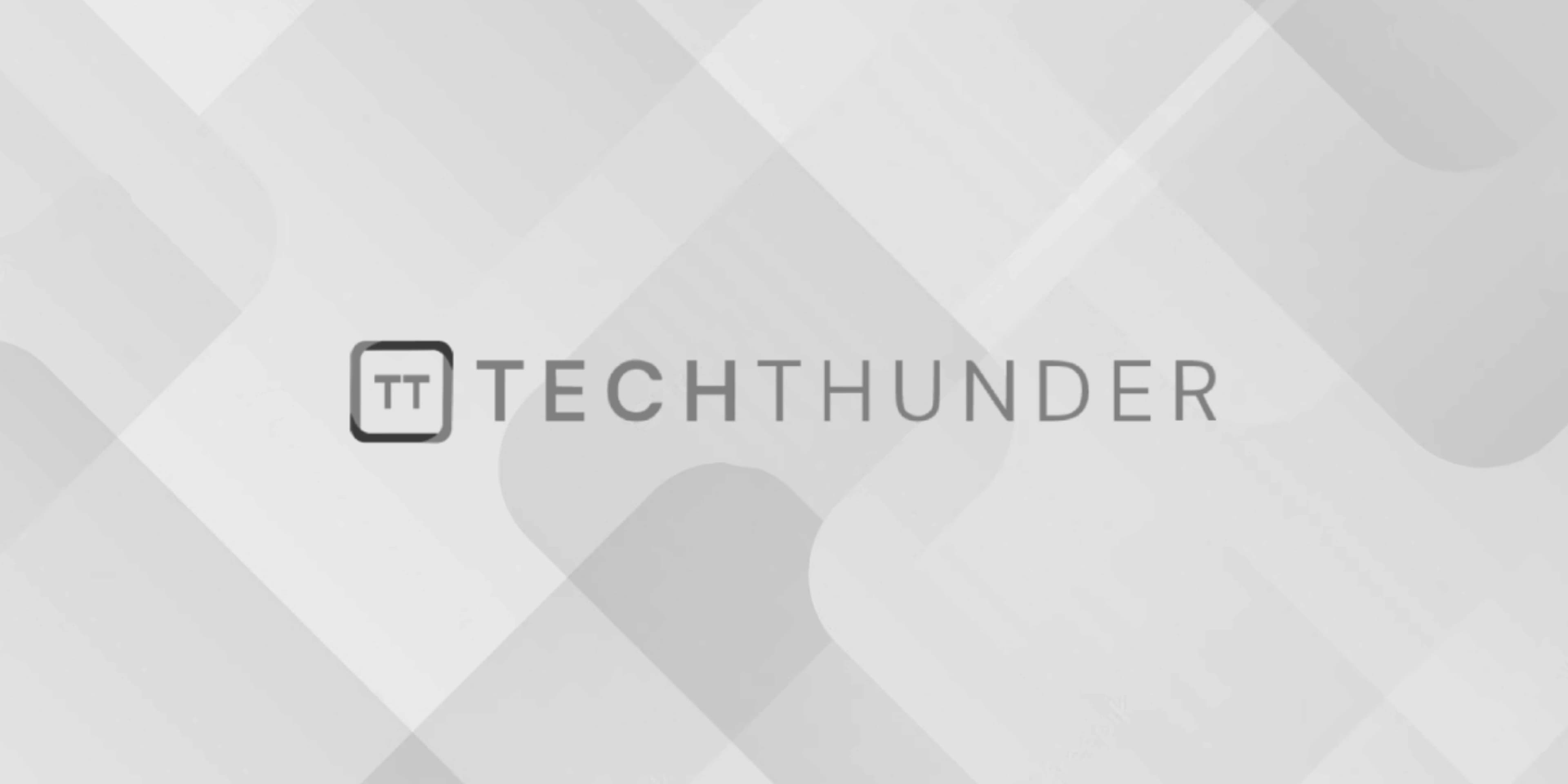
PHP File Upload
In PHP, you can enable file uploads to allow users to upload files to your web server. File uploads are commonly used in web applications for tasks like uploading images, documents, and other files. To implement file upload functionality in PHP, you need to follow these steps:
- Create an HTML form with the appropriate enctype attribute:
<form action="upload.php" method="post" enctype="multipart/form-data">
<input type="file" name="fileToUpload" id="fileToUpload">
<input type="submit" value="Upload File" name="submit">
</form>
- Create a PHP script to handle the file upload (upload.php):
<?php
if ($_SERVER["REQUEST_METHOD"] == "POST" && isset($_FILES["fileToUpload"])) {
$targetDir = "uploads/"; // Directory where the uploaded file will be saved
$targetFile = $targetDir . basename($_FILES["fileToUpload"]["name"]);
$uploadOk = true;
$fileType = strtolower(pathinfo($targetFile, PATHINFO_EXTENSION));
// Check if the file is a valid image (Optional, you can customize this based on your requirements)
$check = getimagesize($_FILES["fileToUpload"]["tmp_name"]);
if ($check !== false) {
echo "File is an image - " . $check["mime"] . ".";
$uploadOk = true;
} else {
echo "File is not an image.";
$uploadOk = false;
}
// Check if file already exists (Optional, you can customize this based on your requirements)
if (file_exists($targetFile)) {
echo "File already exists.";
$uploadOk = false;
}
// Check file size (Optional, you can customize this based on your requirements)
if ($_FILES["fileToUpload"]["size"] > 500000) {
echo "File is too large.";
$uploadOk = false;
}
// Allow certain file formats (Optional, you can customize this based on your requirements)
if ($fileType !== "jpg" && $fileType !== "png" && $fileType !== "jpeg" && $fileType !== "gif") {
echo "Only JPG, JPEG, PNG, and GIF files are allowed.";
$uploadOk = false;
}
if ($uploadOk) {
if (move_uploaded_file($_FILES["fileToUpload"]["tmp_name"], $targetFile)) {
echo "The file " . basename($_FILES["fileToUpload"]["name"]) . " has been uploaded.";
} else {
echo "Error uploading the file.";
}
}
}
?>
In this example, the PHP script checks if the file was submitted via a POST request and if the “fileToUpload” field is set in the POST data. It then performs various checks like file size, file type, and if the file already exists before moving the file to the desired directory using move_uploaded_file()
.
Remember to set appropriate permissions on the directory where you want to store the uploaded files to ensure successful file uploads. Additionally, always validate and sanitize user input to prevent security vulnerabilities like file path manipulation or file injection attacks.