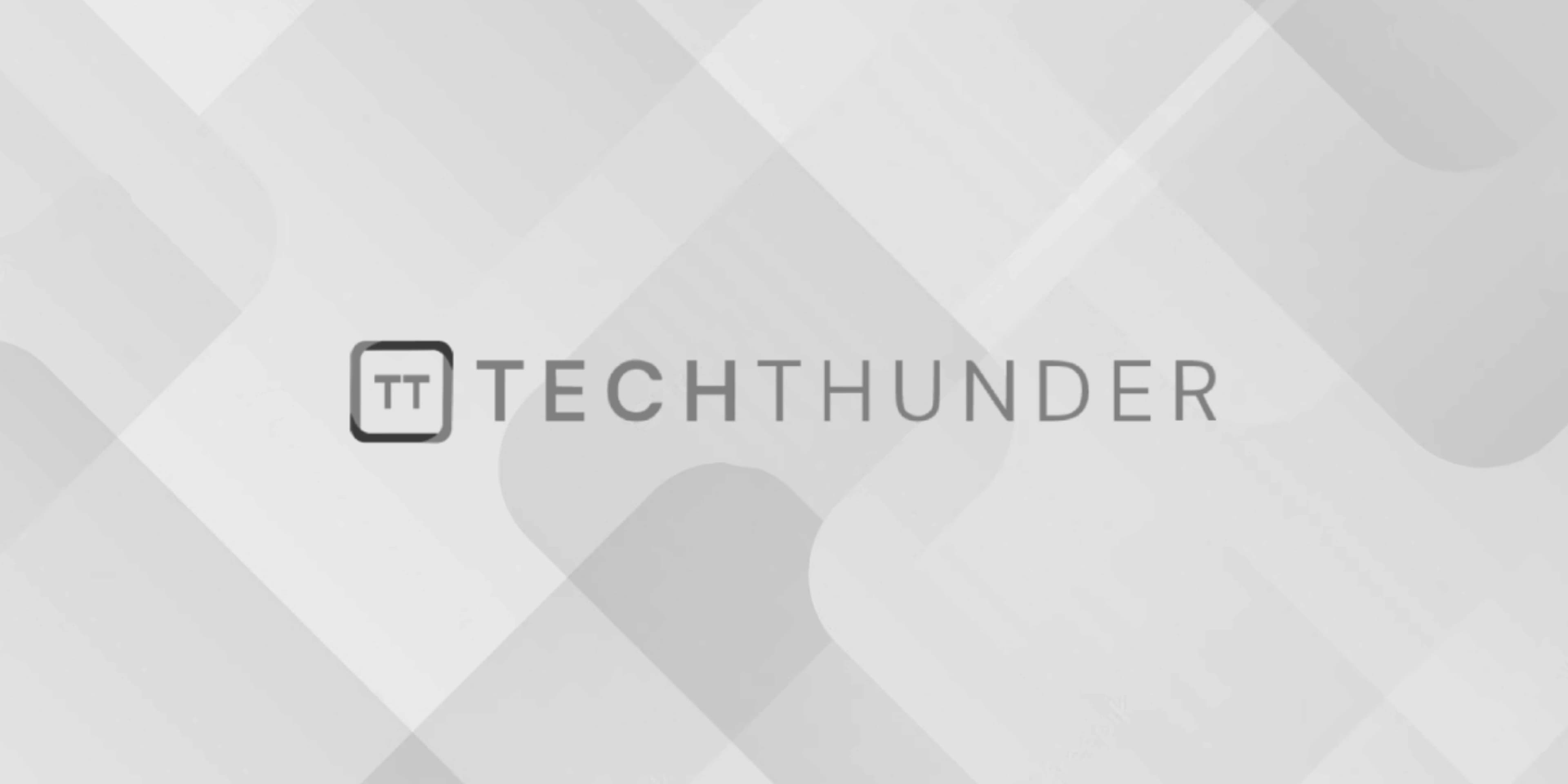
Get & Post Methods in PHP
In PHP, the GET
and POST
methods are two HTTP request methods used to send data from a client (usually a web browser) to a server. These methods are commonly used when submitting forms, passing parameters, or making HTTP requests to fetch data from a server.
- GET Method:
TheGET
method sends data to the server as part of the URL. When using theGET
method, the data is visible in the URL, which means the data is exposed and can be bookmarked or shared. This method is commonly used for fetching data and should not be used for sensitive or private information.
In PHP, you can access the GET
parameters using the $_GET
superglobal array. Here’s an example:
// Example URL: http://example.com/?name=John&age=30
$name = $_GET['name']; // $name will be 'John'
$age = $_GET['age']; // $age will be '30'
- POST Method:
ThePOST
method sends data to the server in the request body, rather than appending it to the URL. This method is more secure thanGET
since the data is not visible in the URL. It is commonly used for submitting forms and sending sensitive data.
In PHP, you can access the POST
parameters using the $_POST
superglobal array. Here’s an example:
<!-- Example HTML form using POST method -->
<form action="process.php" method="post">
<input type="text" name="username">
<input type="password" name="password">
<input type="submit" value="Submit">
</form>
// process.php
$username = $_POST['username'];
$password = $_POST['password'];
It’s important to note that both GET
and POST
methods have their use cases. Use GET
when you want to fetch data from the server, and use POST
when you want to submit data or perform actions that modify server data. Additionally, be cautious with sensitive information and avoid exposing sensitive data in the URL when using GET
. For sensitive information, always use the POST
method.