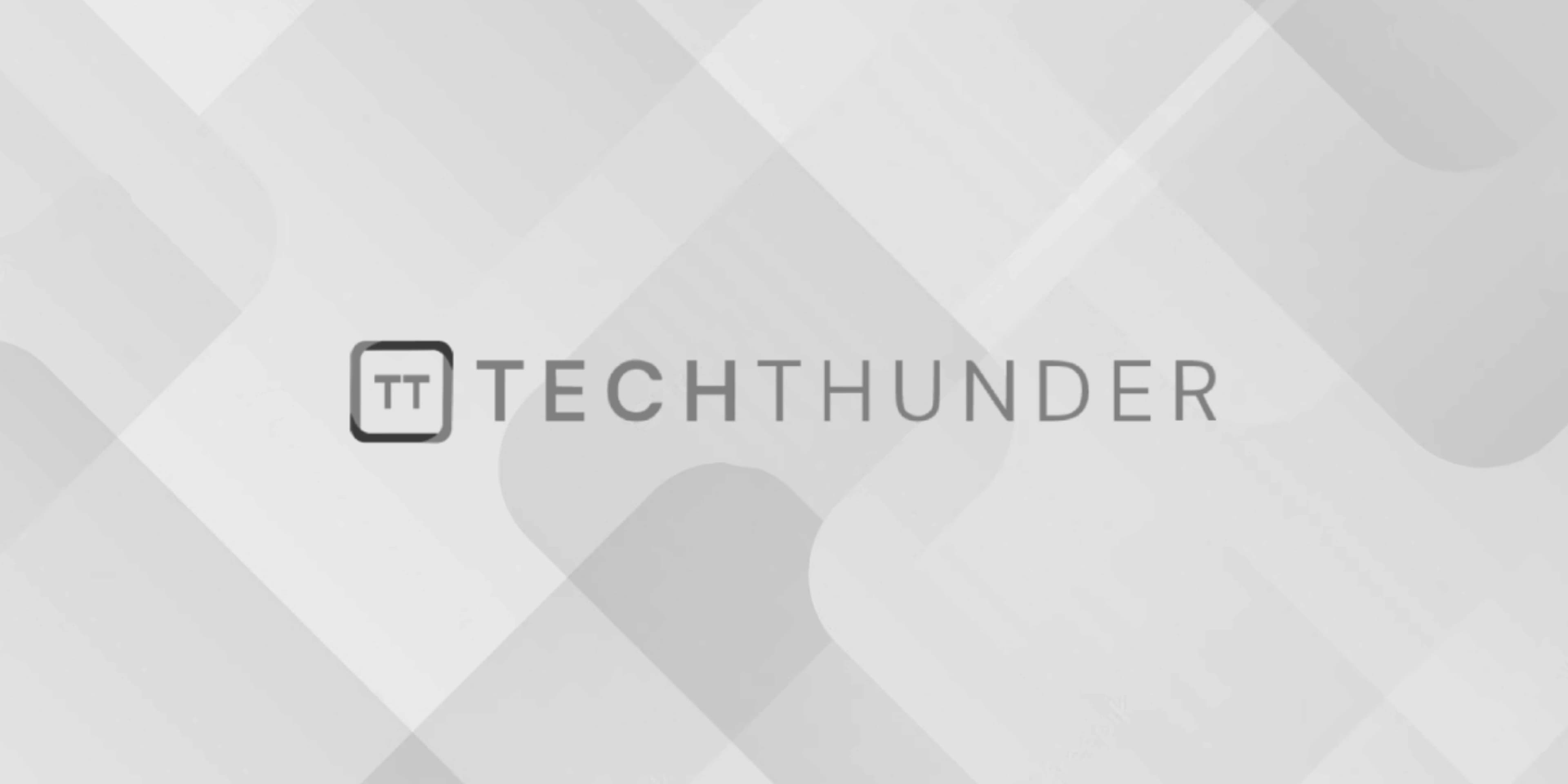
PHP Boolean
In PHP, a boolean is a data type that represents a logical value, which can be either true
or false
. Booleans are often used in conditional statements and logical expressions to control the flow of a program based on the evaluation of certain conditions.
In PHP, the boolean literals are case-insensitive, meaning you can use true
, false
, TRUE
, or FALSE
interchangeably.
Here are some examples of using booleans in PHP:
$hasPermission = true; // A variable storing the boolean value true
$isLoggedOut = false; // A variable storing the boolean value false
// Using boolean values in conditional statements
if ($hasPermission) {
echo "You have permission to access this resource.\n";
} else {
echo "Access denied. You don't have permission.\n";
}
if (!$isLoggedOut) {
echo "You are still logged in.\n";
} else {
echo "You are logged out.\n";
}
Output:
You have permission to access this resource.
You are still logged in.
In the example above, we have two boolean variables, $hasPermission
and $isLoggedOut
. We use these variables in conditional statements to check whether a user has permission to access a resource and whether the user is still logged in.
Boolean values are essential for controlling the flow of your PHP scripts based on conditions. They are commonly used with comparison operators, logical operators, and conditional statements like if
, else
, while
, for
, etc.
In PHP, various functions and operators return boolean values. For example, comparison operators like ==
, !=
, >
, <
, >=
, <=
return booleans when comparing values. Logical operators like &&
(AND), ||
(OR), and !
(NOT) are also used to create complex boolean expressions.
$number = 42;
// Using comparison operators to get boolean results
$isEqual = ($number == 42); // true
$isNotEqual = ($number != 42); // false
$isGreater = ($number > 50); // false
$isLess = ($number < 50); // true
// Using logical operators to combine conditions
$isBetween = ($number > 30 && $number < 60); // true
$isEitherOr = ($number < 10 || $number > 90); // false
$isNotEqualAndBetween = ($number != 42 && ($number > 30 && $number < 60)); // false
These boolean values are then used in conditional statements to control the flow of the program based on the evaluation of these conditions.