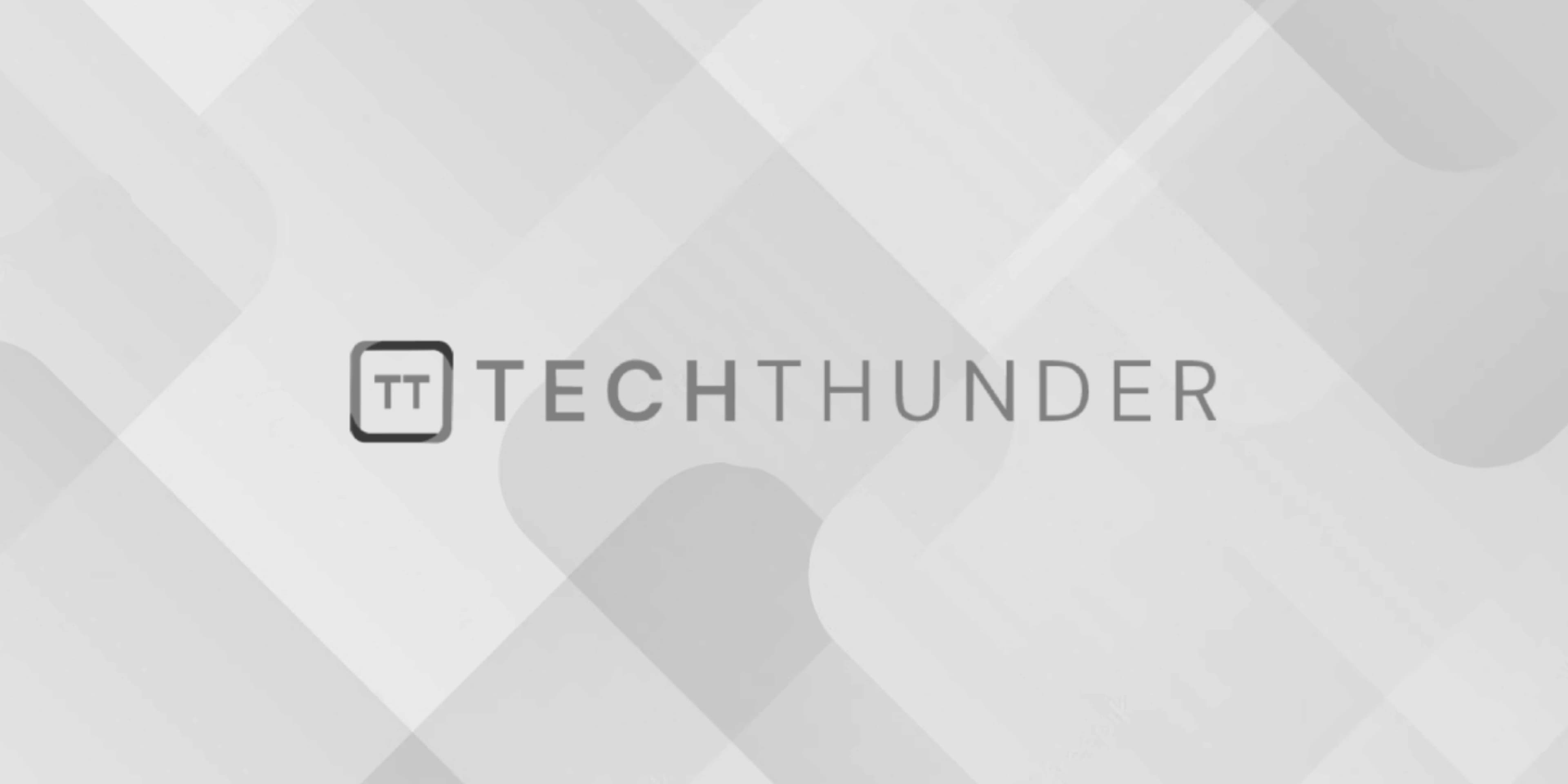
PHP Codeigniter 3 Ajax Pagination using Jquery
To implement Ajax pagination in CodeIgniter 3 using jQuery, you’ll need to create a CodeIgniter controller, model, view, and JavaScript (jQuery) script. Below is a step-by-step guide to achieve this:
- Set up CodeIgniter Project:
Make sure you have CodeIgniter 3 installed and set up on your server. You should have a working folder structure with theapplication
,system
, andassets
directories. - Create Database Table:
Assuming you have a table namedposts
in your database with fieldsid
,title
, andcontent
. - Controller (Posts.php):
Create a controller file calledPosts.php
in theapplication/controllers
folder. This controller will handle the Ajax requests and pagination.
<?php
defined('BASEPATH') OR exit('No direct script access allowed');
class Posts extends CI_Controller {
public function __construct() {
parent::__construct();
$this->load->model('Post_model');
}
public function index() {
$this->load->view('posts');
}
public function get_posts($offset = 0) {
$limit = 10; // Number of posts to load per page
$data['posts'] = $this->Post_model->get_posts($limit, $offset);
$data['total_rows'] = $this->Post_model->count_posts();
$this->output->set_content_type('application/json')->set_output(json_encode($data));
}
}
?>
- Model (Post_model.php):
Create a model file calledPost_model.php
in theapplication/models
folder. This model will handle database operations.
<?php
defined('BASEPATH') OR exit('No direct script access allowed');
class Post_model extends CI_Model {
public function get_posts($limit, $offset) {
$this->db->order_by('id', 'DESC');
$this->db->limit($limit, $offset);
return $this->db->get('posts')->result_array();
}
public function count_posts() {
return $this->db->count_all_results('posts');
}
}
?>
- View (posts.php):
Create a view file calledposts.php
in theapplication/views
folder. This view will display the posts and pagination.
<!DOCTYPE html>
<html>
<head>
<title>Posts with Ajax Pagination</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<h2>Posts</h2>
<div id="postList">
<!-- Posts will be dynamically loaded here -->
</div>
<div id="pagination">
<!-- Pagination links will be dynamically loaded here -->
</div>
<script>
$(document).ready(function() {
loadPosts(0); // Load initial set of posts
function loadPosts(offset) {
$.ajax({
url: '<?php echo site_url("posts/get_posts"); ?>/' + offset,
type: 'GET',
dataType: 'json',
success: function(data) {
// Load posts into the postList div
var html = '';
$.each(data.posts, function(index, post) {
html += '<div>';
html += '<h3>' + post.title + '</h3>';
html += '<p>' + post.content + '</p>';
html += '</div>';
});
$('#postList').html(html);
// Load pagination links into the pagination div
var pagination = '';
var totalPages = Math.ceil(data.total_rows / 10); // Assuming 10 posts per page
for (var i = 0; i < totalPages; i++) {
var page = i + 1;
pagination += '<a href="javascript:void(0);" onclick="loadPosts(' + (i * 10) + ')">' + page + '</a> ';
}
$('#pagination').html(pagination);
}
});
}
});
</script>
</body>
</html>
In this example, we use the $.ajax()
function from jQuery to make an Ajax request to the get_posts()
method in the Posts
controller. The controller returns JSON-encoded data containing posts and the total number of rows. The JavaScript function loads the posts into the postList
div and dynamically generates pagination links in the pagination
div. When a pagination link is clicked, it calls the loadPosts()
function with the appropriate offset to fetch the corresponding set of posts.
Note: You may need to adjust the number of posts per page ($limit
in the controller) based on your requirements.
Make sure you have jQuery loaded before the script. Also, configure your CodeIgniter project and database settings properly for it to work correctly.