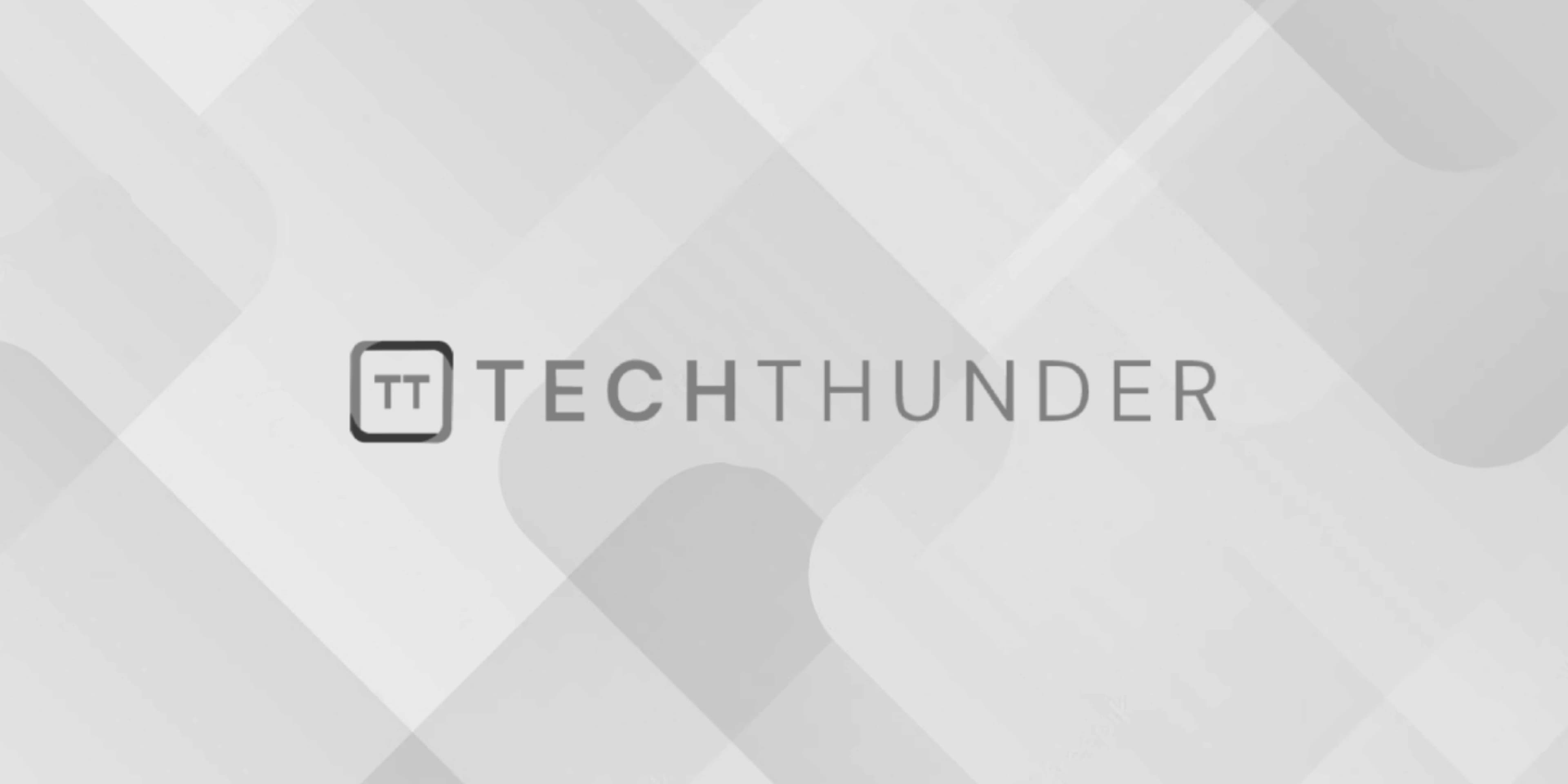
PHP Magic Constants
In PHP, magic constants are special predefined constants that change their value depending on where they are used in the script. These constants start with a double underscore (__
) followed by the constant name. They are called “magic” because their values are automatically determined by PHP without the need for explicit definition.
PHP provides several magic constants that can be used in different contexts:
__LINE__
: Represents the current line number in the script where the constant is used.__FILE__
: Represents the full path and filename of the script where the constant is used.__DIR__
: Represents the directory of the file where the constant is used.__FUNCTION__
: Represents the name of the current function where the constant is used.__CLASS__
: Represents the name of the current class where the constant is used.__TRAIT__
: Represents the name of the current trait where the constant is used.__METHOD__
: Represents the name of the current method (function) where the constant is used.__NAMESPACE__
: Represents the name of the current namespace where the constant is used.
These magic constants are automatically updated by PHP depending on their context. For example:
// Example usage of magic constants
echo "Line number: " . __LINE__ . "<br>";
echo "Current file: " . __FILE__ . "<br>";
echo "Current directory: " . __DIR__ . "<br>";
function myFunction() {
echo "Function name: " . __FUNCTION__ . "<br>";
echo "Class name: " . __CLASS__ . "<br>";
echo "Method name: " . __METHOD__ . "<br>";
}
class MyClass {
use MyTrait;
public function myMethod() {
echo "Function name inside class: " . __FUNCTION__ . "<br>";
echo "Class name inside class: " . __CLASS__ . "<br>";
echo "Method name inside class: " . __METHOD__ . "<br>";
}
}
myFunction();
$object = new MyClass();
$object->myMethod();
Output:
Line number: 2
Current file: /path/to/your/file.php
Current directory: /path/to/your
Function name: myFunction
Class name: MyClass
Method name: MyClass::myMethod
Function name inside class: myMethod
Class name inside class: MyClass
Method name inside class: MyClass::myMethod
As you can see in the example, the magic constants dynamically provide information about the current script’s context, making it easier to handle and debug code. They are particularly useful for logging, error handling, and generating dynamic information about the script’s execution.