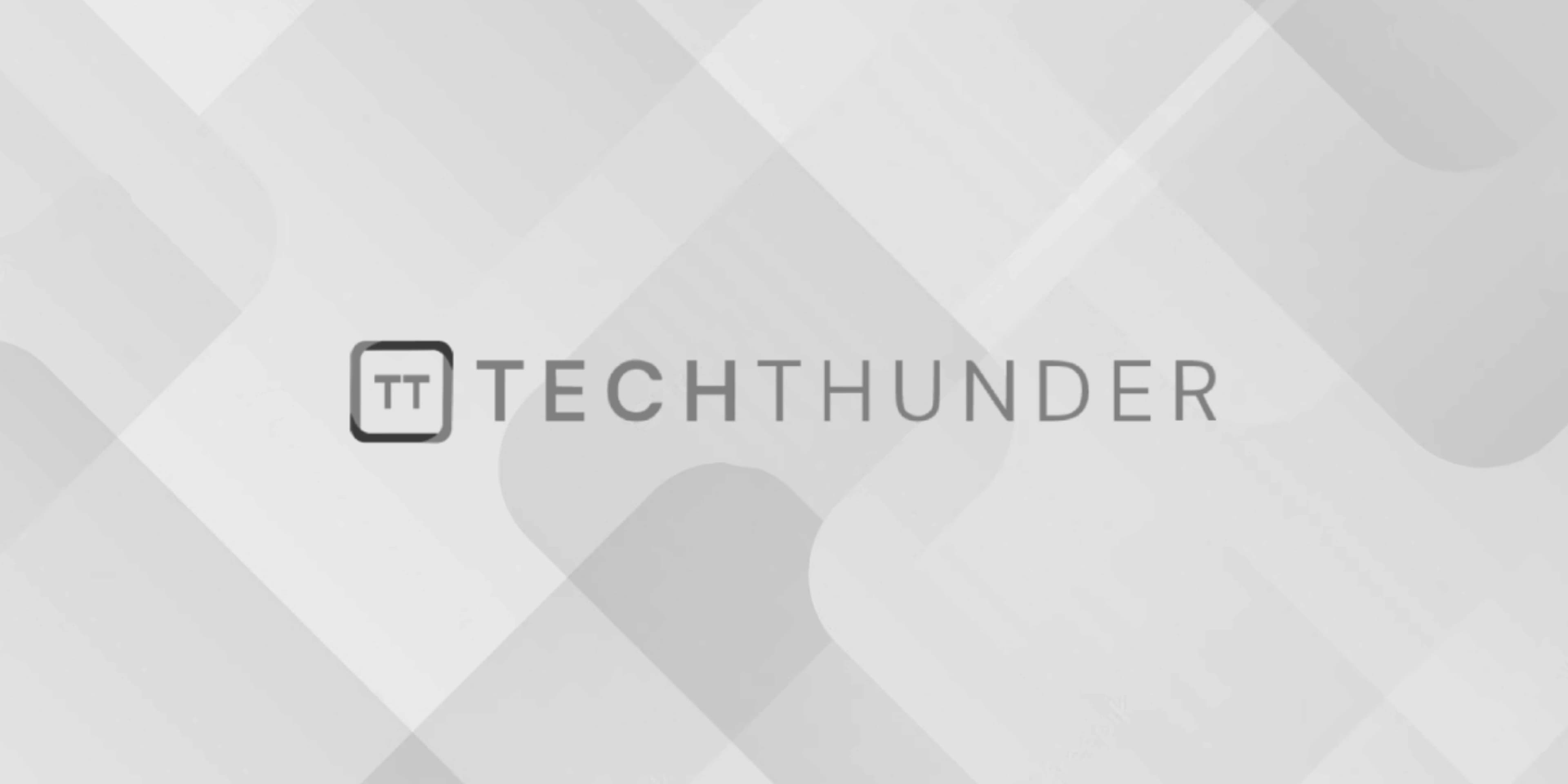
GET vs POST method in PHP
In PHP, as well as in web development in general, the two most commonly used HTTP methods for submitting data to a server are GET and POST. These methods are used to transfer data from the client (usually a web browser) to the server, but they differ in how the data is sent and where it is visible.
- GET method:
- When using the GET method, data is appended to the URL as query parameters.
- The data is visible in the URL, making it less secure for sensitive information.
- The data is limited in size due to URL length restrictions.
- GET requests can be bookmarked and cached by browsers.
- It is typically used for fetching data from the server, like displaying search results or passing parameters to a page.
- In PHP, you can access the data sent via GET using the
$_GET
superglobal array.
Example of a GET request:
http://example.com/page.php?name=John&age=30
- POST method:
- When using the POST method, data is sent in the request body, and it is not visible in the URL.
- The data is more secure for sensitive information since it is not directly visible.
- There is no size limitation for data sent using POST.
- POST requests are not cached or bookmarked by browsers.
- It is typically used for submitting data to the server, like submitting a form with user input.
- In PHP, you can access the data sent via POST using the
$_POST
superglobal array.
Example of a POST request:
<form method="post" action="page.php">
<input type="text" name="name" value="John">
<input type="number" name="age" value="30">
<input type="submit" value="Submit">
</form>
In PHP, you can check which method was used to submit data using the $_SERVER['REQUEST_METHOD']
variable. For example:
if ($_SERVER['REQUEST_METHOD'] === 'GET') {
// Handle GET request
// Access data with $_GET
} elseif ($_SERVER['REQUEST_METHOD'] === 'POST') {
// Handle POST request
// Access data with $_POST
}
In summary, use the GET method for retrieving data from the server, especially when the data is not sensitive and can be cached or bookmarked. Use the POST method for sending data to the server, particularly when handling sensitive information or when the data exceeds the URL length limitations of GET.