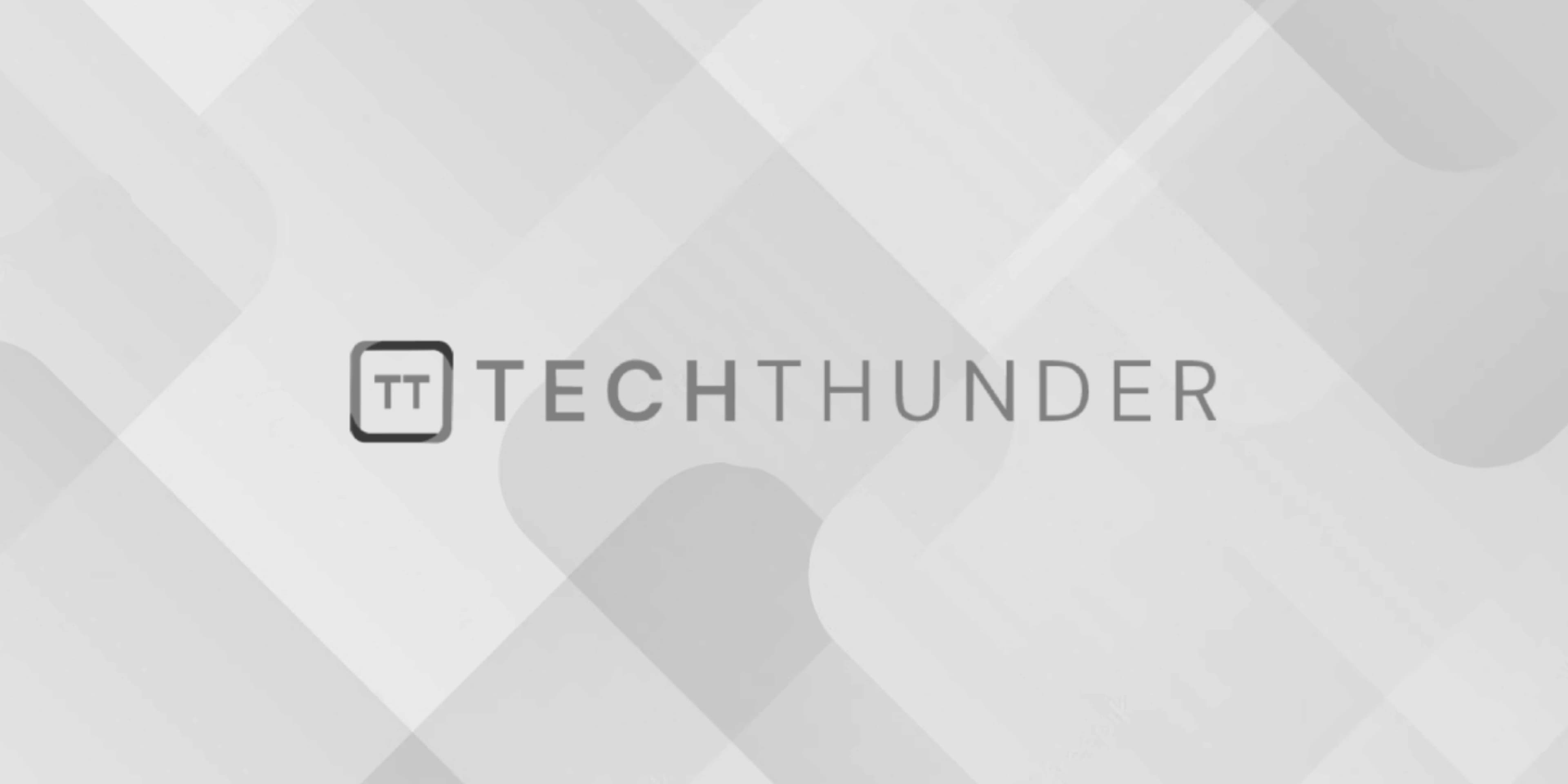
OOPs Const Keyword
In object-oriented programming (OOP), the const
keyword is used to define class constants. Class constants are similar to variables, but their values cannot be changed once they are defined. They are associated with the class rather than instances of the class, and they provide a way to define values that remain constant throughout the execution of the program.
In PHP, class constants are defined using the const
keyword followed by the constant name and its value. Class constants are typically written in uppercase letters to distinguish them from regular class properties.
Here’s the syntax to define a class constant:
class ClassName {
const CONSTANT_NAME = value;
}
Example:
class MathOperations {
const PI = 3.14159;
const EULER_NUMBER = 2.71828;
public function areaOfCircle($radius) {
return self::PI * $radius * $radius;
}
}
echo MathOperations::PI; // Output: 3.14159
echo MathOperations::EULER_NUMBER; // Output: 2.71828
$obj = new MathOperations();
echo $obj->areaOfCircle(5); // Output: 78.53975
In the example above, we defined two class constants PI
and EULER_NUMBER
in the MathOperations
class. These constants are accessible using the scope resolution operator ::
. Class constants are used without the dollar sign ($
) unlike class properties.
Some important points to note about class constants:
- Class constants can be accessed without creating an instance of the class, making them similar to static members.
- Class constants are shared among all instances of the class, and their values cannot be changed during the program’s execution.
- You can access class constants both from outside the class and from within the class methods using the
self::CONSTANT_NAME
syntax. - Class constants are often used for defining fixed values, such as mathematical constants, error codes, or configuration settings that should remain constant throughout the program.
By using class constants, you can define meaningful names for fixed values and make your code more readable and maintainable. They also help ensure that certain values remain consistent across the application, making it easier to manage and modify them when needed.