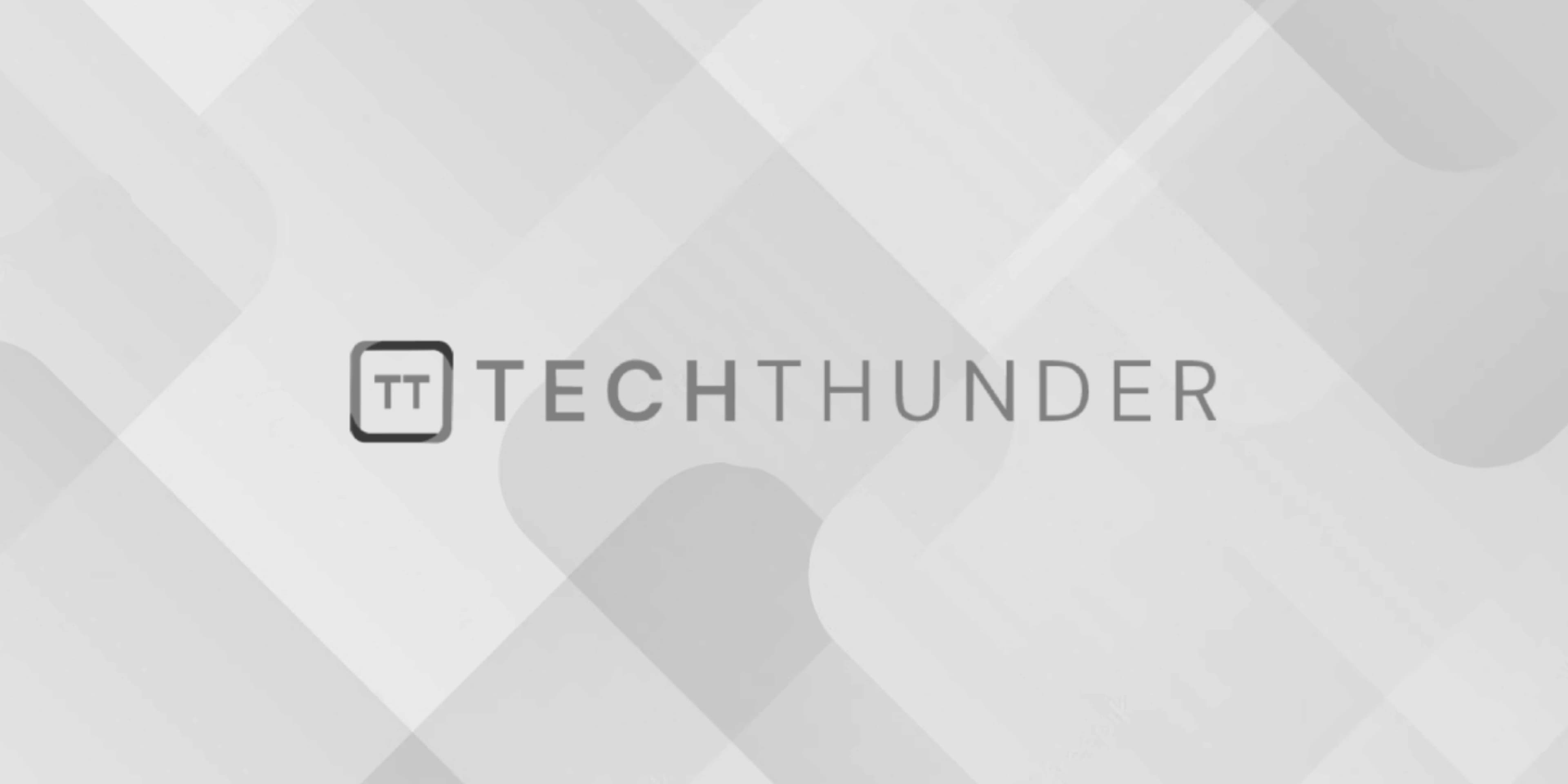
PHP Download File
In PHP, you can use the readfile()
function to download a file to the user’s browser. The readfile()
function reads a file and sends its contents to the output buffer, allowing the user to download the file. Here’s a simple example of how to use readfile()
to initiate a file download:
Assuming you have a file named “example.pdf” that you want to download:
<?php
$filePath = 'path/to/your/example.pdf'; // Replace this with the actual path to your file.
if (file_exists($filePath)) {
// Set the appropriate headers to initiate the download.
header('Content-Description: File Transfer');
header('Content-Type: application/octet-stream');
header('Content-Disposition: attachment; filename="'.basename($filePath).'"');
header('Expires: 0');
header('Cache-Control: must-revalidate');
header('Pragma: public');
header('Content-Length: ' . filesize($filePath));
// Read the file and send it to the user's browser.
readfile($filePath);
exit;
} else {
// File not found, handle the error.
echo "File not found.";
}
?>
In this example, the PHP script first checks if the file exists using file_exists()
to avoid attempting to download a non-existent file. If the file exists, it sets the appropriate HTTP headers to trigger the file download. The Content-Disposition
header tells the browser that the file should be treated as an attachment and gives it a suggested filename.
The readfile()
function then reads the contents of the file and sends them to the user’s browser, initiating the download. The script uses exit;
after readfile()
to ensure that no other output is sent to the browser after the download.
Make sure to replace 'path/to/your/example.pdf'
with the actual path to the file you want to download. Also, consider adding additional security measures, such as restricting access to the file, if required.