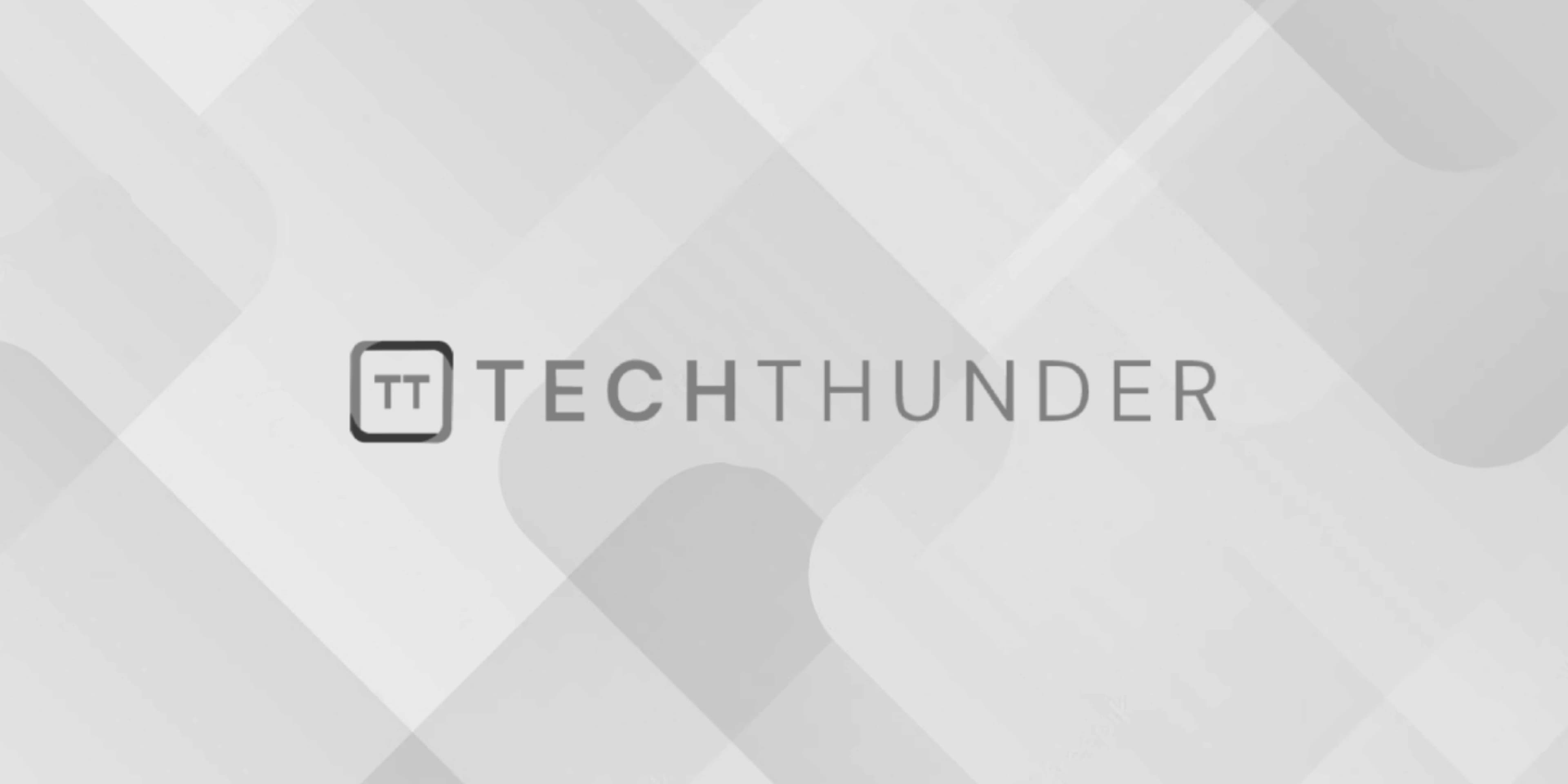
180 views
PHP Operators
In PHP, operators are symbols or special keywords that perform specific operations on one or more operands (variables, values, or expressions). PHP supports a wide range of operators, categorized into different types based on their functionality. Below are the main types of operators in PHP:
- Arithmetic Operators:
+
Addition-
Subtraction*
Multiplication/
Division%
Modulo (returns the remainder of a division)
- Assignment Operators:
=
Assigns the value on the right to the variable on the left+=
Adds the value on the right to the variable on the left and assigns the result-=
Subtracts the value on the right from the variable on the left and assigns the result*=
Multiplies the variable on the left by the value on the right and assigns the result/=
Divides the variable on the left by the value on the right and assigns the result%=
Computes the modulo of the variable on the left by the value on the right and assigns the result
- Comparison Operators:
==
Equality!=
or<>
Inequality===
Identity (equal value and equal type)!==
Non-identity (not equal value or not equal type)>
Greater than<
Less than>=
Greater than or equal to<=
Less than or equal to
- Logical Operators:
&&
orand
Logical AND||
oror
Logical OR!
ornot
Logical NOT
- Increment/Decrement Operators:
++
Increment by 1--
Decrement by 1
- String Operators:
.
Concatenation (joins two strings together)
- Array Operators:
+
Union (combines arrays, elements from both arrays, duplicates removed)==
Equality (checks if both arrays have the same key/value pairs)===
Identity (checks if both arrays are identical)
- Ternary Operator:
expr1 ? expr2 : expr3
Ifexpr1
is true, evaluates toexpr2
; otherwise, evaluates toexpr3
.
- Null Coalescing Operator (introduced in PHP 7):
??
Returns the value of the left operand if it is not null; otherwise, returns the right operand.
These are the main operators in PHP. Understanding how to use them effectively is essential for writing efficient and concise PHP code.