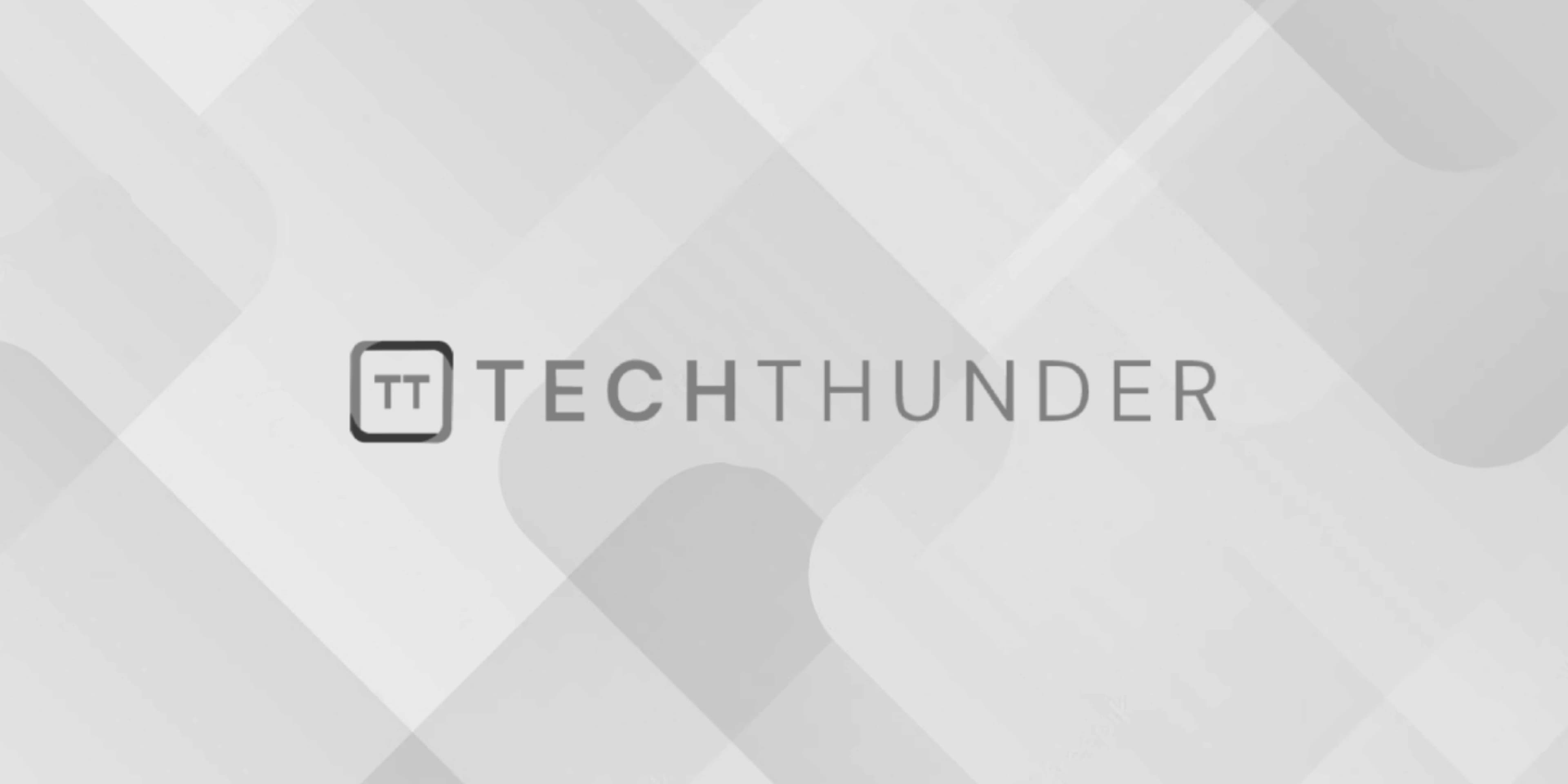
file_put_contents() Function in PHP
In PHP, file_put_contents()
is a built-in function used to write data to a file. It provides a simple and convenient way to create or overwrite the contents of a file with the given data.
Here’s the syntax of the file_put_contents()
function:
int|false file_put_contents(string $filename, mixed $data [, int $flags = 0 [, resource $context ]])
Parameters:
$filename
: The name of the file to write to. If the file does not exist, it will be created. If it already exists, its contents will be overwritten.$data
: The data to write to the file. It can be a string, an array (which will be serialized), or a stream resource.$flags
(optional): Additional flags that modify the behavior of the function. The available flags are:FILE_USE_INCLUDE_PATH
: Search for the file in the include path.FILE_APPEND
: Append the data to the file instead of overwriting it.LOCK_EX
: Acquire an exclusive lock on the file while writing.$context
(optional): A stream context resource created withstream_context_create()
.
Return value:
- If the function is successful, it returns the number of bytes written to the file. If the write operation fails, it returns
false
.
Example:
$file = 'example.txt';
$data = 'Hello, World!';
// Write the data to the file (create or overwrite)
$bytesWritten = file_put_contents($file, $data);
if ($bytesWritten !== false) {
echo "Data written to the file: $bytesWritten bytes\n";
} else {
echo "Error writing to the file\n";
}
In this example, we use file_put_contents()
to write the string “Hello, World!” to the file named “example.txt”. If the file does not exist, it will be created. If it already exists, its contents will be replaced with the new data. The function returns the number of bytes written to the file, and we check if the operation was successful by comparing the return value with false
.
It’s worth noting that if the file cannot be written (due to permission issues or other reasons), file_put_contents()
will return false
. Therefore, it’s a good practice to check the return value for errors when using this function.
Additionally, if you want to append data to an existing file instead of overwriting it, you can use the FILE_APPEND
flag:
$file = 'example.txt';
$data = 'Hello again, World!';
// Append the data to the file
file_put_contents($file, $data, FILE_APPEND);
This will add the string “Hello again, World!” to the end of the file without removing its existing contents.