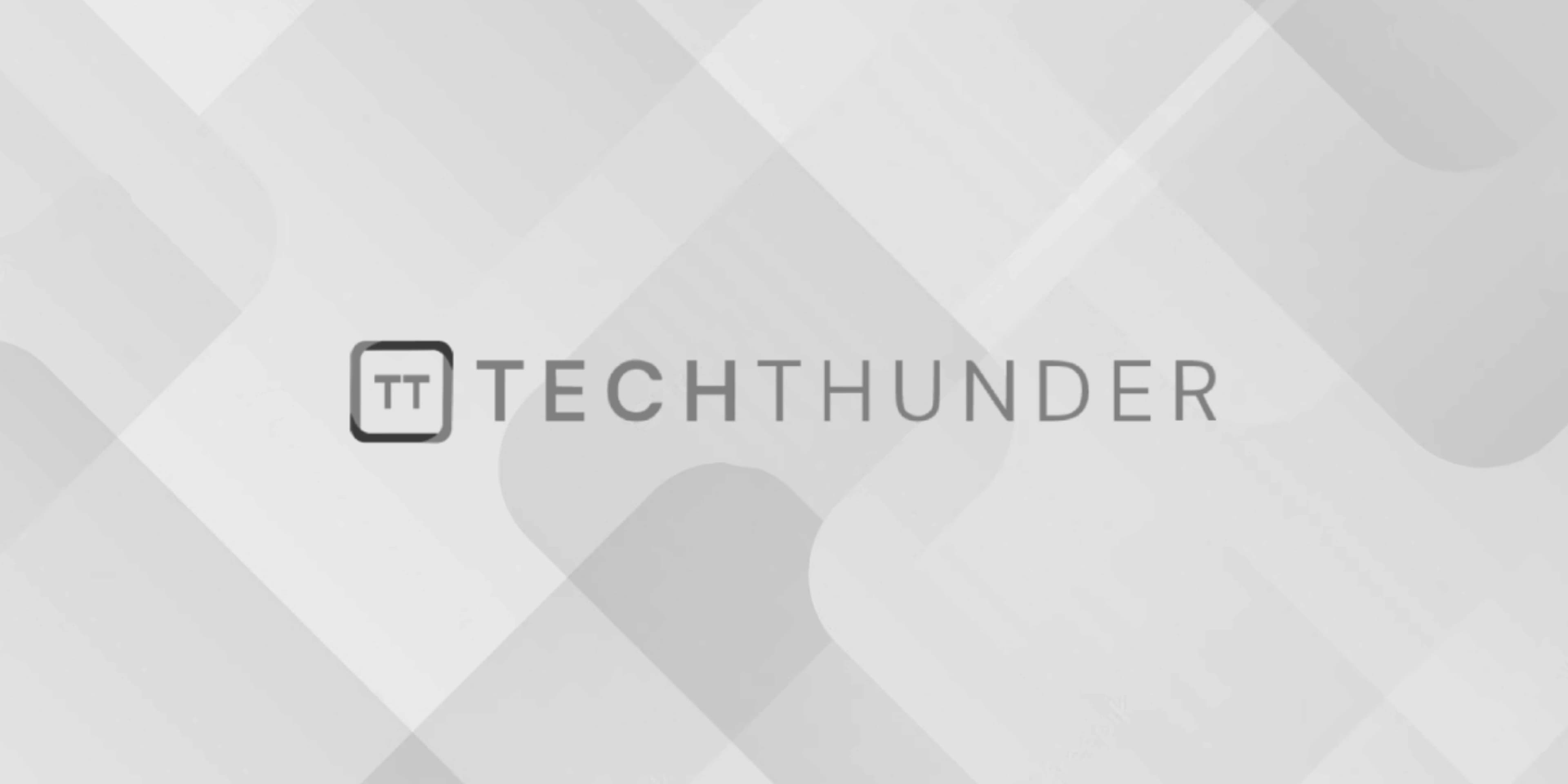
PHP Print
In PHP, print
is another language construct used to output data to the browser or the standard output, similar to the echo
construct. Just like echo
, print
allows you to display text, HTML, or the values of variables.
The basic syntax of print
is:
print expression;
Here, the expression
can be a string (enclosed in quotes) or a variable containing the value you want to display.
The main difference between print
and echo
is that print
returns a value of 1, while echo
does not have a return value.
Examples:
- Displaying a String:
<?php
print "Hello, World!";
?>
Output: Hello, World!
- Displaying Variables:
<?php
$name = "John";
$age = 30;
print "My name is " . $name . " and I am " . $age . " years old.";
?>
Output: My name is John and I am 30 years old.
- Displaying HTML:
<?php
print "<h1>Welcome to My Website</h1>";
print "<p>This is a paragraph of text.</p>";
?>
Output:
Welcome to My Website
This is a paragraph of text.
- Displaying the Result of an Expression:
<?php
$number1 = 10;
$number2 = 5;
$result = $number1 + $number2;
print "The sum of $number1 and $number2 is: " . $result;
?>
Output: The sum of 10 and 5 is: 15
Just like echo
, you can use single quotes or double quotes to define strings with print
. The choice depends on whether you need variable interpolation or not.
Remember to use semicolons at the end of each print
statement to terminate the PHP statement properly.
In most cases, developers prefer using echo
over print
because it is slightly faster and widely used in PHP codebases. However, both echo
and print
are suitable for displaying output, and you can choose whichever you find more comfortable or consistent with your coding style.