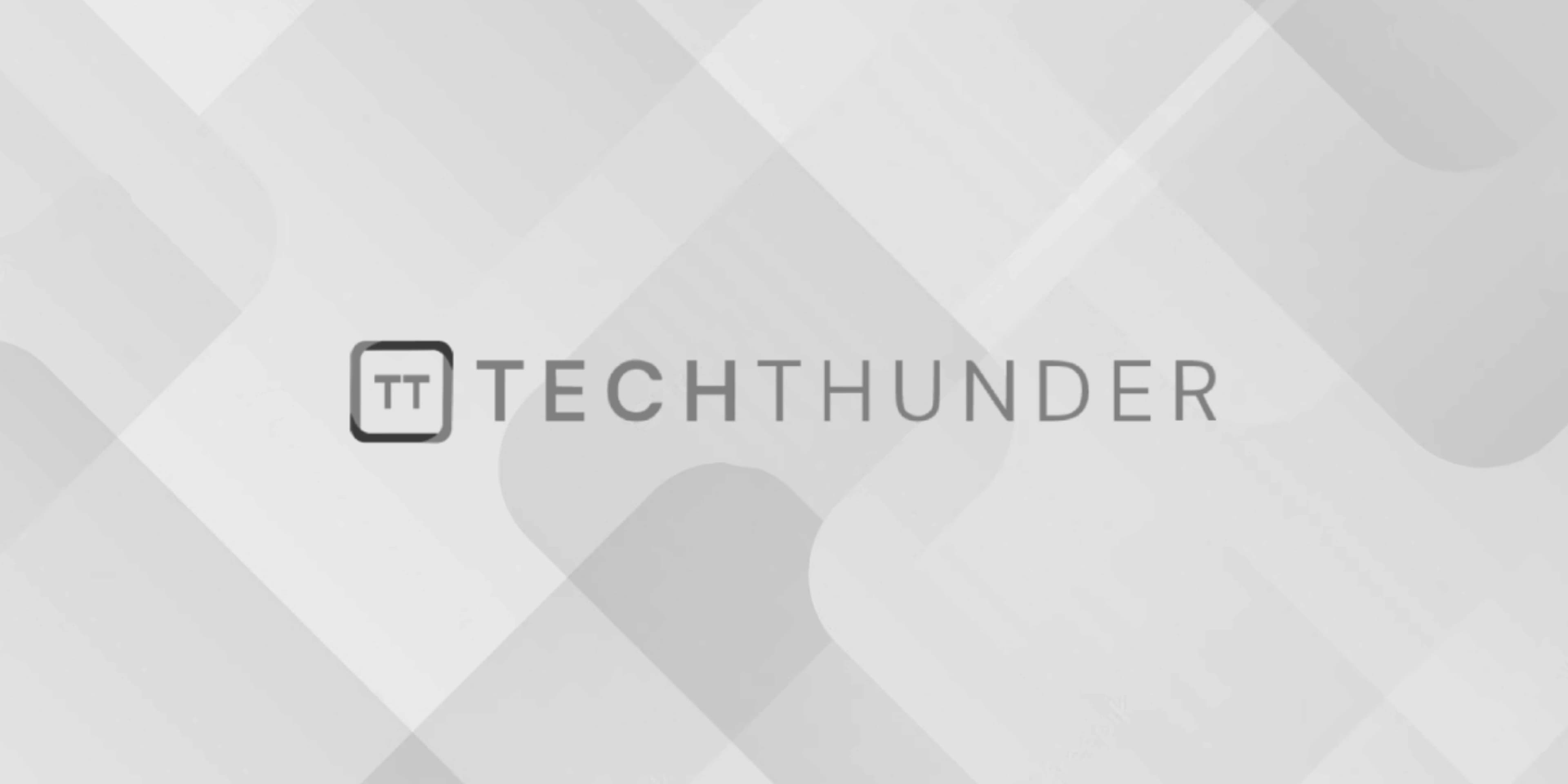
PHP While Loop
In PHP, the while
loop is a control structure that allows you to execute a block of code repeatedly as long as a specified condition is true. It is useful when you don’t know the exact number of iterations in advance but want to continue the loop as long as the condition remains true.
The basic syntax of the while
loop is as follows:
while (condition) {
// Code to be executed in each iteration
}
Here’s how the while
loop works:
- Condition: The loop will continue as long as the condition is true. The condition is evaluated before each iteration, and if it evaluates to true, the code block inside the loop is executed.
- Code Execution: The code block inside the loop is executed in each iteration as long as the condition is true.
- Termination: The loop will terminate when the condition evaluates to false. If the condition is false from the beginning, the code block inside the loop will never be executed.
Example of a while
loop:
$i = 1;
while ($i <= 5) {
echo $i . " ";
$i++;
}
Output:
1 2 3 4 5
In this example, the while
loop starts with the variable $i
initialized to 1. The loop will continue as long as $i
is less than or equal to 5. In each iteration, the value of $i
is echoed, and then $i
is incremented by 1 ($i++
) after each iteration.
The while
loop is useful when you want to repeatedly execute a block of code based on a condition. It is commonly used when the number of iterations is determined dynamically or when you want to continue the loop until a specific condition is no longer true. However, you need to be cautious with the loop’s condition to avoid infinite loops if the condition never becomes false.