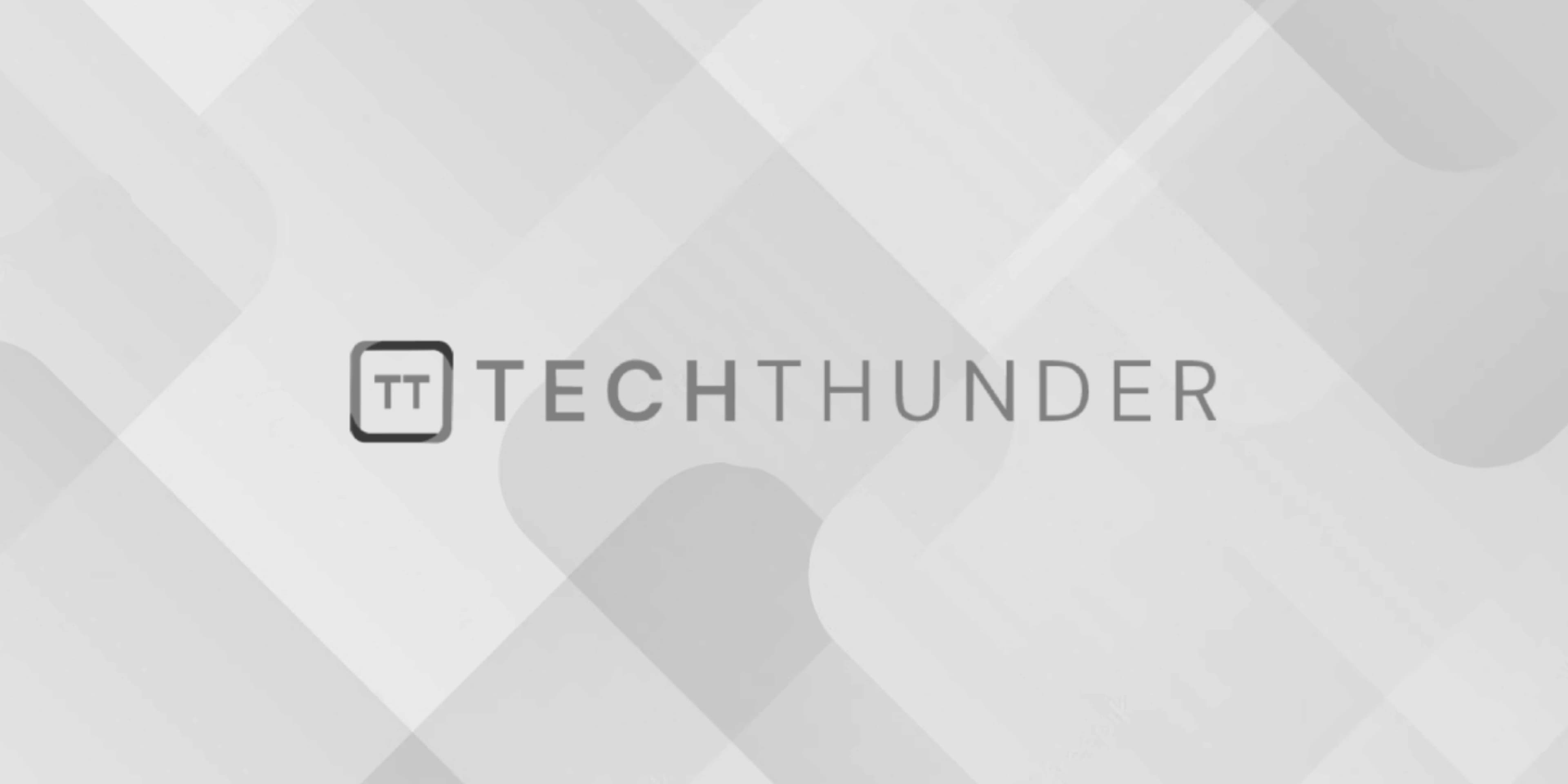
PHP Parameterized Function
In PHP, a parameterized function is a function that accepts one or more parameters (arguments) to perform specific tasks based on the values passed to it. These parameters act as placeholders for the values that will be supplied when calling the function.
To create a parameterized function in PHP, you define the function with parameter names within the parentheses in the function declaration. When the function is called, you provide values for those parameters, and the function uses those values to perform its operations.
Here’s the syntax for creating a parameterized function in PHP:
function functionName(parameter1, parameter2, ...) {
// Function code that uses the parameters
}
Example of a Simple Parameterized Function:
function greet($name) {
echo "Hello, " . $name . "!";
}
// Call the function with a parameter
greet("John"); // Output: Hello, John!
In the example above, we define the function greet
with one parameter $name
. When we call the function using greet("John")
, the value “John” is passed as an argument to the $name
parameter, and the function uses it to greet the person.
You can create functions with multiple parameters as well:
function add($num1, $num2) {
$sum = $num1 + $num2;
echo "The sum of " . $num1 . " and " . $num2 . " is: " . $sum;
}
// Call the function with two parameters
add(5, 10); // Output: The sum of 5 and 10 is: 15
In this example, we define the function add
with two parameters $num1
and $num2
. When we call the function using add(5, 10)
, the values 5 and 10 are passed as arguments to $num1
and $num2
respectively, and the function calculates their sum and outputs the result.
Parameterized functions are powerful because they allow you to create flexible and reusable code that can perform different tasks based on the input values provided to them. By using parameters, you can pass data to functions and make your code more dynamic and adaptable to various scenarios.