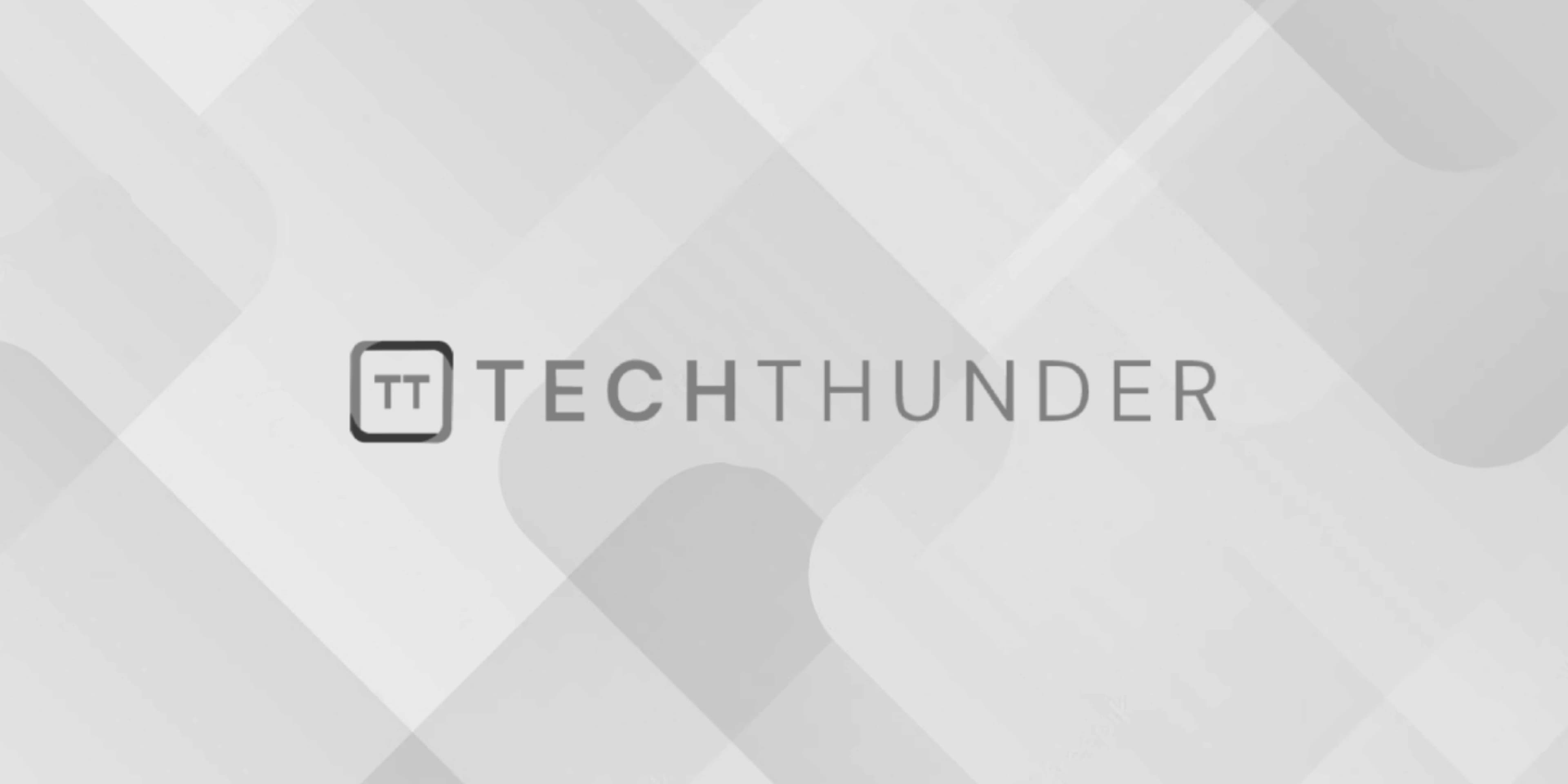
302 views
PHP Read File
In PHP, you can read the contents of a file using various file reading functions. Here are some commonly used methods to read a file:
file_get_contents()
:
Thefile_get_contents()
function reads the entire contents of a file into a string. It is a simple and convenient way to read small files.
Example:
$filename = "example.txt";
$file_contents = file_get_contents($filename);
// Output the file contents
echo $file_contents;
fopen()
andfread()
:
Thefopen()
function is used to open the file and establish a file handle, andfread()
is used to read a specified number of bytes from the file.
Example:
$filename = "example.txt";
$file_handle = fopen($filename, "r");
// Read the first 100 bytes from the file
$file_contents = fread($file_handle, 100);
// Output the read contents
echo $file_contents;
// Close the file handle
fclose($file_handle);
fgets()
:
Thefgets()
function reads a single line from the file.
Example:
$filename = "example.txt";
$file_handle = fopen($filename, "r");
// Read a line from the file
$line = fgets($file_handle);
// Output the line
echo $line;
// Close the file handle
fclose($file_handle);
- Reading Line by Line with
feof()
andfgets()
:
You can use a loop to read the file line by line until the end of the file is reached.
Example:
$filename = "example.txt";
$file_handle = fopen($filename, "r");
while (!feof($file_handle)) {
$line = fgets($file_handle);
echo $line;
}
// Close the file handle
fclose($file_handle);
These are basic examples for reading files in PHP. Remember to handle error cases, such as file not found or permission issues, by using appropriate error handling mechanisms like try-catch blocks or file_exists()
checks before attempting to read the file. Also, always close the file handle after reading to free system resources.