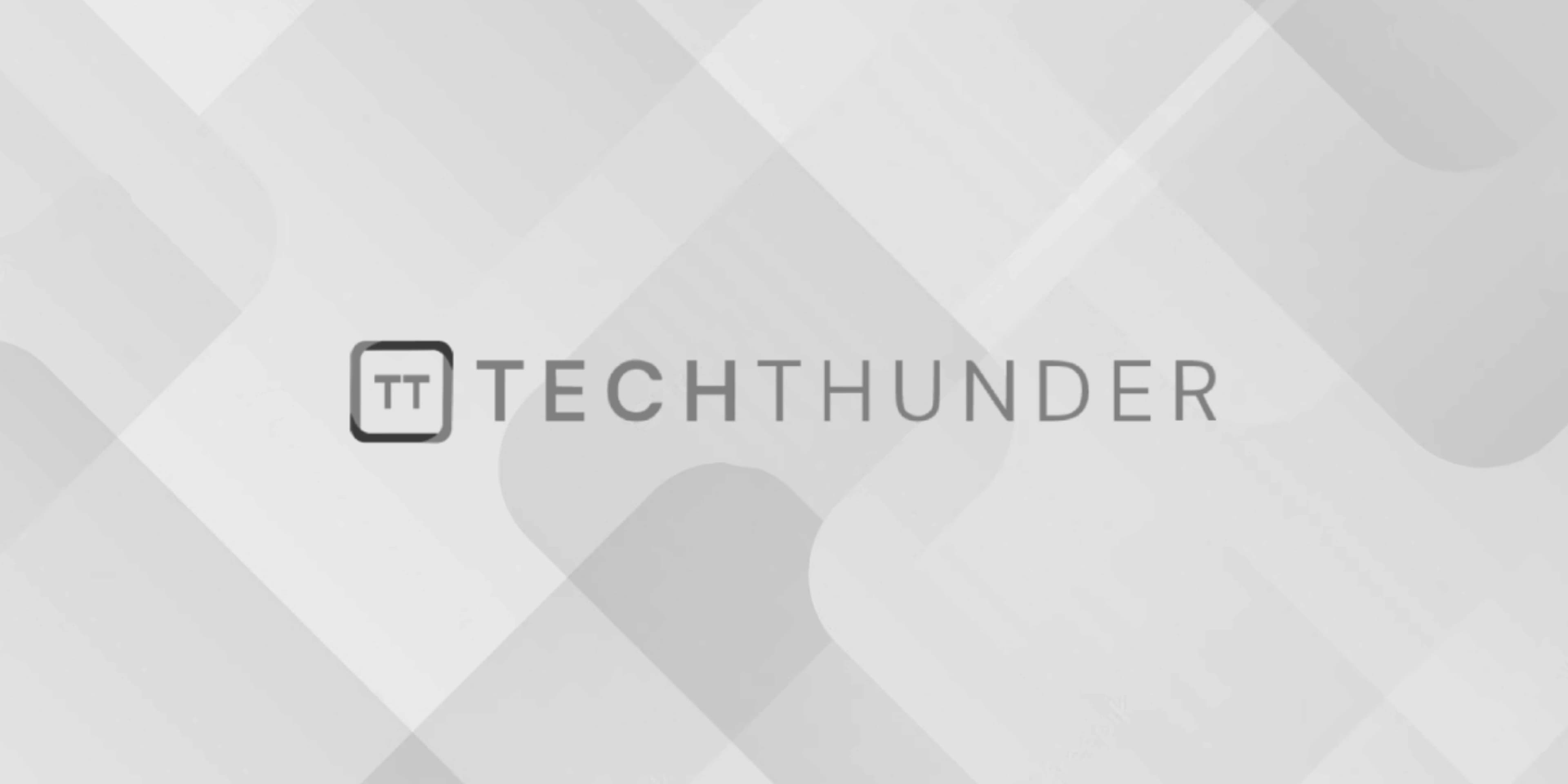
PHP array_push
In PHP, array_push
is a built-in function used to add one or more elements to the end of an array. It is a convenient way to add new elements to an existing array without explicitly specifying the array’s key.
Here’s the syntax of the array_push
function:
array_push(array &$array, mixed $value1 [, mixed $value2 [, mixed $... ]]): int
Parameters:
$array
: The input array to which the elements will be added. This parameter is passed by reference, which means the changes will directly modify the original array.$value1
,$value2
, …: The values to be added to the end of the array.
Return Value:
The function returns the new number of elements in the array after adding the specified values.
Example:
// Initialize an empty array
$fruits = array();
// Add elements to the array using array_push
array_push($fruits, "apple", "banana", "orange");
// Output the modified array
print_r($fruits);
Output:
Array
(
[0] => apple
[1] => banana
[2] => orange
)
In this example, the array_push
function is used to add the elements “apple,” “banana,” and “orange” to the end of the $fruits
array.
It’s worth noting that array_push
can be used with arrays that have both numerical and associative keys. However, if you want to add a single element to an array with a specific key, you can directly assign the value to that key, like this:
// Initialize an empty array
$person = array();
// Add an element to the array with a specific key
$person['name'] = 'John';
$person['age'] = 30;
// Output the modified array
print_r($person);
Output:
Array
(
[name] => John
[age] => 30
)