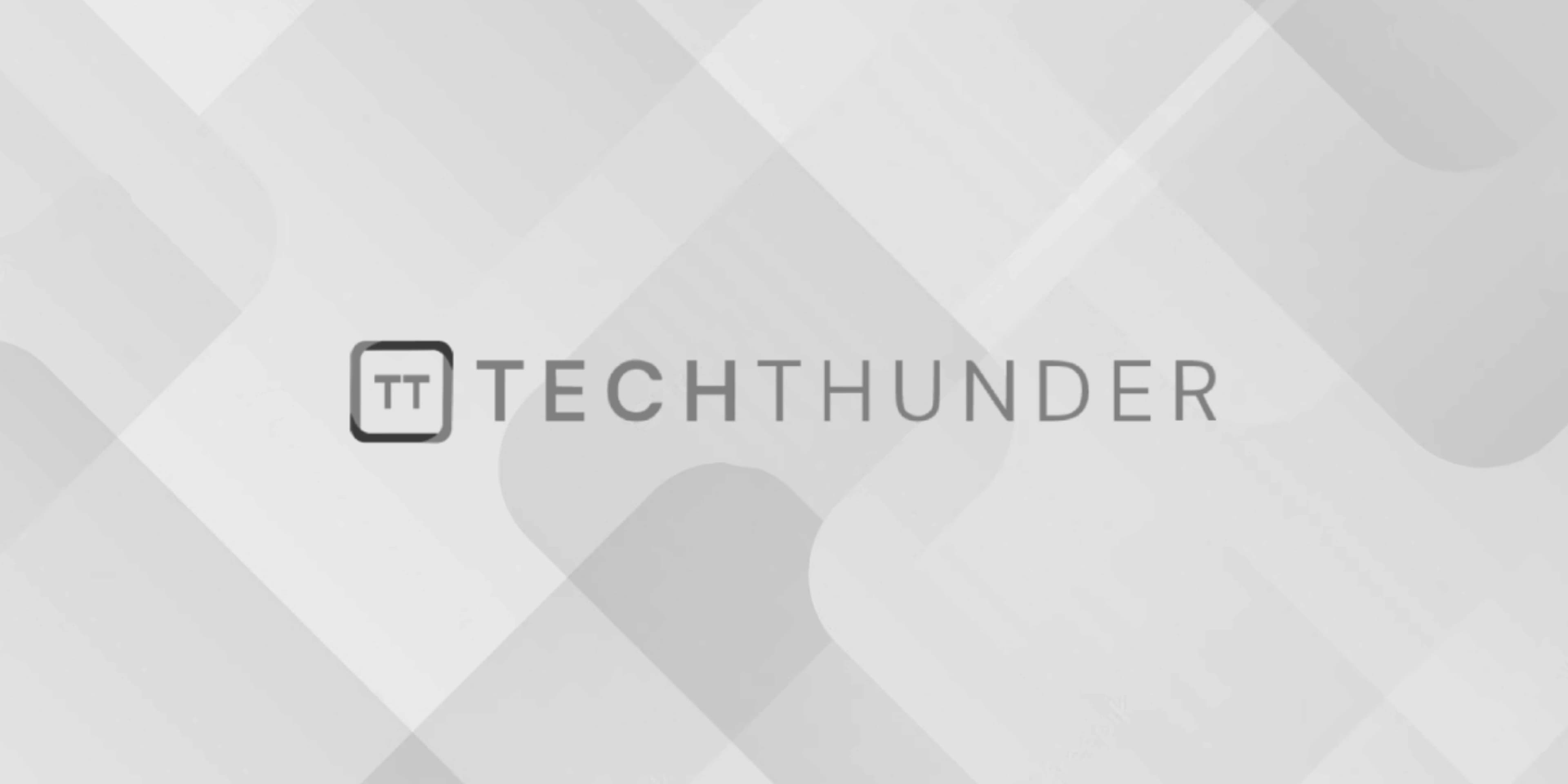
OOPs Abstraction
Abstraction is a fundamental concept in object-oriented programming (OOP) that focuses on simplifying complex systems by presenting only essential information and hiding unnecessary details. It allows you to create abstract representations of real-world entities, known as classes, which can be used to create objects with specific attributes and behaviors. Abstraction provides a clear separation between the external view of an object and its internal implementation, allowing developers to work at a higher level of understanding.
In OOP, abstraction is achieved through two main mechanisms:
- Abstract Classes: An abstract class is a class that cannot be instantiated directly, meaning you cannot create objects directly from it. Instead, an abstract class serves as a blueprint for other classes and may contain abstract methods (methods without an implementation) that its subclasses must implement. Abstract classes define a common interface and set of attributes that derived classes share, but they leave the specific implementation details to be provided by the subclasses. Abstract classes provide a level of abstraction, allowing developers to work with generic concepts and establish common behavior for a group of related classes.
- Interfaces: An interface is a collection of abstract methods that define a contract for a class. Unlike abstract classes, an interface cannot contain any implementation details, only method signatures. Classes that implement an interface must provide concrete implementations of all the methods declared in the interface. Interfaces are useful for creating a common understanding among classes that may not have a direct hierarchical relationship but need to adhere to a specific contract. They enable polymorphism, where different classes can be treated as instances of a common interface, providing flexibility in the code.
Example of Abstraction using Abstract Class:
abstract class Animal {
abstract makeSound(): void;
move(): void {
console.log("Animal is moving.");
}
}
class Dog extends Animal {
makeSound(): void {
console.log("Dog barks.");
}
}
class Cat extends Animal {
makeSound(): void {
console.log("Cat meows.");
}
}
const dog = new Dog();
dog.makeSound(); // Output: Dog barks.
dog.move(); // Output: Animal is moving.
const cat = new Cat();
cat.makeSound(); // Output: Cat meows.
cat.move(); // Output: Animal is moving.
In this example, Animal
is an abstract class with an abstract method makeSound()
and a concrete method move()
. The Dog
and Cat
classes extend Animal
and implement the makeSound()
method with their specific behavior. When calling the makeSound()
and move()
methods on instances of Dog
and Cat
, we see polymorphism in action as the appropriate behavior is invoked.
Abstraction helps in designing cleaner and more maintainable code by separating concerns and providing a higher-level view of complex systems. It allows developers to work with abstract concepts rather than getting lost in low-level implementation details, leading to more flexible and extensible software.