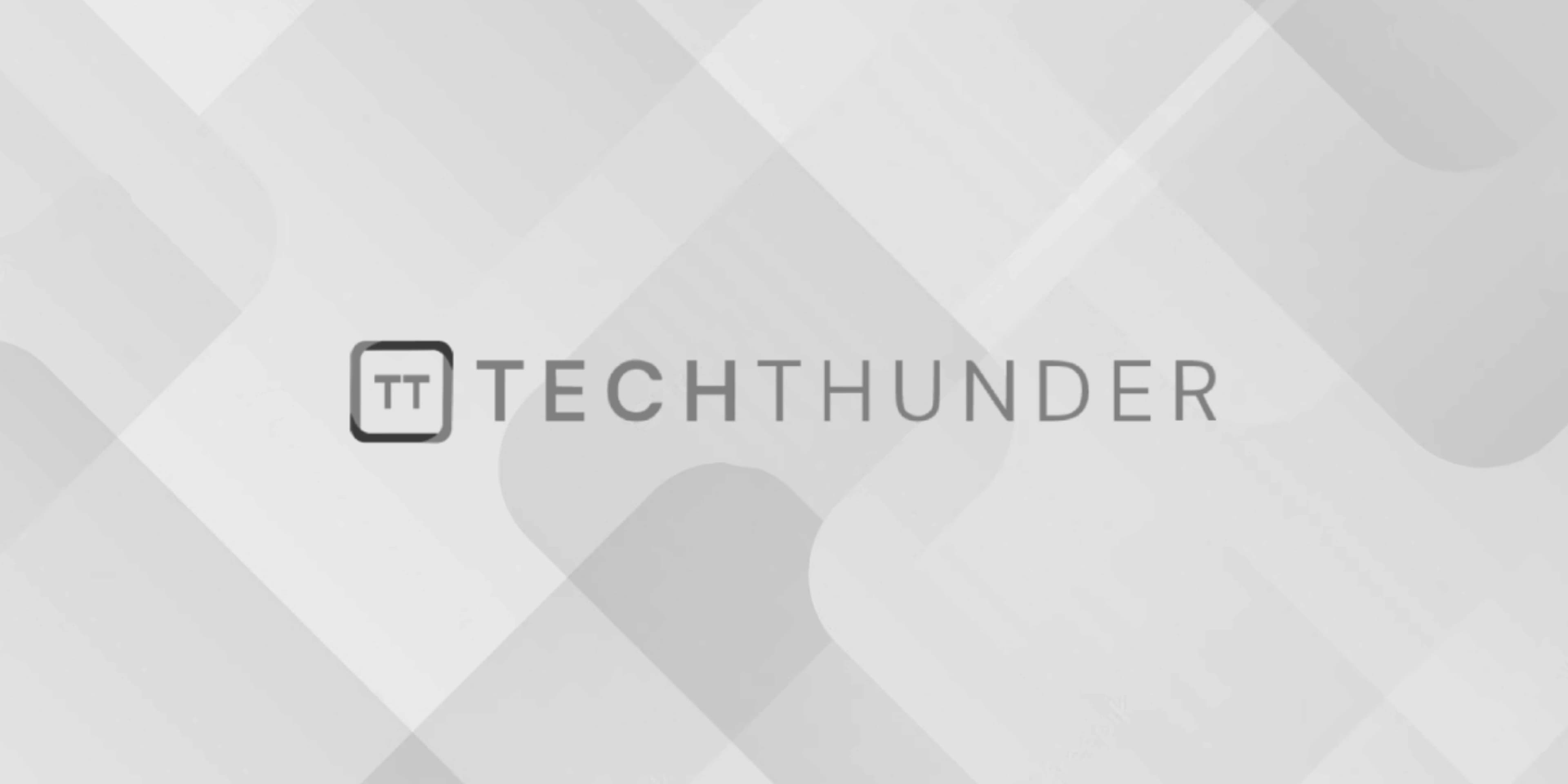
PHP Variable Scope
In PHP, variable scope refers to the context or part of the program where a variable is accessible and can be used. The scope determines the visibility and lifetime of a variable, which means where the variable can be accessed and how long it exists in memory during the execution of the script.
PHP supports three main types of variable scope:
- Global Scope:
- Variables declared outside of any function or class have global scope.
- Global variables can be accessed from any part of the script, including inside functions and classes.
- However, to modify the value of a global variable inside a function, you need to use the
global
keyword to indicate that the variable is from the global scope.
Example of a global variable:
$globalVar = 10;
function incrementGlobal() {
global $globalVar;
$globalVar++;
}
incrementGlobal();
echo $globalVar; // Output: 11
- Local Scope:
- Variables declared inside a function have local scope.
- Local variables can only be accessed and used within the function where they are declared.
- Local variables are created when the function is called and destroyed when the function completes execution, making them temporary and local to the function.
Example of a local variable:
function showMessage() {
$message = "Hello, World!";
echo $message;
}
showMessage(); // Output: Hello, World!
echo $message; // Error: $message is not defined outside the function
- Static Scope:
- A variable declared as
static
inside a function retains its value between function calls. - The variable persists in memory even after the function finishes executing, unlike regular local variables that get destroyed after each function call.
- Static variables are useful when you need to maintain state across multiple calls to the same function.
Example of a static variable:
function countCalls() {
static $count = 0;
$count++;
echo "Call count: " . $count . "<br>";
}
countCalls(); // Output: Call count: 1
countCalls(); // Output: Call count: 2
countCalls(); // Output: Call count: 3
In this example, the static variable $count
retains its value across multiple calls to the countCalls
function, and its value is incremented on each call.
Understanding variable scope is important for writing clean and well-organized code. Properly managing variable scope helps avoid conflicts, unintended changes, and memory issues in your PHP applications.