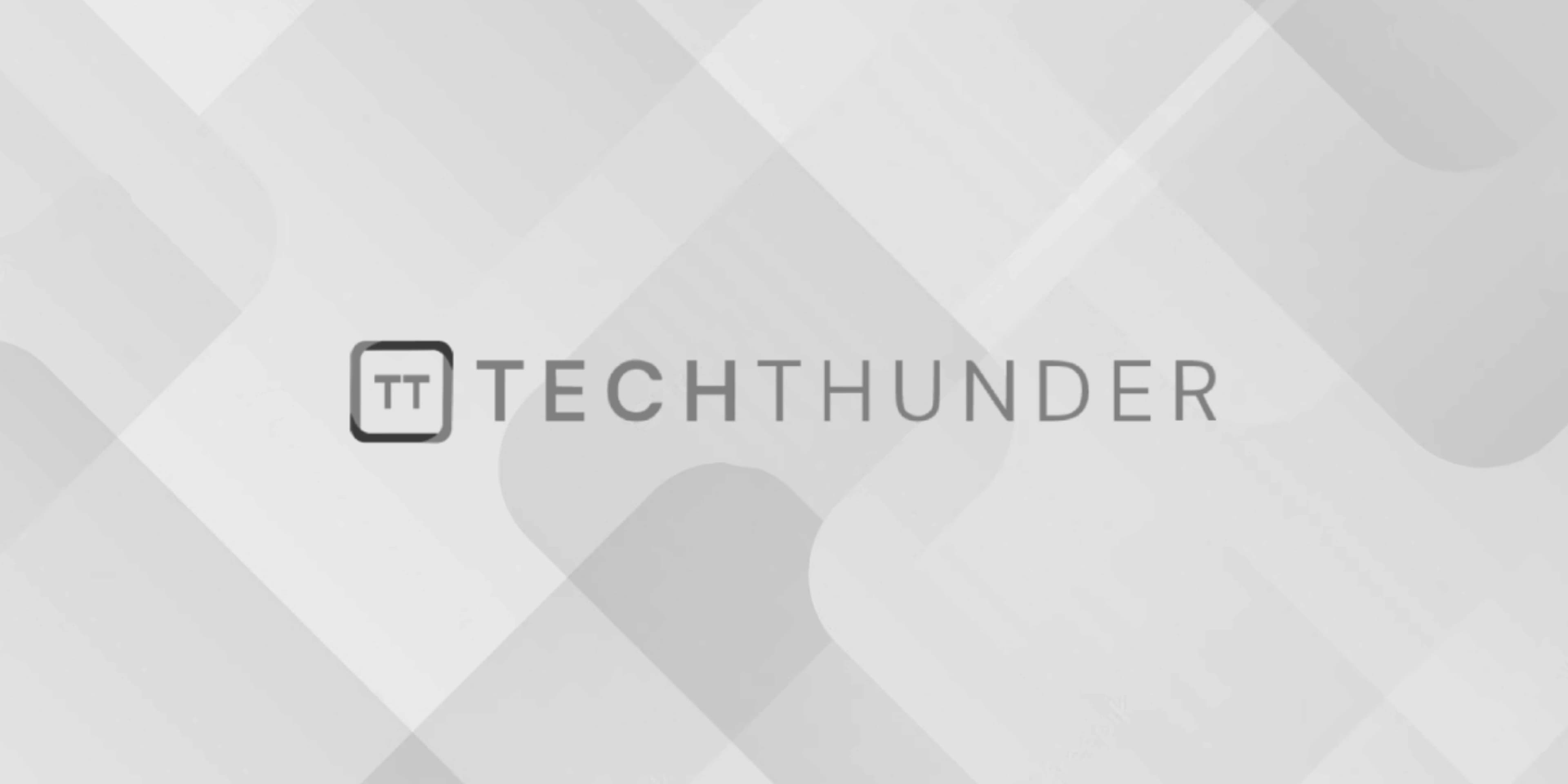
PHP include & require
In PHP, both include
and require
are used to include and execute external PHP files within another PHP file. They allow you to split your code into reusable components, making your application more modular and maintainable.
The main difference between include
and require
lies in how they handle errors if the specified file is not found or if there are other issues during inclusion:
include
:
- If the specified file is not found or if there is an error during inclusion, PHP will produce a warning, but the script will continue execution. This means that if the file is missing or contains errors, the script will still run, and other parts of the code may work as expected.
Example:
// If file.php is missing or contains errors, a warning will be shown, but the script continues.
include 'file.php';
require
:
- If the specified file is not found or if there is an error during inclusion, PHP will produce a fatal error, and the script execution will stop immediately. This means that if the file is missing or contains errors, the entire script will terminate, and nothing beyond the
require
statement will be executed.
Example:
// If file.php is missing or contains errors, a fatal error will occur, and script execution stops.
require 'file.php';
It’s essential to choose between include
and require
based on the criticality of the file you want to include. If the file is essential for the proper functioning of your application, and you want to ensure that the application cannot proceed without it, then require
is a better choice. On the other hand, if the file is optional, or you want to continue executing the script even if the file is missing or contains errors, you can use include
.
Both include
and require
can include files with either PHP code or HTML content. Additionally, you can use include_once
and require_once
to ensure that a file is included only once, even if the inclusion statement appears multiple times in the script. This can prevent issues related to redefining functions or classes.
Example of include_once
:
include_once 'file.php'; // Ensures that file.php is included only once.
Example of require_once
:
require_once 'file.php'; // Ensures that file.php is included only once.
It’s worth noting that the use of require
or require_once
is more common when including essential files, as it enforces strict inclusion, and any issues are detected and addressed during development or testing. For non-essential files or cases where you want to handle potential errors gracefully, you may choose to use include
or include_once
.