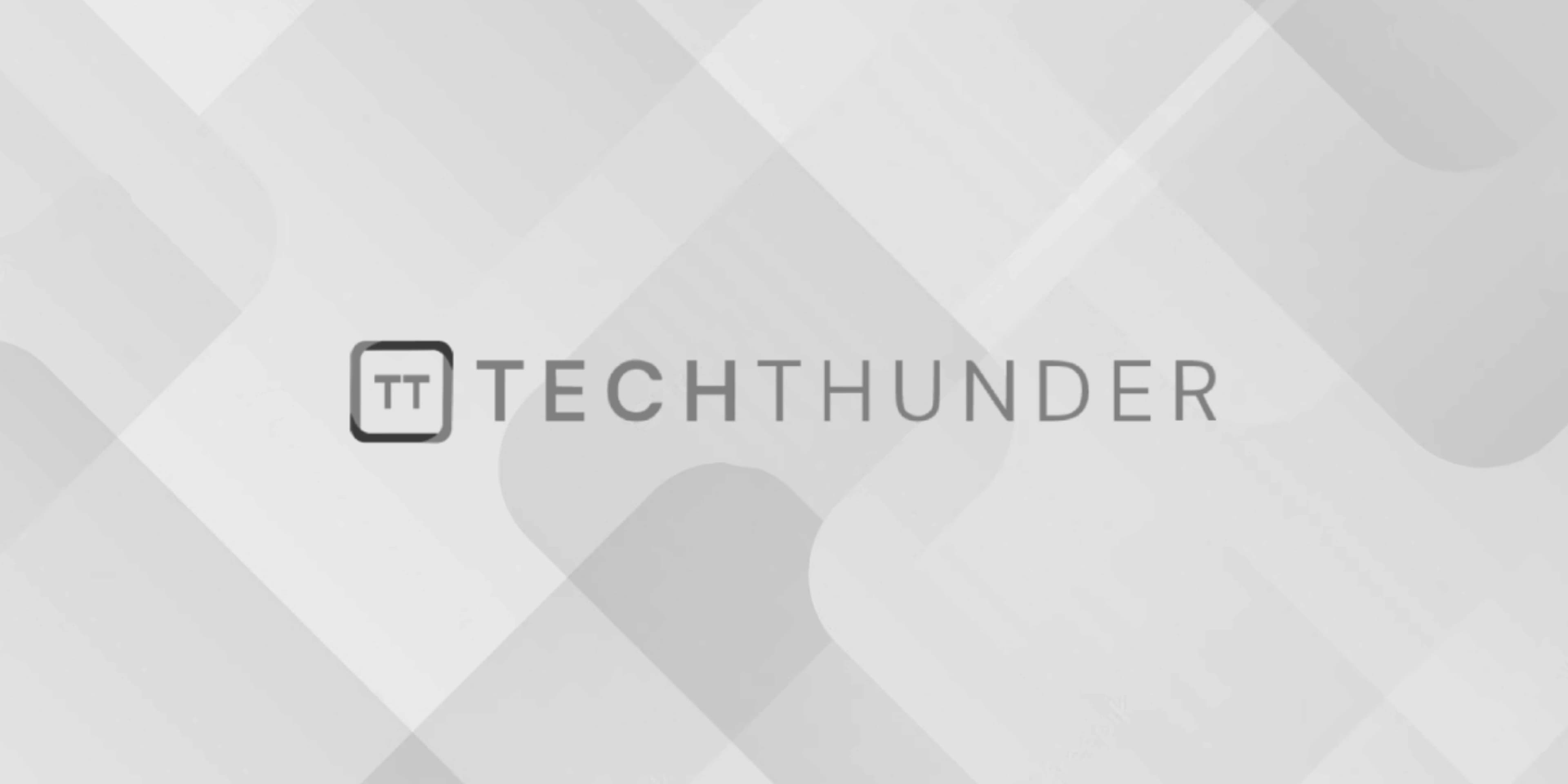
PHP Append File
In PHP, you can use the file_put_contents()
function with the FILE_APPEND
flag to append data to an existing file. The FILE_APPEND
flag ensures that the data is added to the end of the file without overwriting its existing content. Here’s how to use file_put_contents()
to append data to a file:
<?php
$file = 'path/to/your/file.txt'; // Replace this with the actual path and filename of the file you want to append to.
$data = "This is the additional content that will be appended to the file.\n";
$data .= "You can include multiple lines of text using the concatenation operator.\n";
// Append data to the file
if (file_put_contents($file, $data, FILE_APPEND) !== false) {
echo "Data appended to the file successfully.";
} else {
echo "Error appending data to the file.";
}
?>
In this example, replace 'path/to/your/file.txt'
with the actual path and filename of the file you want to append to. The $data
variable contains the content that will be appended to the file. The \n
characters are used to add line breaks between the lines of text.
The file_put_contents()
function, when used with the FILE_APPEND
flag, will open the file in append mode and add the new data to the end of the file without deleting its existing content.
Just like when using file_put_contents()
to write to a file, make sure that the directory where you want to write the file has the appropriate permissions to allow PHP to write to it. Additionally, be cautious when using user input to append to files to prevent security vulnerabilities like path traversal attacks. Always validate and sanitize user input before appending it to a file.