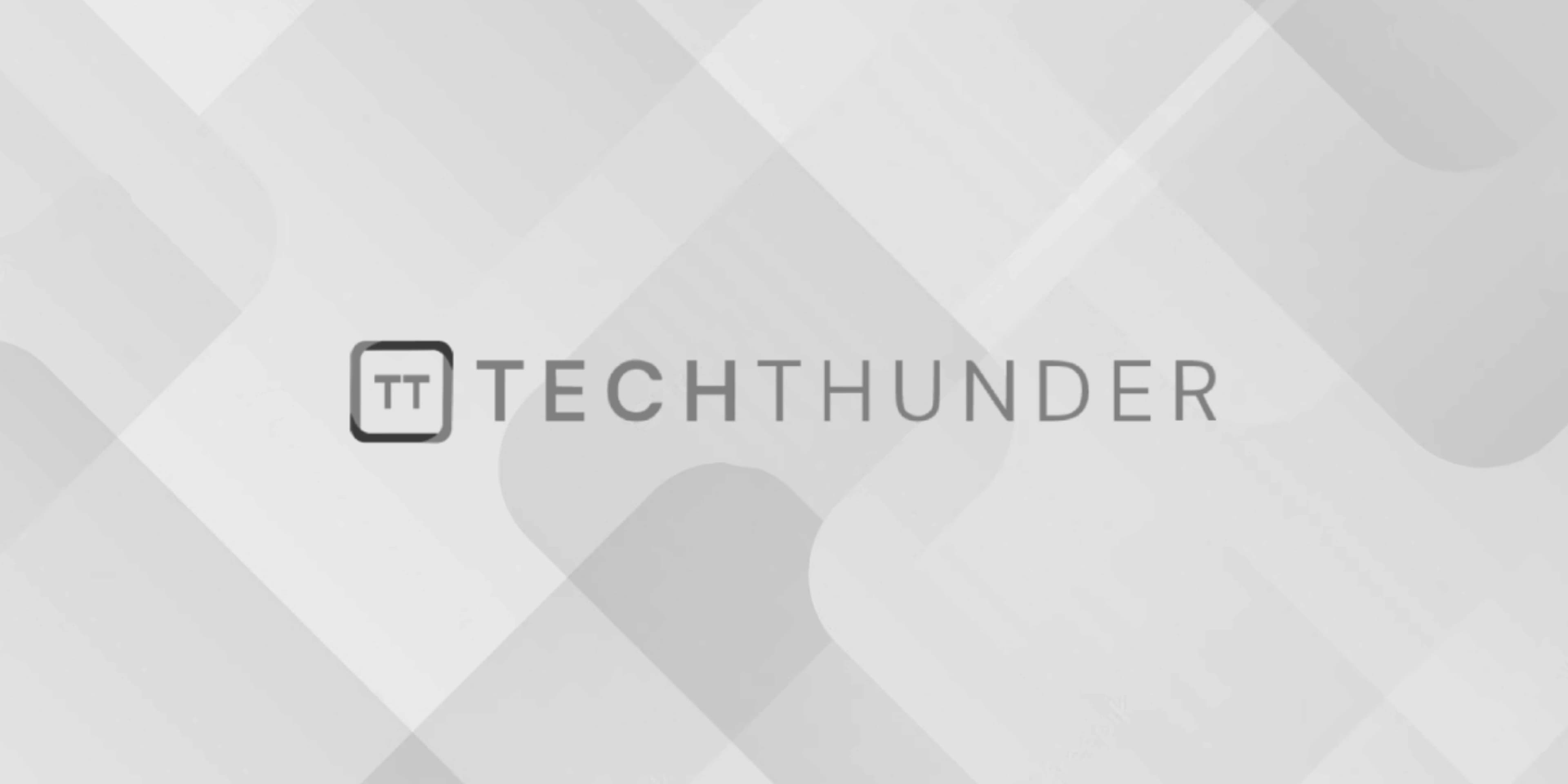
PHP MySQLi SELECT
In PHP, you can use MySQLi (MySQL Improved) to retrieve data from a MySQL database table. To perform a SELECT operation, follow these steps:
- Connect to the MySQL Database:
First, establish a connection to the MySQL database using themysqli_connect()
function, as shown in the INSERT, UPDATE, and DELETE examples.
<?php
$servername = "localhost";
$username = "your_username";
$password = "your_password";
$dbname = "your_database";
// Create a connection
$conn = mysqli_connect($servername, $username, $password, $dbname);
// Check the connection
if (!$conn) {
die("Connection failed: " . mysqli_connect_error());
}
?>
- Prepare and Execute the SELECT Query:
Next, prepare and execute a SELECT query to retrieve data from the database table. Use themysqli_query()
function to execute the query and obtain a result set.
<?php
// Prepare the SELECT query
$sql = "SELECT id, name, email FROM users";
// Execute the query
$result = mysqli_query($conn, $sql);
// Check if the query was successful
if ($result) {
// Fetch the data from the result set
while ($row = mysqli_fetch_assoc($result)) {
echo "ID: " . $row['id'] . ", Name: " . $row['name'] . ", Email: " . $row['email'] . "<br>";
}
} else {
echo "Error: " . $sql . "<br>" . mysqli_error($conn);
}
?>
In this example, we are retrieving data from the “users” table, displaying the ID, name, and email of each user.
- Close the Database Connection:
After retrieving the data, close the database connection using themysqli_close()
function to free up resources.
<?php
// Close the connection
mysqli_close($conn);
?>
As with the previous examples, always use proper data sanitization and validation techniques to prevent SQL injection and other security issues. Prepared statements or parameterized queries are safer methods to interact with the database.
Important Note: When building dynamic SQL queries with user input, use prepared statements or escape user input using functions like mysqli_real_escape_string()
to prevent SQL injection attacks. Never directly embed user input in the SQL query. Additionally, consider limiting the data returned in the SELECT query using WHERE clauses or pagination to improve performance and avoid unnecessary data retrieval.