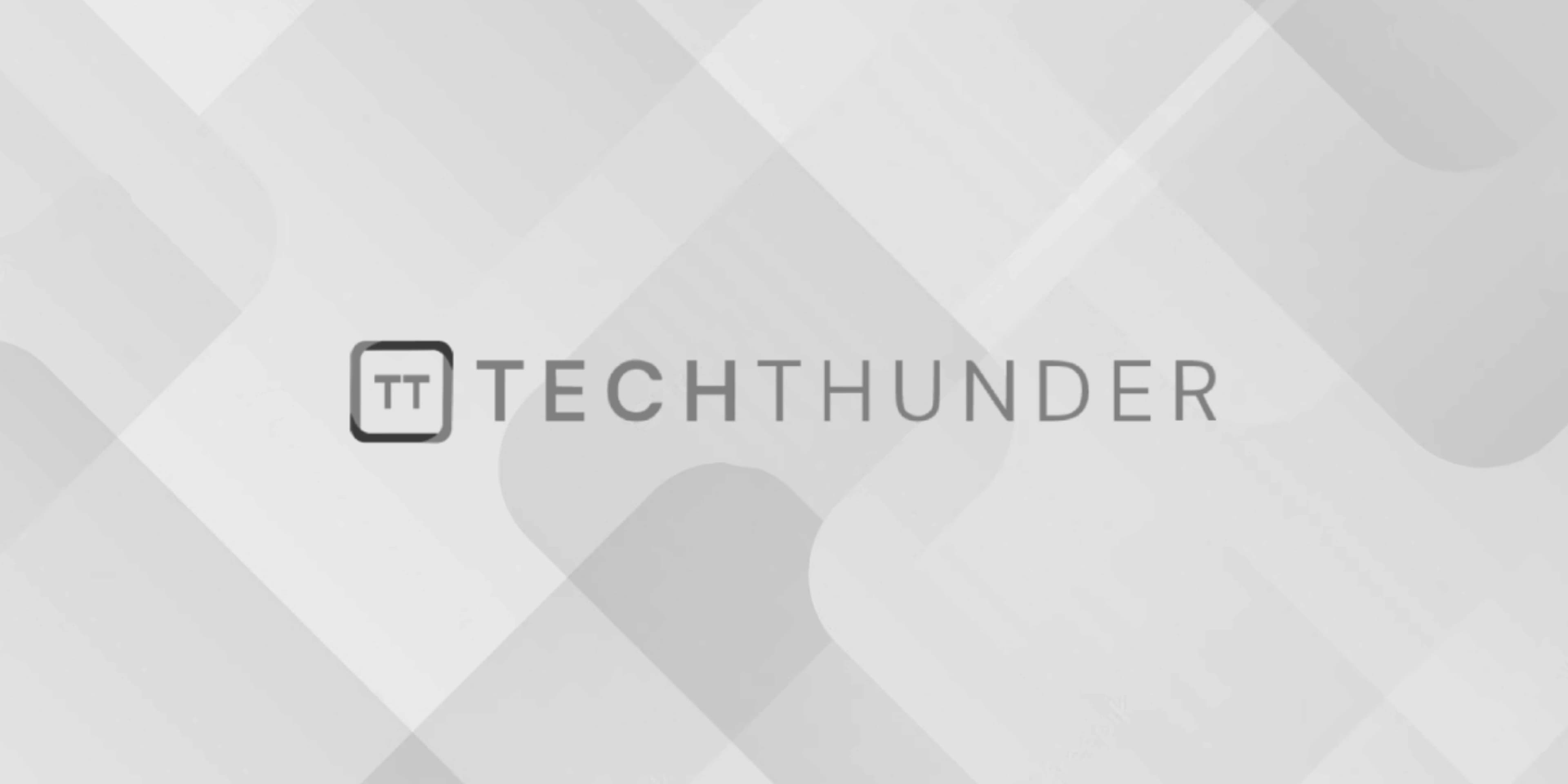
282 views
PHP Special Types
In PHP, there are several special types that represent specific values or conditions. These special types include:
NULL
: TheNULL
type represents a variable with no value or an undefined value. It is used to indicate the absence of a value.resource
: Theresource
type represents a reference to an external resource, such as a file, database connection, or network connection. Resources are typically created and managed by PHP extensions.callable
: Thecallable
type represents a reference to a callable entity, such as a function, method, or closure. It is often used to specify callback functions or callables in various PHP functions.iterable
: Theiterable
type represents any data type that can be iterated over using aforeach
loop. It can be used to accept both arrays and objects that implement theTraversable
interface.
Here are examples of how these special types are used in PHP:
// Example of NULL type
$variable = null; // Variable with no value
if (is_null($variable)) {
echo "The variable is NULL.\n";
} else {
echo "The variable is not NULL.\n";
}
// Example of resource type
$fileHandle = fopen('data.txt', 'r'); // Open a file in read mode
if (is_resource($fileHandle)) {
echo "File handle is a resource.\n";
fclose($fileHandle); // Close the file handle
} else {
echo "File handle is not a resource.\n";
}
// Example of callable type
function sayHello($name) {
echo "Hello, " . $name . "!\n";
}
$functionName = 'sayHello';
if (is_callable($functionName)) {
$functionName("John"); // Call the function dynamically
} else {
echo "Function not callable.\n";
}
// Example of iterable type
$array = [1, 2, 3, 4, 5];
if (is_iterable($array)) {
foreach ($array as $value) {
echo $value . " ";
}
echo "\n";
} else {
echo "Not iterable.\n";
}
Output:
The variable is NULL.
File handle is a resource.
Hello, John!
1 2 3 4 5
These special types play a crucial role in PHP, enabling you to handle different data scenarios and make your code more expressive and robust. Understanding and using these special types appropriately can help you write more efficient and error-free PHP code.