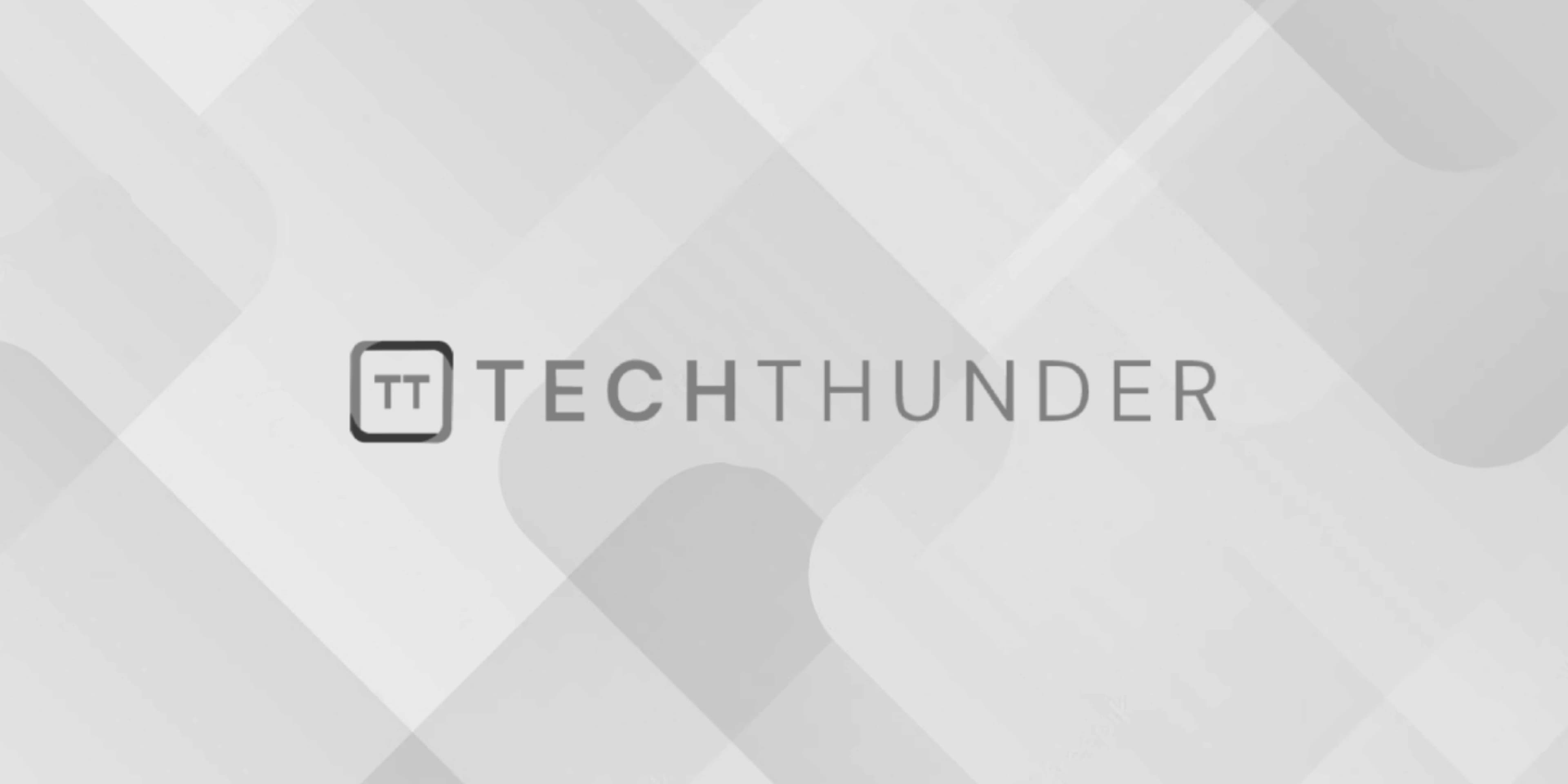
174 views
PHP append to array
In PHP, you can append elements to an array using various methods. Arrays are one of the fundamental data structures in PHP, and they allow you to store multiple values of different types in a single variable. Here are some common ways to append elements to an array:
- Using square brackets with empty index (Recommended):
$myArray = array(); // or $myArray = [];
$myArray[] = 'Apple';
$myArray[] = 'Banana';
$myArray[] = 'Orange';
- Using the
array_push()
function:
$myArray = array();
array_push($myArray, 'Apple', 'Banana', 'Orange');
- Assigning a value to a specific index in the array:
$myArray = array();
$myArray[0] = 'Apple';
$myArray[1] = 'Banana';
$myArray[2] = 'Orange';
- Using the
+=
operator to merge arrays:
$myArray = array('Apple', 'Banana');
$newElements = array('Orange', 'Mango');
$myArray += $newElements;
In the last example, the +=
operator merges the $newElements
array into the $myArray
, appending the elements from $newElements
to the end of $myArray
.
After appending elements to the array, you can access them using their index as follows:
echo $myArray[0]; // Output: Apple
echo $myArray[1]; // Output: Banana
echo $myArray[2]; // Output: Orange
Remember that arrays in PHP are zero-indexed, meaning the first element has an index of 0, the second element has an index of 1, and so on.