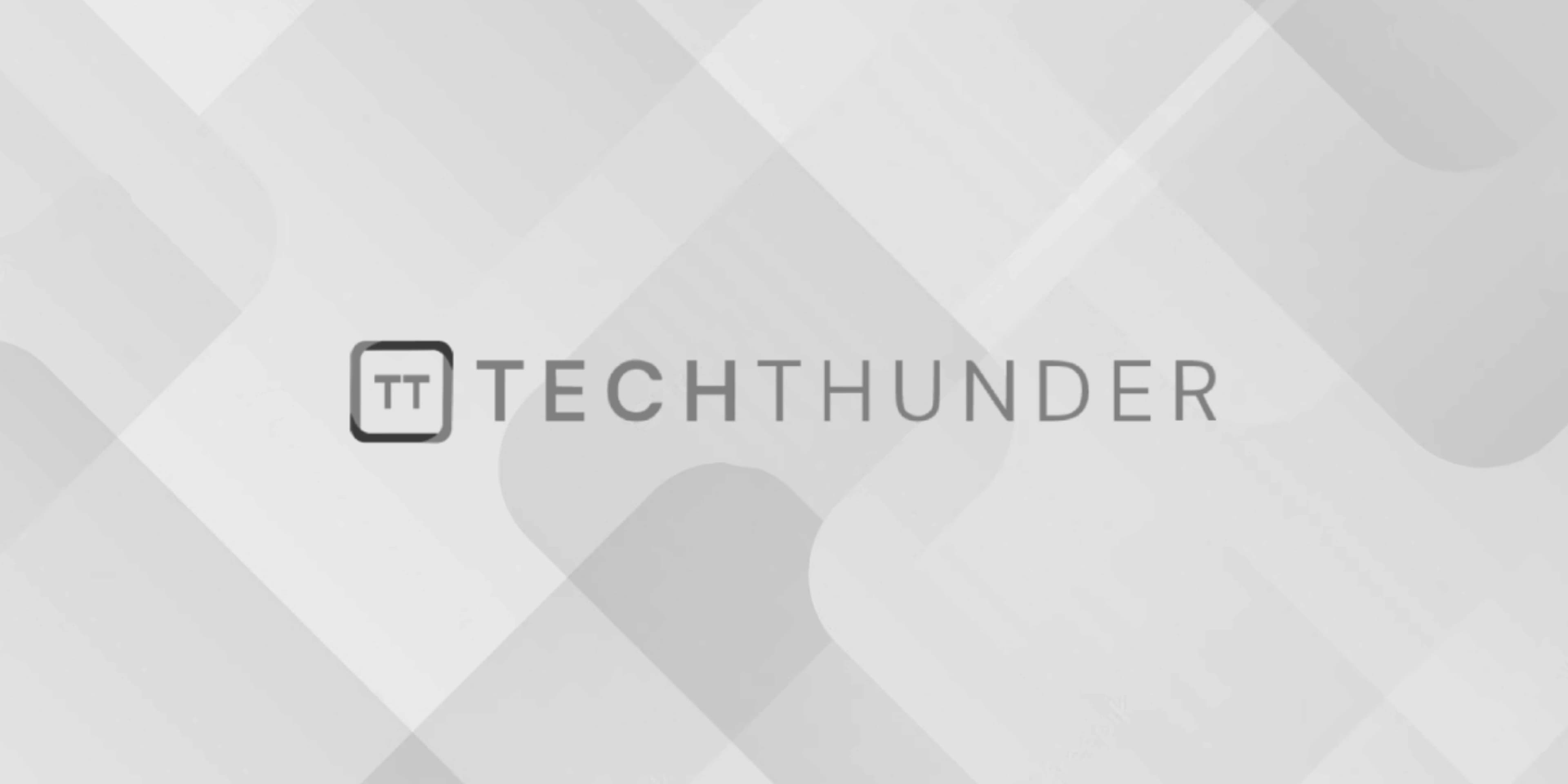
250 views
OOPs Concepts
Object-Oriented Programming (OOP) is a programming paradigm that organizes and models software based on the concept of “objects.” It focuses on breaking down complex problems into smaller, more manageable entities that can interact with each other to perform tasks and solve problems. OOP revolves around four main concepts:
- Encapsulation: Encapsulation is the concept of bundling data (attributes) and methods (functions) that operate on that data within a single unit called an “object.” An object acts as a self-contained entity, and its internal data is hidden from the outside world. The object exposes specific methods that allow controlled access to its internal state. This principle ensures data integrity and protects the internal implementation details of the object.
- Abstraction: Abstraction is the process of simplifying complex systems by presenting only the essential features and hiding unnecessary details. In OOP, classes and objects provide abstraction, allowing you to create models of real-world entities with relevant properties and behaviors. Abstraction allows you to focus on what an object does rather than how it does it, making the code easier to understand and maintain.
- Inheritance: Inheritance is the concept of creating new classes (derived classes or subclasses) based on existing classes (base classes or superclasses). The derived class inherits the properties and behaviors (attributes and methods) of the base class, and it can also extend or override these inherited features. Inheritance promotes code reuse and helps build a hierarchical relationship between classes.
- Polymorphism: Polymorphism allows objects of different classes to be treated as objects of a common superclass. It enables you to use a single interface to represent different types of objects, providing flexibility and extensibility in your code. Polymorphism is achieved through method overloading and method overriding.
Other related concepts in OOP include:
- Class: A class is a blueprint or template for creating objects. It defines the structure and behavior of objects of that type.
- Object: An object is an instance of a class. It represents a real-world entity and encapsulates data and behavior defined by the class.
- Method: A method is a function defined within a class that defines the behavior of objects of that class.
- Constructor: A constructor is a special method used to initialize objects when they are created from a class. It sets initial values for the object’s attributes.
- Encapsulation, inheritance, and polymorphism together are often referred to as the “three pillars” of OOP.
OOP allows developers to create modular, reusable, and maintainable code by organizing it into objects and classes. It has become a widely used paradigm for software development, providing a powerful and intuitive way to design and build complex systems.