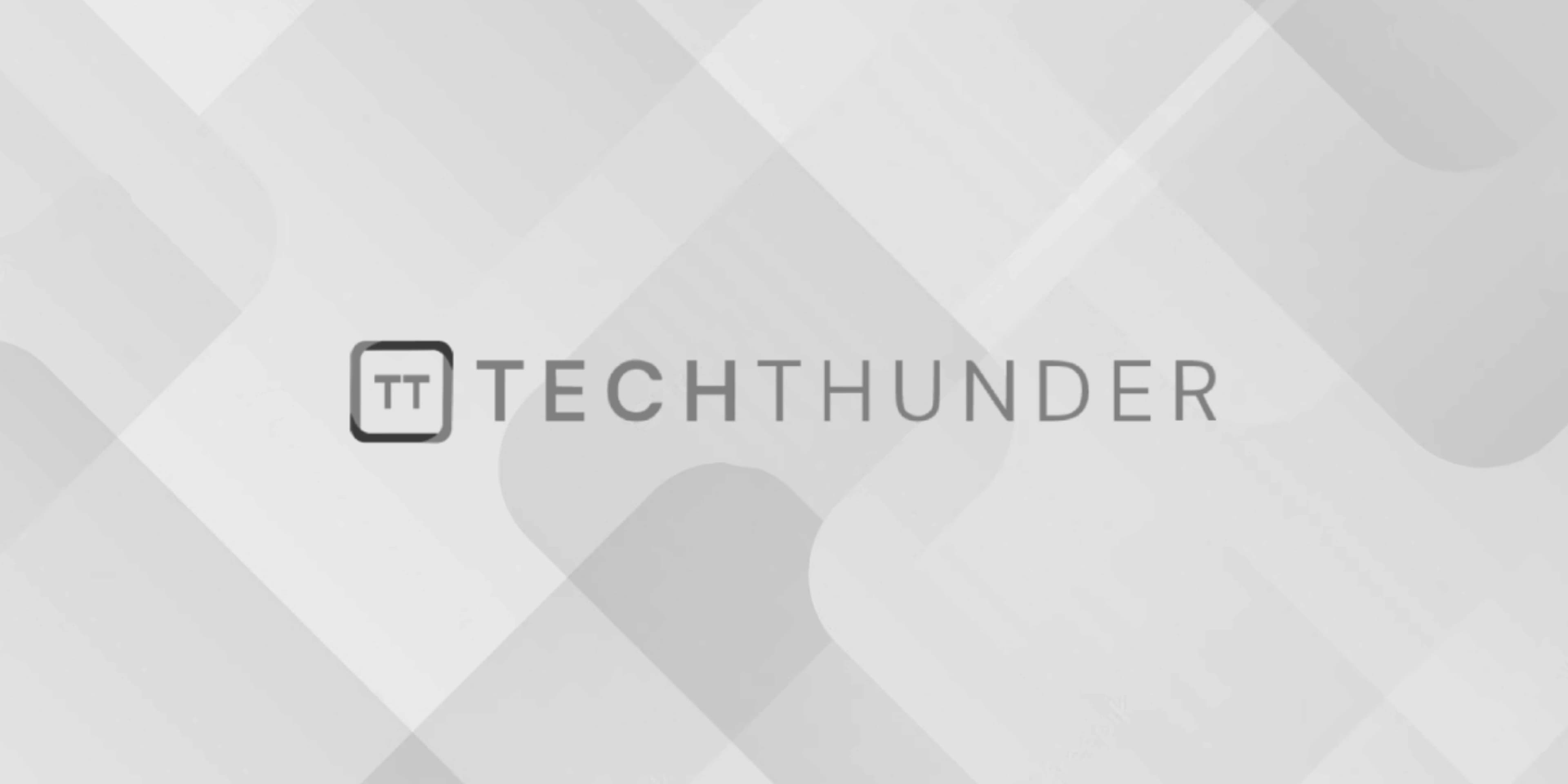
PHP Continue
In PHP, the continue
statement is used within loop structures (such as for
, while
, and do-while
loops) to skip the current iteration of the loop and continue with the next iteration.
When the continue
statement is encountered, the remaining code within the loop for the current iteration is skipped, and the loop proceeds with the next iteration.
Let’s see how continue
works in different loop contexts:
- Using
continue
in a Loop:
for ($i = 1; $i <= 5; $i++) {
if ($i == 3) {
continue; // Skip the current iteration when $i equals 3
}
echo $i . " ";
}
Output:
1 2 4 5
In the example above, the for
loop iterates from 1 to 5. When $i
is equal to 3, the continue
statement is executed, and the current iteration is skipped. The loop then proceeds with the next iteration.
- Using
continue
in a While Loop:
$i = 1;
while ($i <= 5) {
if ($i == 3) {
$i++;
continue; // Skip the current iteration when $i equals 3
}
echo $i . " ";
$i++;
}
Output:
1 2 4 5
In the example above, the while
loop runs while $i
is less than or equal to 5. When $i
is equal to 3, the continue
statement is executed, and the current iteration is skipped. We explicitly increment $i
within the loop to avoid an infinite loop due to the skipped iteration.
The continue
statement is useful when you want to bypass certain iterations of a loop based on specific conditions. It allows you to control the flow of your loop and proceed to the next iteration without executing the remaining code in the current iteration.