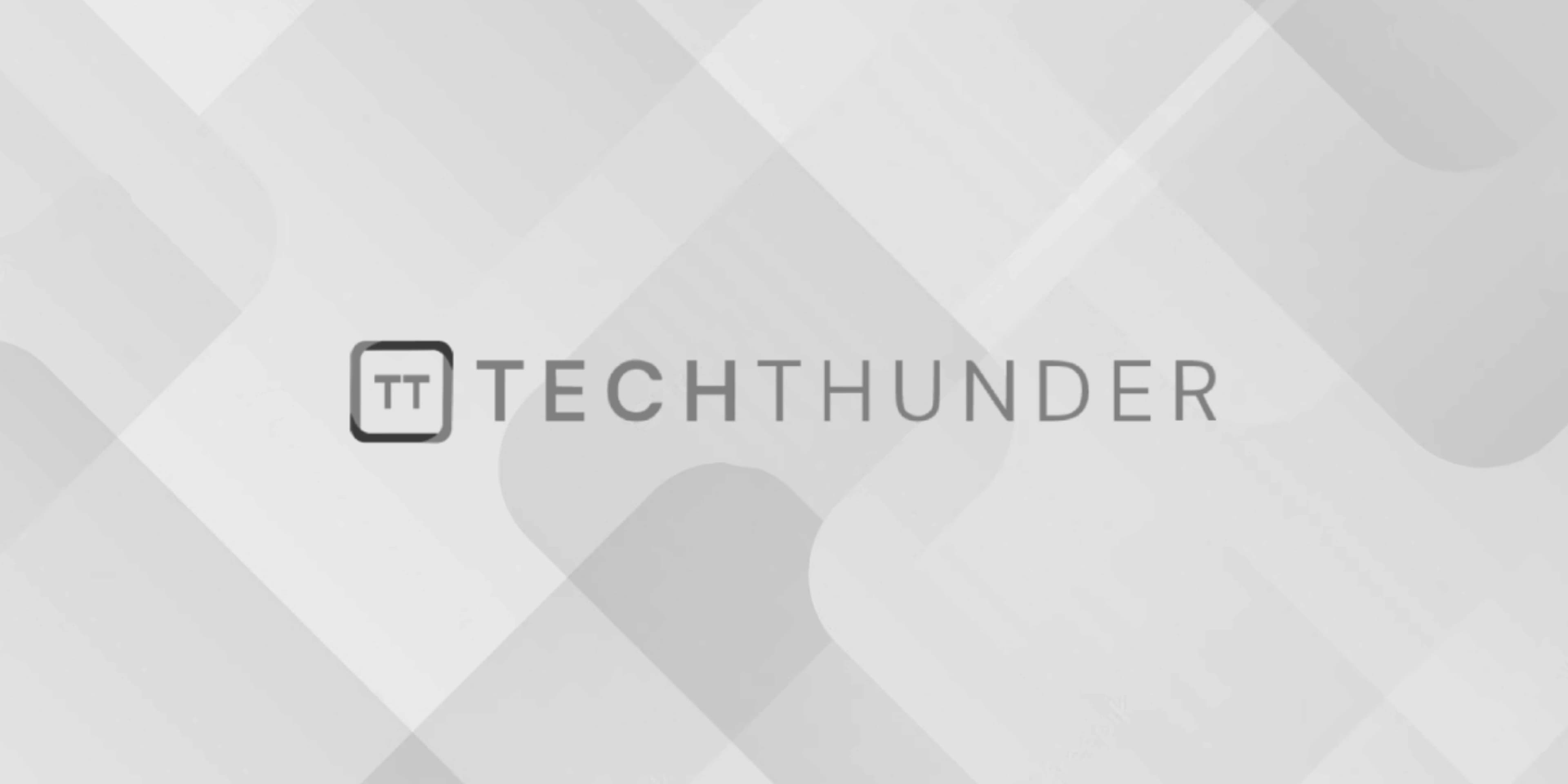
Is_array() Function in PHP
In PHP, is_array()
is a built-in function used to determine whether a given variable is an array or not. It returns true
if the variable is an array and false
if it is not.
Here’s the syntax of the is_array()
function:
bool is_array(mixed $variable)
Parameters:
$variable
: The variable to be checked for array type.
Return value:
- The function returns
true
if the variable is an array, andfalse
if it is not.
Example:
// Examples of different variables
$arrayVariable = [1, 2, 3]; // An array
$stringVariable = "Hello"; // A string
$integerVariable = 42; // An integer
$nullVariable = null; // Null value
// Checking if the variables are arrays
var_dump(is_array($arrayVariable)); // Output: bool(true)
var_dump(is_array($stringVariable)); // Output: bool(false)
var_dump(is_array($integerVariable)); // Output: bool(false)
var_dump(is_array($nullVariable)); // Output: bool(false)
In this example, we have various variables of different types. The is_array()
function is used to check each variable, and the var_dump()
function is used to display the result.
- For
$arrayVariable
, which is an array,is_array()
returnstrue
. - For
$stringVariable
,$integerVariable
, and$nullVariable
, which are not arrays,is_array()
returnsfalse
.
is_array()
is often used in PHP code to verify if a variable is an array before performing array-specific operations, such as iterating over elements, accessing specific keys, or manipulating array data. It helps ensure that the variable is of the expected type before applying array-specific functionality, reducing the risk of errors in the code.