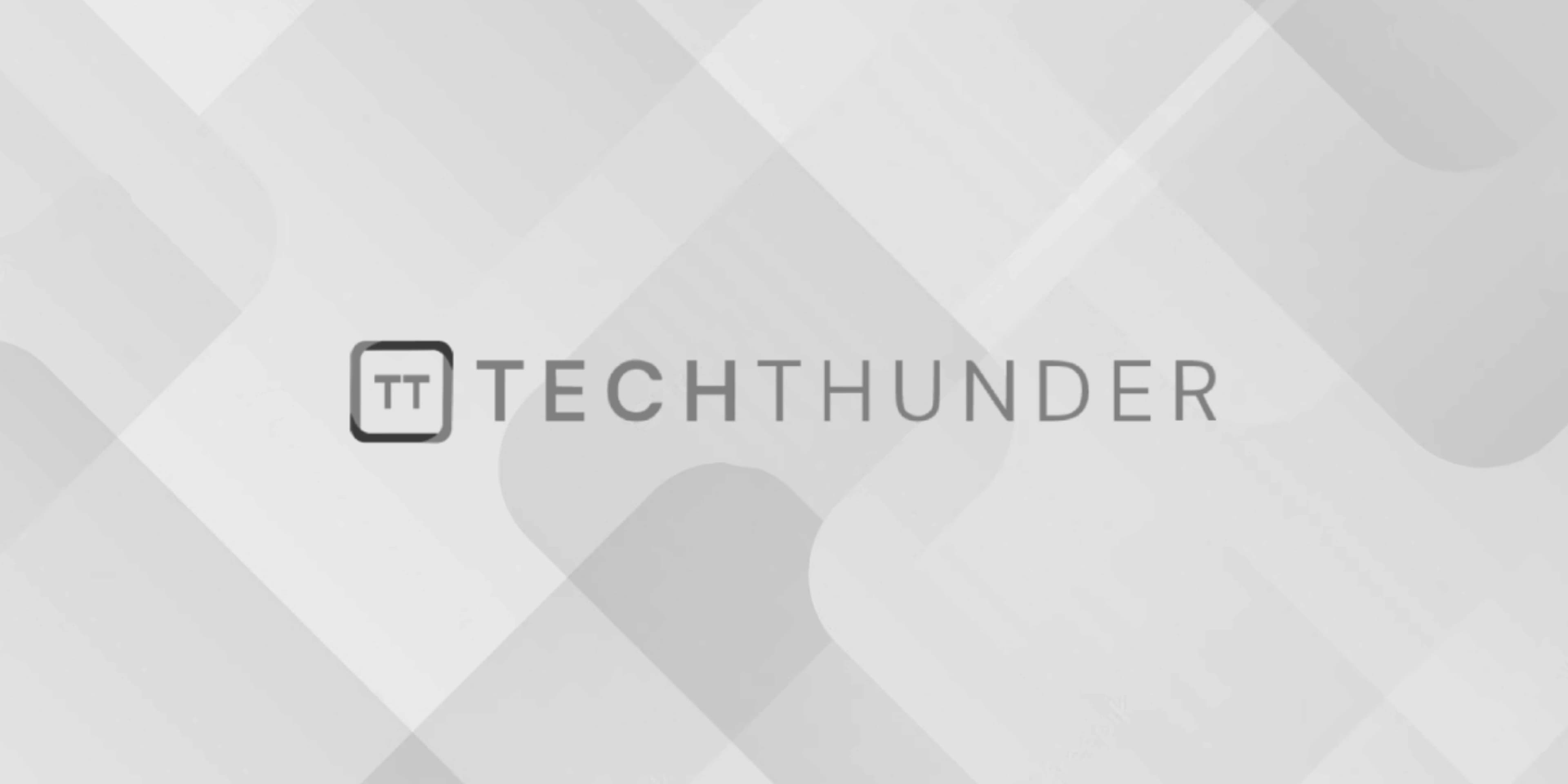
PHP Imagearc() Function
In PHP, imagearc()
is a function used to draw an elliptical arc on an image created with the GD (GIF Draw) library. The GD library is commonly used in PHP for creating and manipulating images.
Here’s the syntax of the imagearc()
function:
bool imagearc(resource $image, int $cx, int $cy, int $width, int $height, int $start, int $end, int $color)
Parameters:
$image
: The GD image resource obtained from functions likeimagecreate()
orimagecreatetruecolor()
.$cx
: The x-coordinate (horizontal position) of the center of the ellipse.$cy
: The y-coordinate (vertical position) of the center of the ellipse.$width
: The width of the ellipse.$height
: The height of the ellipse.$start
: The start angle of the arc in degrees (0° is at the 3 o’clock position).$end
: The end angle of the arc in degrees (0° is at the 3 o’clock position).$color
: The color identifier obtained from functions likeimagecolorallocate()
.
Return value:
- The function returns
true
on success orfalse
on failure.
Example:
// Create a new true-color image with width 400 pixels and height 200 pixels
$imageWidth = 400;
$imageHeight = 200;
$image = imagecreatetruecolor($imageWidth, $imageHeight);
// Allocate colors for the background (white) and arc (blue)
$backgroundColor = imagecolorallocate($image, 255, 255, 255); // White
$arcColor = imagecolorallocate($image, 0, 0, 255); // Blue
// Set the background color
imagefill($image, 0, 0, $backgroundColor);
// Draw a blue elliptical arc on the image
$cx = 200; // Center x-coordinate
$cy = 100; // Center y-coordinate
$width = 300; // Width of the ellipse
$height = 100; // Height of the ellipse
$start = 0; // Start angle in degrees
$end = 180; // End angle in degrees (180° = half circle)
imagearc($image, $cx, $cy, $width, $height, $start, $end, $arcColor);
// Set the content type header to output the image as PNG
header('Content-Type: image/png');
// Output the image as PNG to the browser
imagepng($image);
// Free up memory by destroying the image resource
imagedestroy($image);
In this example, we create a new true-color image with a width of 400 pixels and a height of 200 pixels. We allocate colors for the background (white) and the elliptical arc (blue). We set the background color for the entire image using imagefill()
. Then, we draw a blue elliptical arc on the image using imagearc()
with the specified center coordinates, width, height, start angle, and end angle. Finally, we output the image as PNG to the browser using imagepng()
and free up memory by destroying the image resource with imagedestroy()
.
The imagearc()
function is useful for drawing elliptical arcs, which can be part of more complex graphics and illustrations created with GD.