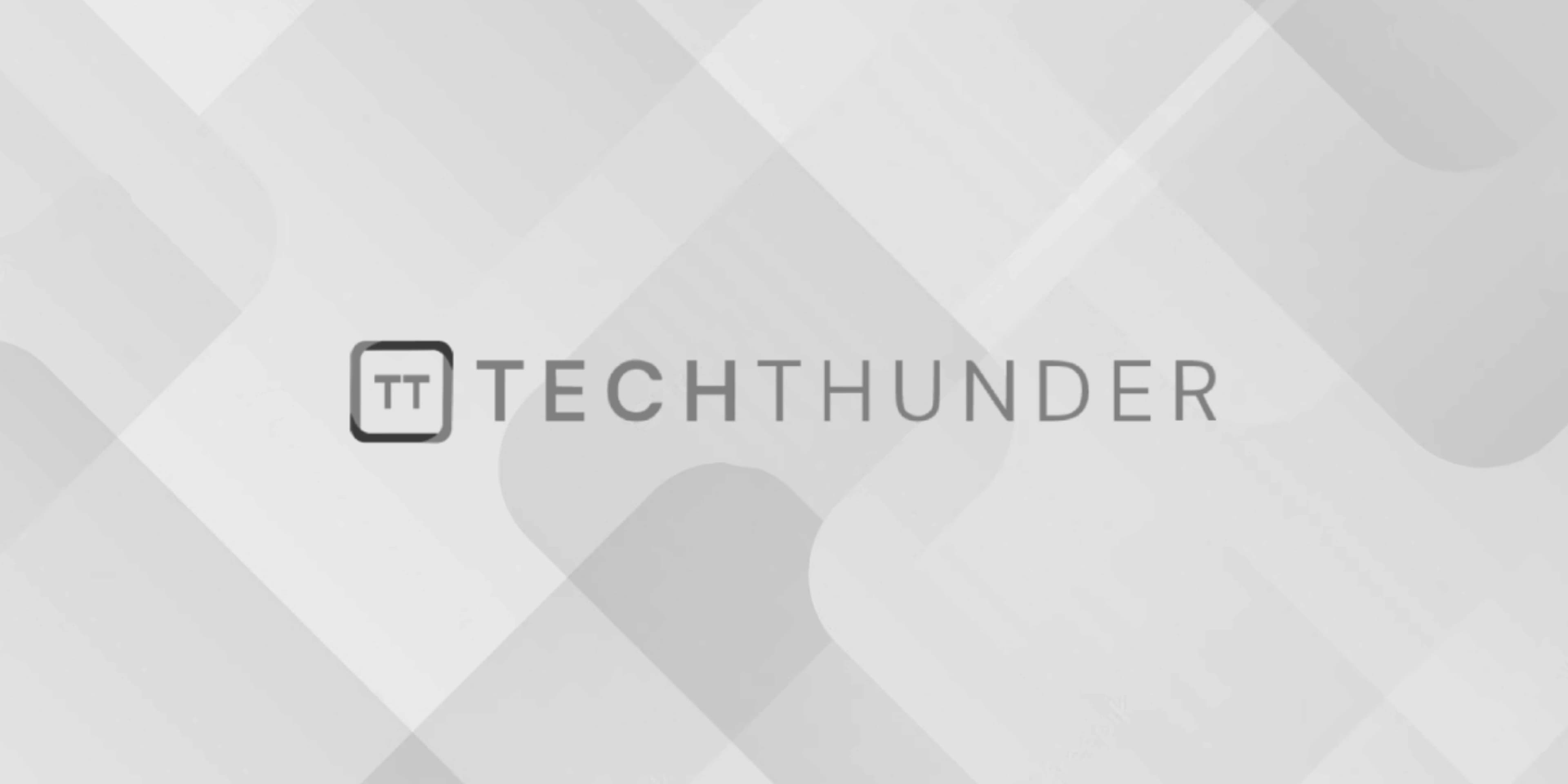
229 views
PHP Contact Form
Creating a PHP contact form involves setting up an HTML form to collect user information and using PHP to process the form data and send an email. Below is a basic example of how to create a PHP contact form:
- HTML Form (index.html):
Create an HTML form with input fields for the user’s name, email address, subject, and message.
<!DOCTYPE html>
<html>
<head>
<title>Contact Form</title>
</head>
<body>
<h2>Contact Us</h2>
<form action="contact.php" method="post">
<label for="name">Name:</label>
<input type="text" name="name" required><br>
<label for="email">Email:</label>
<input type="email" name="email" required><br>
<label for="subject">Subject:</label>
<input type="text" name="subject" required><br>
<label for="message">Message:</label><br>
<textarea name="message" rows="5" cols="40" required></textarea><br>
<input type="submit" name="submit" value="Submit">
</form>
</body>
</html>
- PHP Processing Script (contact.php):
Create a PHP script (contact.php) to process the form data and send an email to the desired recipient.
<?php
if ($_SERVER["REQUEST_METHOD"] === "POST") {
// Get form data
$name = $_POST["name"];
$email = $_POST["email"];
$subject = $_POST["subject"];
$message = $_POST["message"];
// Set recipient email (replace with your email address)
$recipient = "[email protected]";
// Set headers
$headers = "From: $name <$email>\r\n";
$headers .= "Reply-To: $email\r\n";
$headers .= "Content-Type: text/html; charset=UTF-8\r\n";
// Send the email
$mailSent = mail($recipient, $subject, $message, $headers);
if ($mailSent) {
echo "Thank you for contacting us. We will get back to you shortly.";
} else {
echo "Oops! Something went wrong. Please try again later.";
}
}
?>
In this example, the contact.php
script checks if the form is submitted (via $_SERVER["REQUEST_METHOD"] === "POST"
) and then retrieves the form data using the $_POST
superglobal array. It then sets up the email headers and sends the email using the mail()
function.
Note:
- Make sure to replace “[email protected]” with the actual recipient email address where you want the contact form submissions to be sent.
- The
mail()
function may not work correctly on all servers, and emails from some servers may be flagged as spam. For production use, consider using a reliable email library or an email service provider.
Remember to properly sanitize and validate user input before processing it to prevent security vulnerabilities. Additionally, implement CAPTCHA or other anti-spam measures to prevent abuse of the contact form.