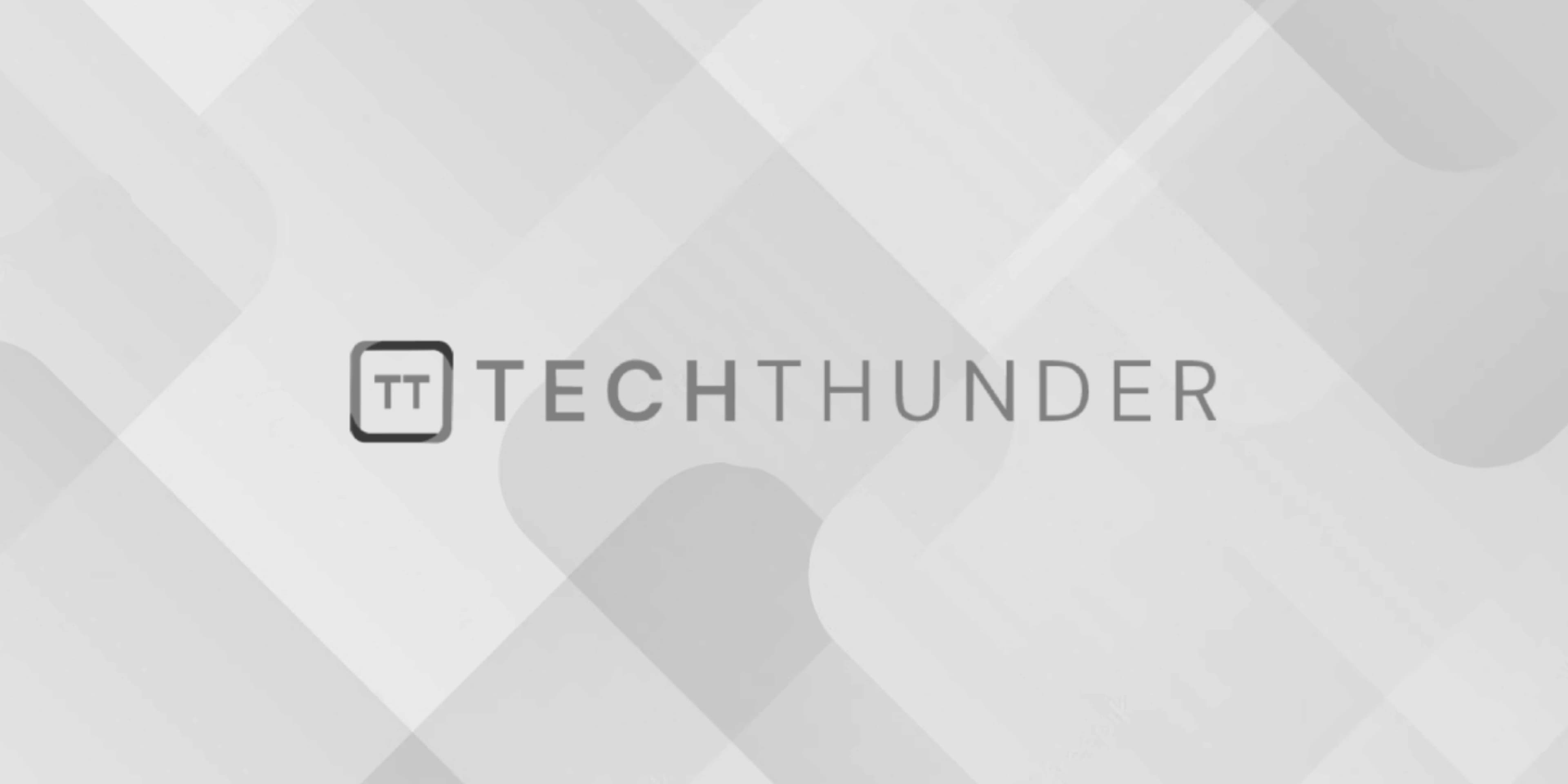
191 views
PHP string Concatenation
In PHP, string concatenation is the process of combining two or more strings into a single string. There are several ways to perform string concatenation in PHP:
- Using the
.
(dot) Operator:
The most common method for concatenation in PHP is using the dot operator, which is used to concatenate strings together.
$firstName = 'John';
$lastName = 'Doe';
// Concatenation using the dot operator
$fullName = $firstName . ' ' . $lastName;
echo $fullName; // Output: "John Doe"
- Using the
.=
Assignment Operator:
The.=
operator is a shorthand way of concatenating a string to an existing string variable.
$greeting = 'Hello, ';
// Concatenation using the .= operator
$greeting .= 'World!';
echo $greeting; // Output: "Hello, World!"
- Using
sprintf()
Function:
Thesprintf()
function allows you to format a string and replace placeholders with variables.
$firstName = 'John';
$lastName = 'Doe';
// Concatenation using sprintf()
$fullName = sprintf('%s %s', $firstName, $lastName);
echo $fullName; // Output: "John Doe"
- Using Double Quotes (Interpolation):
When using double quotes to define a string, you can directly include variables inside the string, and PHP will automatically interpolate the variable values.
$firstName = 'John';
$lastName = 'Doe';
// Concatenation using double quotes (interpolation)
$fullName = "$firstName $lastName";
echo $fullName; // Output: "John Doe"
- Using Heredoc Syntax:
Heredoc syntax is another way to create a multi-line string and include variables within it.
$firstName = 'John';
$lastName = 'Doe';
// Concatenation using heredoc syntax
$fullName = <<<EOD
{$firstName} {$lastName}
EOD;
echo $fullName; // Output: "John Doe"
All these methods achieve the same result of combining strings, but the choice of which one to use depends on the specific requirements and coding style preferences. Using the dot operator and interpolation with double quotes are the most commonly used and straightforward ways for string concatenation in PHP.