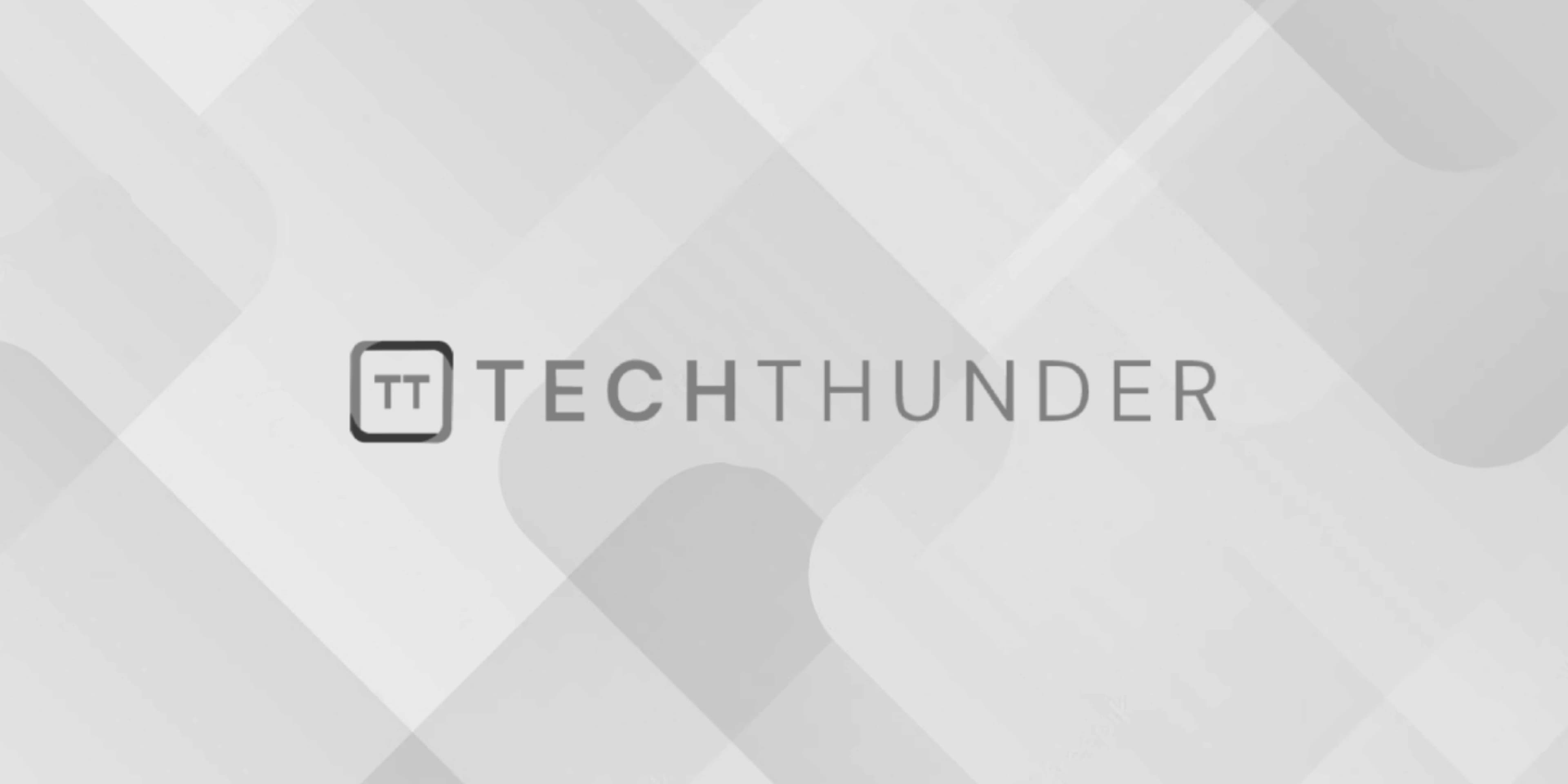
PHP JSON
In PHP, you can work with JSON (JavaScript Object Notation) data to encode PHP data structures into a JSON format or decode JSON data back into PHP data types. JSON is a lightweight data interchange format that is widely used for data exchange between client and server or between different systems.
Here’s how you can work with JSON in PHP:
- Encoding PHP Data to JSON:
To convert a PHP array or object into a JSON string, you can use thejson_encode()
function. This function takes the PHP data as input and returns a JSON-encoded string.
<?php
// PHP array
$data = array(
'name' => 'John Doe',
'age' => 30,
'email' => '[email protected]'
);
// Encode PHP array to JSON
$jsonData = json_encode($data);
// Output the JSON data
echo $jsonData;
?>
- Decoding JSON Data to PHP:
To convert a JSON string back into PHP data types (array or object), you can use thejson_decode()
function. This function takes the JSON string as input and returns a PHP array or object.
<?php
// JSON data
$jsonData = '{"name":"John Doe","age":30,"email":"[email protected]"}';
// Decode JSON data to PHP array
$data = json_decode($jsonData, true);
// Output the PHP array
print_r($data);
?>
In the json_decode()
function, the second parameter is optional and determines whether the returned data will be an associative array (if set to true
) or a PHP object (if set to false
or omitted). If you don’t pass the second parameter, it returns a PHP object by default.
- Working with JSON in API Endpoints:
JSON is commonly used for communication between client-side and server-side applications or when building API endpoints. For example, you can send JSON data as a response from a PHP API endpoint usingjson_encode()
:
<?php
// Assume this data comes from a database query or other source
$data = array(
'name' => 'John Doe',
'age' => 30,
'email' => '[email protected]'
);
// Set the response content type to JSON
header('Content-Type: application/json');
// Encode PHP array to JSON and send as the response
echo json_encode($data);
?>
When working with API endpoints, it’s common to receive JSON data from client applications and then decode it using json_decode()
to process the data on the server side.
Remember to handle JSON data carefully, especially if it comes from external sources, to prevent security vulnerabilities like JSON hijacking or other attacks. Validate and sanitize user input before encoding it to JSON or decoding JSON data in your PHP application.