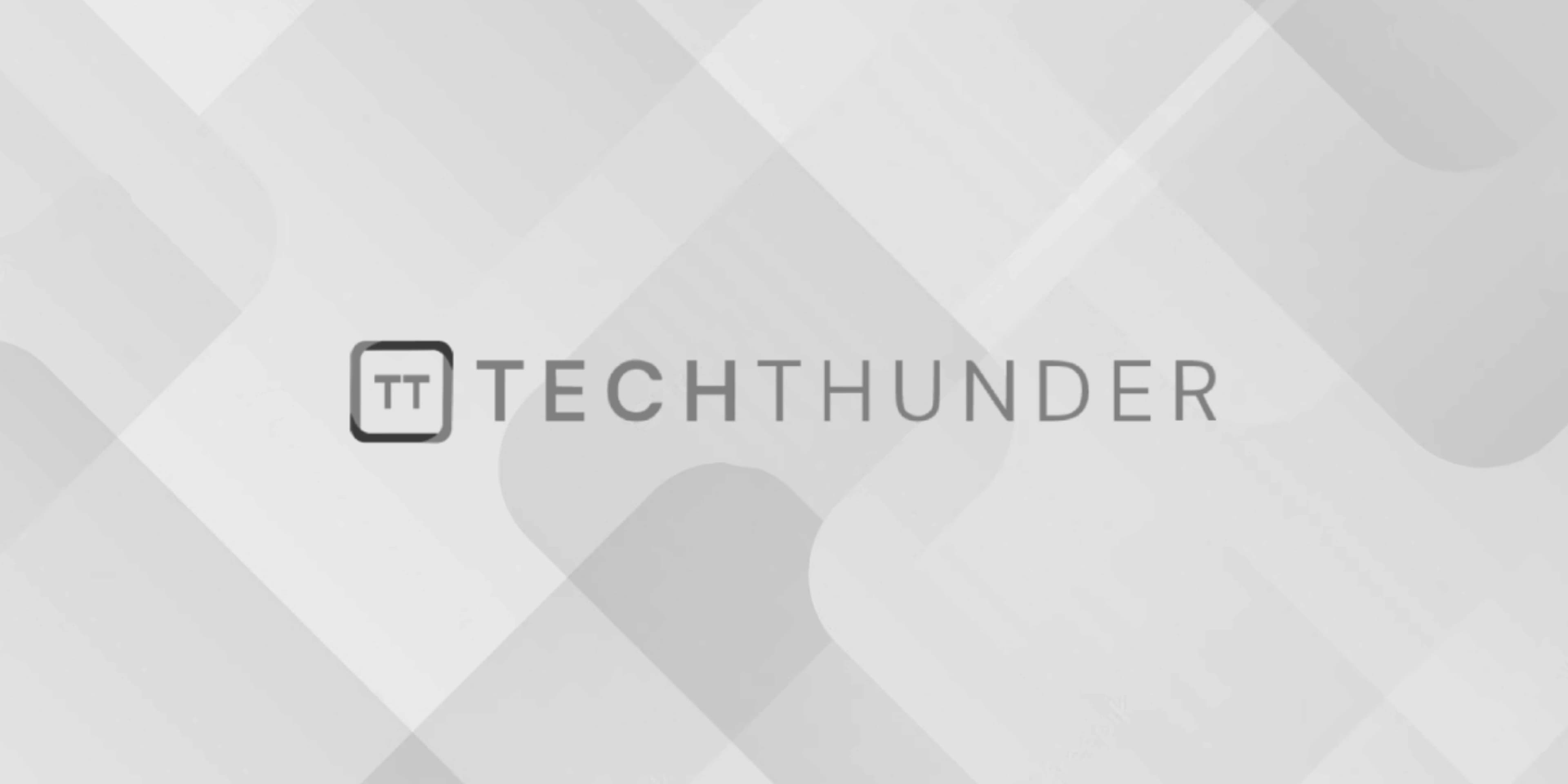
232 views
PHP Data Types
In PHP, data types are used to define the type of data that a variable or expression can hold. PHP is a loosely typed language, which means that variables do not need to have their data types explicitly declared. Instead, PHP determines the data type automatically based on the value assigned to the variable. PHP supports several fundamental data types, which can be categorized into the following categories:
- Scalar Data Types:
- Integer: Represents whole numbers, such as 1, 100, -500, etc.
- Float (or Double): Represents numbers with decimal points, such as 3.14, -0.5, 1.0, etc.
- String: Represents sequences of characters, such as “Hello, World!”, ‘PHP’, etc.
- Boolean: Represents true or false values, denoted by
true
orfalse
.
- Compound Data Types:
- Array: Represents an ordered collection of elements, where each element can have its own data type.
- Object: Represents instances of classes, and each object can have its own properties and methods.
- Callable: Represents a callback or callable function.
- Special Data Types:
- NULL: Represents a variable with no value or an explicitly assigned NULL value.
- Resource: Represents external resources such as file handles or database connections.
Here are some examples of PHP data types:
// Scalar Data Types
$integerVar = 42;
$floatVar = 3.14;
$stringVar = "Hello, World!";
$booleanVar = true;
// Array
$fruits = array("Apple", "Banana", "Orange");
// Object
class Person {
public $name;
public $age;
}
$personObj = new Person();
$personObj->name = "John";
$personObj->age = 30;
// NULL
$nullVar = null;
// Resource
$fileHandle = fopen("example.txt", "r");
You can use the gettype()
function to determine the data type of a variable:
echo gettype($integerVar); // Output: integer
echo gettype($floatVar); // Output: double
echo gettype($stringVar); // Output: string
echo gettype($booleanVar); // Output: boolean
echo gettype($fruits); // Output: array
echo gettype($personObj); // Output: object
echo gettype($nullVar); // Output: NULL
echo gettype($fileHandle); // Output: resource
Understanding data types in PHP is essential for writing robust and efficient code. It helps you handle data correctly and avoid unexpected results or errors in your PHP applications.