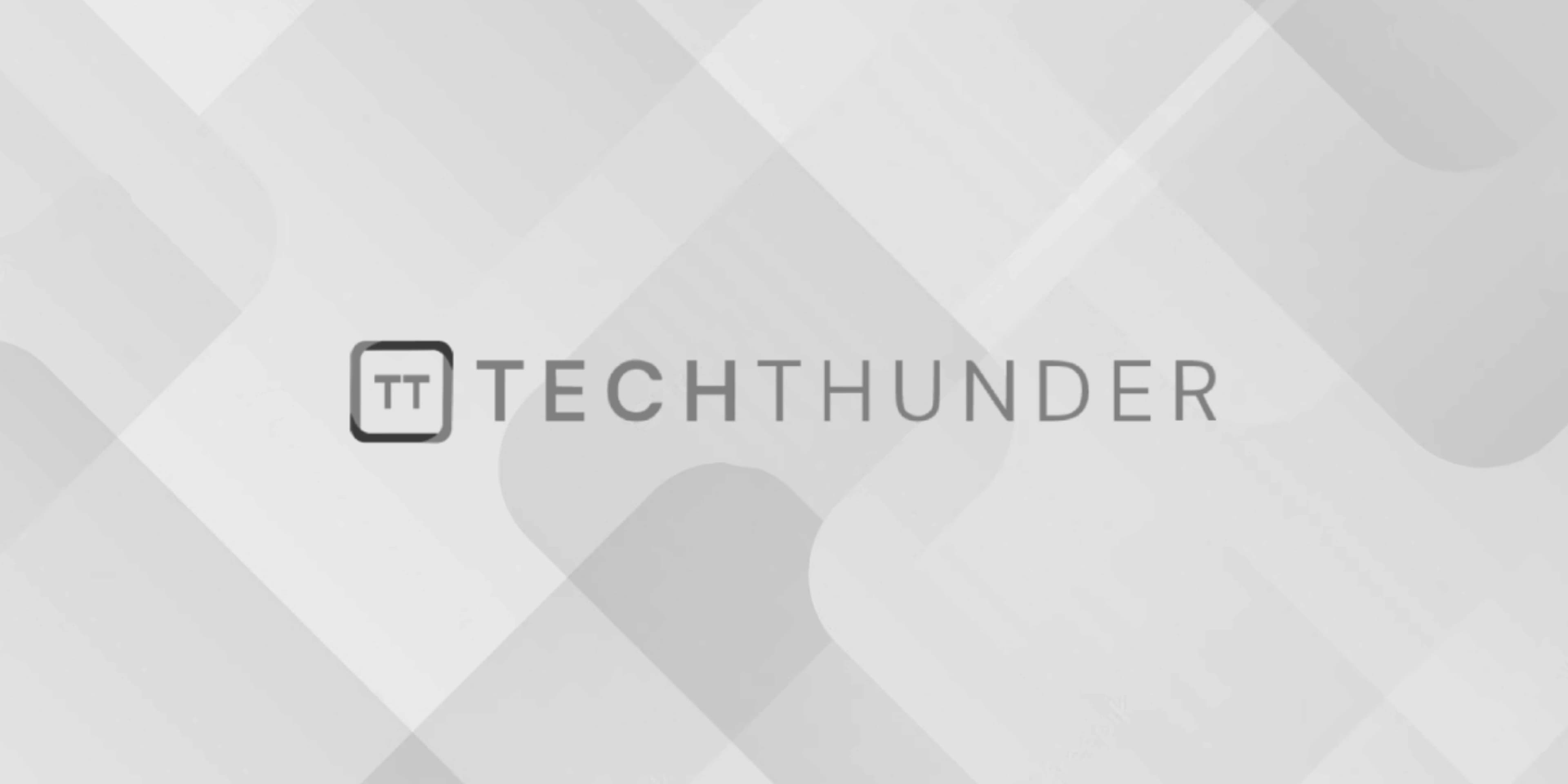
OOPs Interface
In object-oriented programming (OOP), an interface is a programming construct that defines a contract for a class. It specifies a set of methods (and possibly properties) that a class implementing the interface must provide. An interface defines what a class can do, but it doesn’t contain the actual implementation of those methods.
In OOP, an interface is a way to achieve abstraction and establish a common understanding between classes, allowing them to interact with each other without knowing the specifics of each class’s implementation.
In many programming languages that support OOP, including Java, C#, and TypeScript, you can define an interface using the interface
keyword. Here’s an example in TypeScript:
interface Shape {
getArea(): number;
getPerimeter(): number;
}
In this example, the Shape
interface defines two methods, getArea()
and getPerimeter()
, that any class implementing the Shape
interface must provide. The interface doesn’t contain the implementation details of these methods but sets the requirement for any class that claims to be a Shape
.
To implement an interface in a class, you use the implements
keyword. Here’s an example:
class Rectangle implements Shape {
private width: number;
private height: number;
constructor(width: number, height: number) {
this.width = width;
this.height = height;
}
getArea(): number {
return this.width * this.height;
}
getPerimeter(): number {
return 2 * (this.width + this.height);
}
}
In this example, the Rectangle
class implements the Shape
interface, which means it must provide the getArea()
and getPerimeter()
methods as required by the Shape
interface.
Interfaces help promote code reusability and maintainability by allowing you to create classes that adhere to a specific contract without being tightly coupled to each other’s implementation. They enable polymorphism, where different classes can be treated as instances of a common interface, allowing for more flexible and scalable code structures.