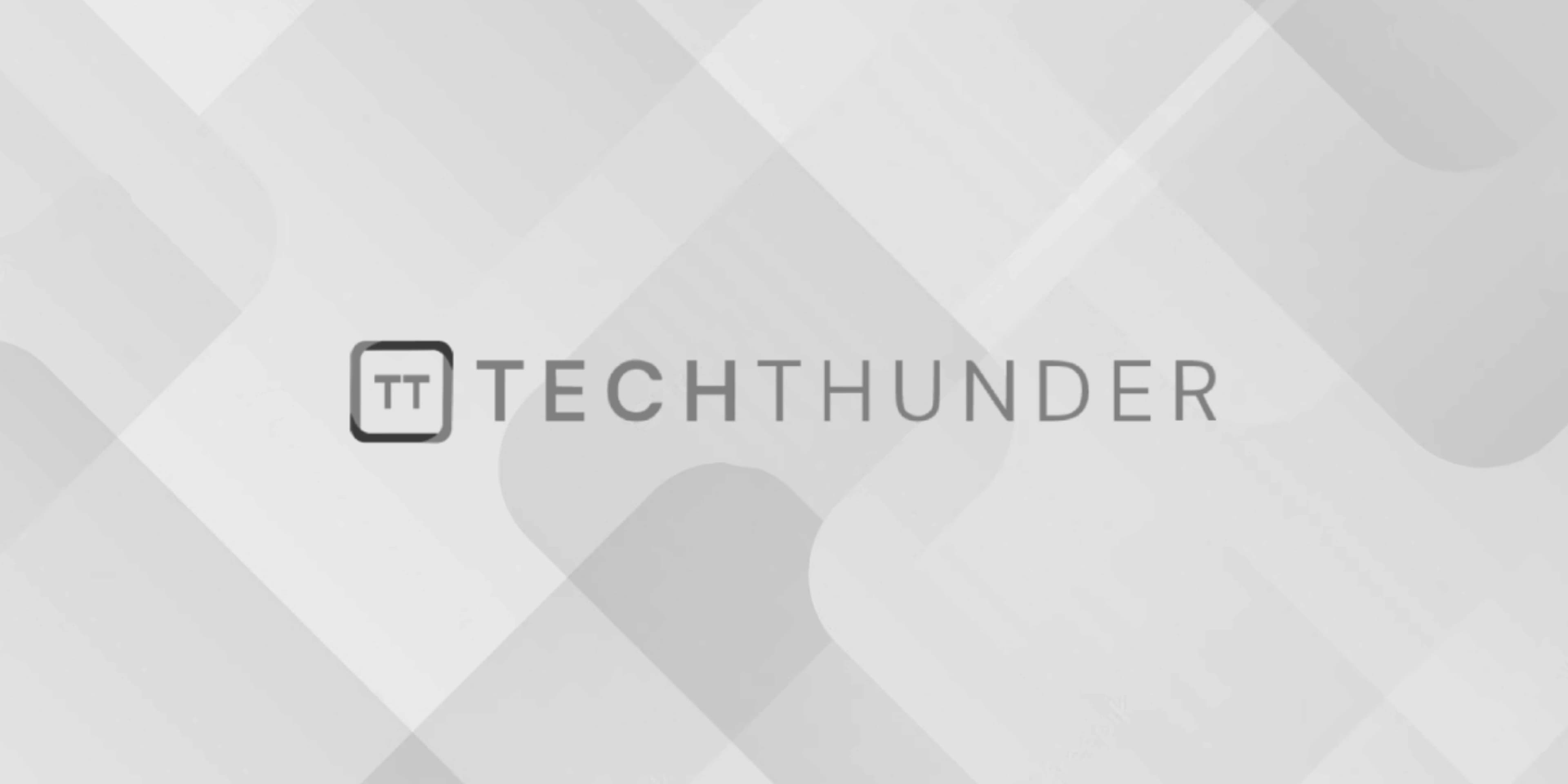
PHP file_get_contents() Function
The file_get_contents()
function in PHP is used to read the contents of a file into a string. It provides a simple and convenient way to access the contents of a file as a string, which can be useful for various tasks such as reading configuration files, reading data from text files, or fetching data from URLs.
Here’s the syntax of the file_get_contents()
function:
string|false file_get_contents ( string $filename [, bool $use_include_path = false [, resource $context [, int $offset = 0 [, int $maxlen ]]]] )
Parameters:
$filename
: The path to the file you want to read. This can be a local file path or a URL.$use_include_path
(optional): If set totrue
, PHP will also search for the file in the include path.$context
(optional): A stream context resource created withstream_context_create()
that can be used to set additional options for the file reading operation.$offset
(optional): The starting offset within the file where reading should begin.$maxlen
(optional): The maximum number of bytes to read from the file. If not specified, the whole file will be read.
Return value:
- On success, the function returns the contents of the file as a string.
- On failure, it returns
false
.
Here’s a simple example of using file_get_contents()
to read a local file:
$filename = 'example.txt';
$content = file_get_contents($filename);
if ($content !== false) {
echo $content;
} else {
echo "Error reading the file.";
}
In this example, the contents of the file “example.txt” will be read into the $content
variable, and then it will be echoed on the screen. If there is an error reading the file, the function will return false
, and an error message will be displayed.
You can also use file_get_contents()
to read data from URLs. For example:
$url = 'https://www.example.com/data.txt';
$content = file_get_contents($url);
if ($content !== false) {
echo $content;
} else {
echo "Error fetching data from URL.";
}
In this case, the function will fetch the contents of the URL “https://www.example.com/data.txt” and store it in the $content
variable. If there is an error fetching the data, it will return false
, and an error message will be displayed.
Remember that when using file_get_contents()
to read data from URLs, make sure that the allow_url_fopen
directive is enabled in your PHP configuration to allow fetching data from remote URLs. Also, keep in mind that the function returns the entire content of the file as a string, so it may not be suitable for large files or cases where you need to process the data in smaller chunks. In such cases, you may consider using other file handling functions or stream processing methods.