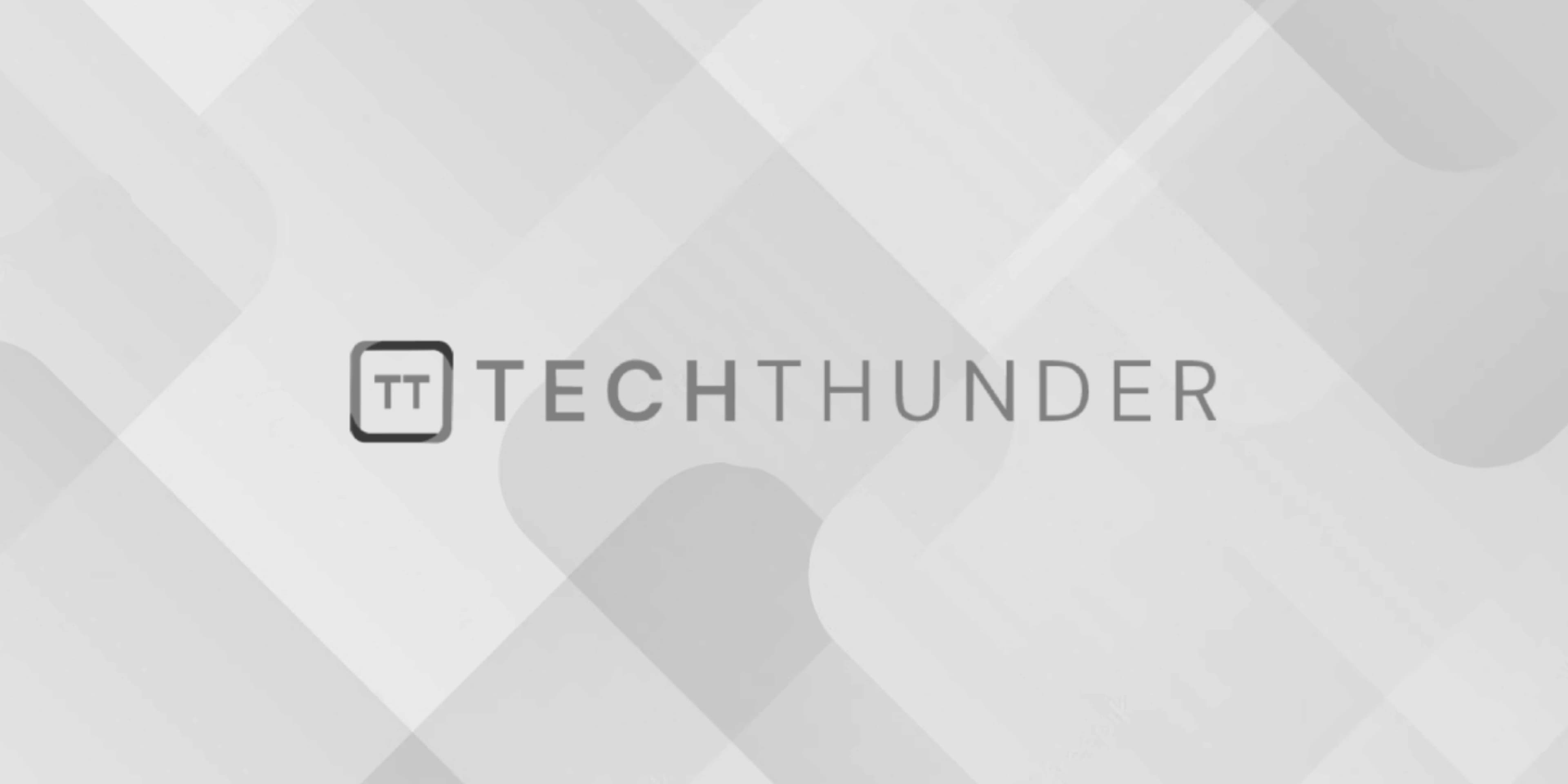
229 views
PHP compare dates
In PHP, you can compare dates using various methods depending on your specific requirements. Here are some common ways to compare dates in PHP:
- Using Comparison Operators:
PHP’s comparison operators (<
,<=
,>
,>=
,==
, and!=
) can be used to compare dates represented as strings in the format ‘YYYY-MM-DD’ or ‘YYYY-MM-DD HH:MM:SS’. These operators will compare the dates based on their chronological order.
Example:
$date1 = '2023-07-15';
$date2 = '2023-07-20';
if ($date1 < $date2) {
echo "Date 1 is earlier than Date 2";
} elseif ($date1 > $date2) {
echo "Date 1 is later than Date 2";
} else {
echo "Date 1 and Date 2 are the same";
}
- Using
strtotime()
Function:
Thestrtotime()
function in PHP can convert date strings into Unix timestamps, which are numeric representations of dates. You can then compare these timestamps using comparison operators.
Example:
$date1 = '2023-07-15';
$date2 = '2023-07-20';
$timestamp1 = strtotime($date1);
$timestamp2 = strtotime($date2);
if ($timestamp1 < $timestamp2) {
echo "Date 1 is earlier than Date 2";
} elseif ($timestamp1 > $timestamp2) {
echo "Date 1 is later than Date 2";
} else {
echo "Date 1 and Date 2 are the same";
}
- Using DateTime Objects:
PHP’sDateTime
class allows you to create date objects and perform various date-related operations, including comparisons.
Example:
$date1 = new DateTime('2023-07-15');
$date2 = new DateTime('2023-07-20');
if ($date1 < $date2) {
echo "Date 1 is earlier than Date 2";
} elseif ($date1 > $date2) {
echo "Date 1 is later than Date 2";
} else {
echo "Date 1 and Date 2 are the same";
}
Using DateTime
objects is often preferred because they provide more flexibility and features for date manipulation.
Keep in mind that when comparing dates, it’s essential to ensure that the date formats are consistent and valid to get accurate results. Using PHP’s built-in date and time functions or the DateTime
class provides reliable ways to work with dates and perform comparisons.