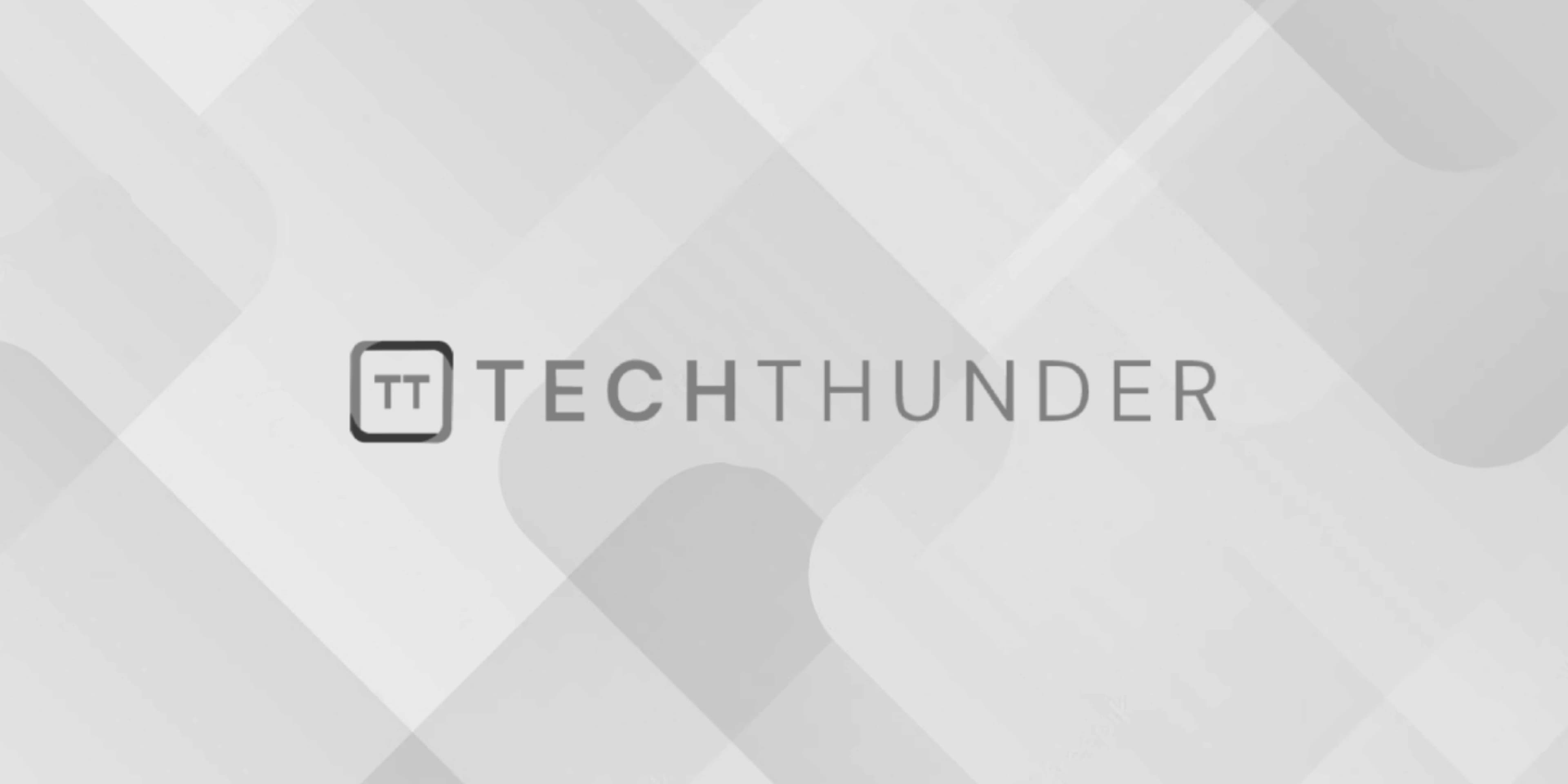
PHP ternary operator
In PHP, the ternary operator is a shorthand conditional expression that allows you to make concise decisions based on a condition. It is a compact way to write simple if-else statements. The ternary operator has the following syntax:
condition ? expression1 : expression2;
Here’s how it works:
- If the
condition
is true, the ternary operator returnsexpression1
. - If the
condition
is false, the ternary operator returnsexpression2
.
Example:
// Regular if-else statement
$age = 25;
if ($age >= 18) {
$status = "Adult";
} else {
$status = "Minor";
}
// Equivalent code using the ternary operator
$status = $age >= 18 ? "Adult" : "Minor";
In this example, we have an if-else statement that checks the value of the $age
variable. If the age is greater than or equal to 18, the $status
variable is set to “Adult”; otherwise, it is set to “Minor”. The same logic is achieved using the ternary operator in a more concise way.
The ternary operator is especially useful when you need to assign a value to a variable based on a simple condition. However, it’s important to use it judiciously and keep it simple. Avoid using complex expressions within the ternary operator, as it can reduce code readability and maintainability.
Nested ternary operators should also be used with caution, as they can quickly become difficult to read. In such cases, it’s often better to use regular if-else statements for clarity.
Remember that using the ternary operator is a matter of preference and coding style. Some developers prefer using it for short and simple conditionals, while others may choose to stick with traditional if-else statements for better readability and maintainability.