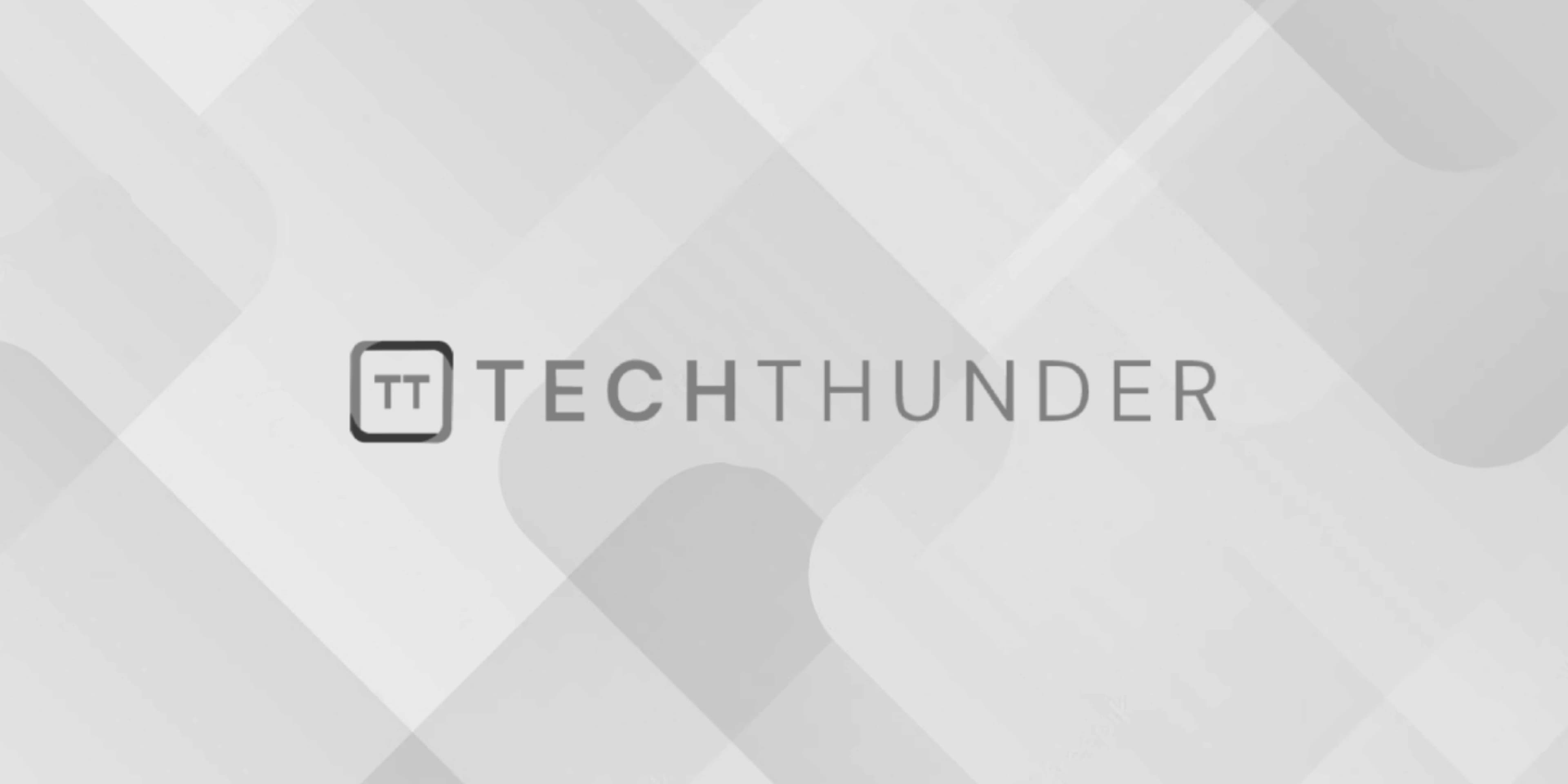
209 views
PHP Codeigniter 3 Create Dynamic Tree View using Bootstrap Treeview JS
To create a dynamic tree view using Bootstrap Treeview JS in CodeIgniter 3, you need to follow these steps:
- Set Up CodeIgniter Project:
Make sure you have CodeIgniter 3 installed and set up on your server. Create a controller, model, and view files for displaying the dynamic tree view. - Install Bootstrap and Bootstrap Treeview:
Download or include Bootstrap and Bootstrap Treeview JS and CSS files in your project. You can use CDNs to include them in your view file. - Controller (TreeController.php):
Create a controller file calledTreeController.php
in theapplication/controllers
folder. This controller will handle the dynamic tree view data and render the view.
<?php
defined('BASEPATH') OR exit('No direct script access allowed');
class TreeController extends CI_Controller {
public function __construct() {
parent::__construct();
$this->load->model('Tree_model');
}
public function index() {
$data['tree_data'] = $this->Tree_model->get_tree_data();
$this->load->view('treeview', $data);
}
}
?>
- Model (Tree_model.php):
Create a model file calledTree_model.php
in theapplication/models
folder. This model will fetch the data required for the dynamic tree view.
<?php
defined('BASEPATH') OR exit('No direct script access allowed');
class Tree_model extends CI_Model {
public function get_tree_data() {
// Fetch your data from the database or any other source and return it as an array
// The array should have a hierarchical structure to represent the tree
// For example, you can have an array like this:
$tree_data = array(
array(
'id' => 1,
'name' => 'Node 1',
'nodes' => array(
array(
'id' => 2,
'name' => 'Subnode 1.1',
'nodes' => array(
array(
'id' => 3,
'name' => 'Subnode 1.1.1',
'nodes' => array()
)
)
),
array(
'id' => 4,
'name' => 'Subnode 1.2',
'nodes' => array()
)
)
),
// Add more nodes as needed...
);
return $tree_data;
}
}
?>
- View (treeview.php):
Create a view file calledtreeview.php
in theapplication/views
folder. This view will render the dynamic tree view using Bootstrap Treeview JS.
<!DOCTYPE html>
<html>
<head>
<title>Dynamic Tree View with Bootstrap Treeview JS</title>
<link href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css" rel="stylesheet">
<link href="https://cdnjs.cloudflare.com/ajax/libs/bootstrap-treeview/1.2.0/bootstrap-treeview.min.css" rel="stylesheet">
</head>
<body>
<div class="container">
<h2>Dynamic Tree View</h2>
<div id="treeview"></div>
</div>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/bootstrap-treeview/1.2.0/bootstrap-treeview.min.js"></script>
<script>
$(document).ready(function() {
var treeData = <?php echo json_encode($tree_data); ?>;
// Initialize Bootstrap Treeview with the data
$('#treeview').treeview({
data: treeData,
showBorder: false,
expandIcon: 'glyphicon glyphicon-plus',
collapseIcon: 'glyphicon glyphicon-minus'
});
});
</script>
</body>
</html>
In this example, we fetch the tree data from the Tree_model
model and pass it to the view. The view then uses Bootstrap Treeview JS to render the dynamic tree view.
Make sure you have Bootstrap and Bootstrap Treeview JS correctly included in your project. Adjust the data fetched from the model to represent the actual hierarchical structure of your tree data.
That’s it! After completing these steps, you should have a dynamic tree view displayed in your CodeIgniter 3 project using Bootstrap Treeview JS.