call
}
}
echo fibonacci(7); // Output: 13 (7th number in the Fibonacci sequence)
In this example, the fibonacci
function calculates the nth number in the Fibonacci sequence. If $n
is 0 or 1, it returns the respective base case values. Otherwise, it makes two recursive calls to calculate the (n-1)th and (n-2)th Fibonacci numbers and adds them together to get the result.
Recursive functions can be very powerful for solving certain types of problems, but they should be used with caution. If not implemented carefully, recursive functions can lead to stack overflow errors due to excessive function calls. Always ensure that your recursive function has a proper base case and is designed to handle the termination condition correctly.