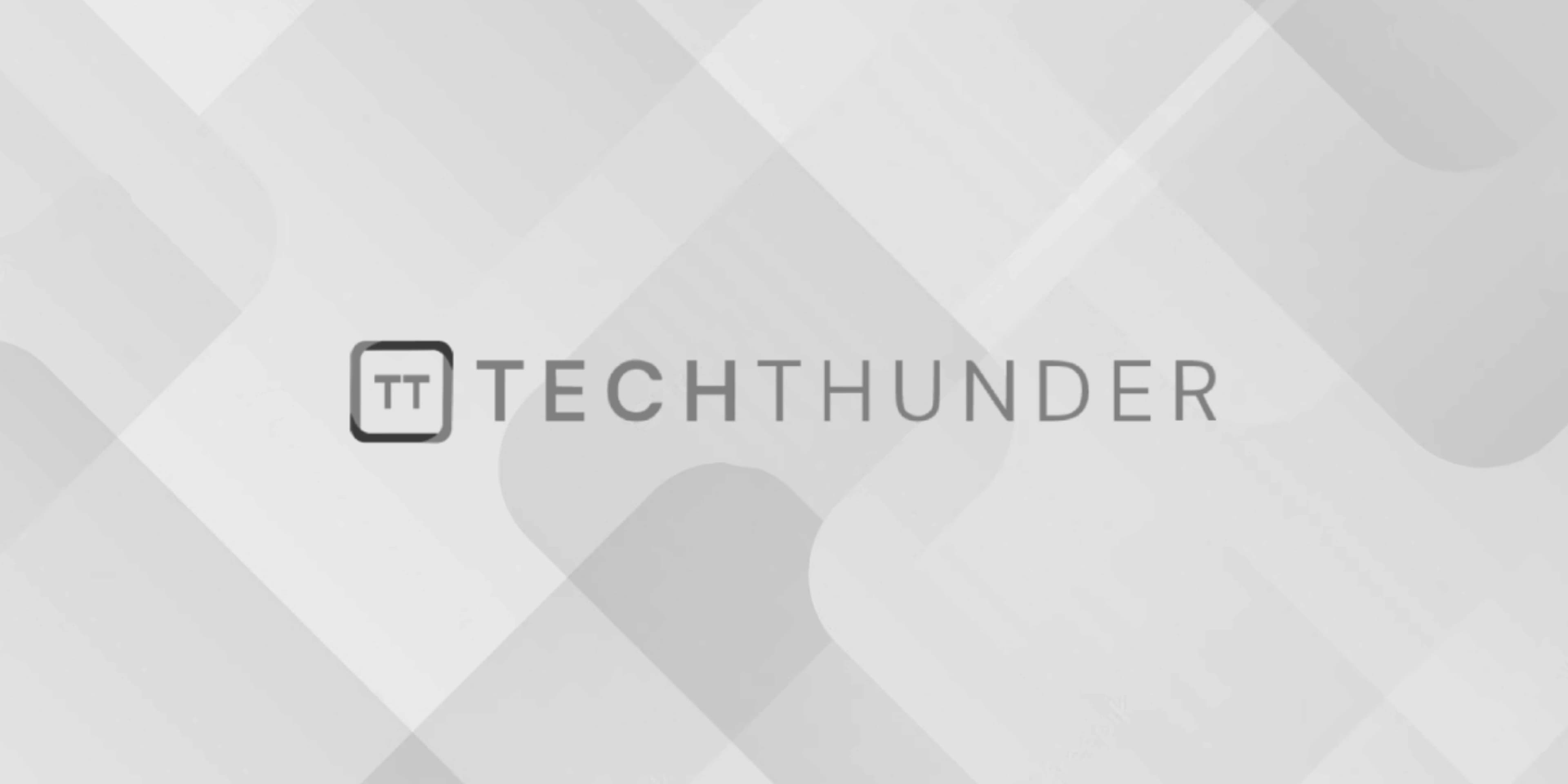
PHP Break
In PHP, the break
statement is used within loop structures (such as for
, while
, and do-while
loops) and switch
statements to terminate the loop or switch block prematurely.
When the break
statement is encountered, the control immediately exits the loop or switch block, and the program continues executing the code after the loop or switch block.
Let’s see how break
works in different contexts:
- Using
break
in a Loop:
for ($i = 1; $i <= 5; $i++) {
echo $i . " ";
if ($i == 3) {
break; // Terminate the loop when $i equals 3
}
}
Output:
1 2 3
In the example above, the for
loop iterates from 1 to 5. When $i
becomes equal to 3, the break
statement is executed, and the loop terminates immediately.
- Using
break
in a Switch Statement:
$day = 'Wednesday';
switch ($day) {
case 'Monday':
echo 'Today is Monday';
break;
case 'Tuesday':
echo 'Today is Tuesday';
break;
case 'Wednesday':
echo 'Today is Wednesday';
break; // Terminate the switch block when $day is 'Wednesday'
default:
echo 'Today is not Monday, Tuesday, or Wednesday';
break;
}
Output:
Today is Wednesday
In the example above, the switch
block checks the value of $day
and executes the corresponding case. When $day
is ‘Wednesday’, the break
statement inside the corresponding case is executed, and the switch block terminates.
The break
statement is useful when you want to exit a loop early based on a specific condition or when you want to stop the execution of further case blocks in a switch
statement once a match is found. It allows you to control the flow of your code within loops and switch statements.