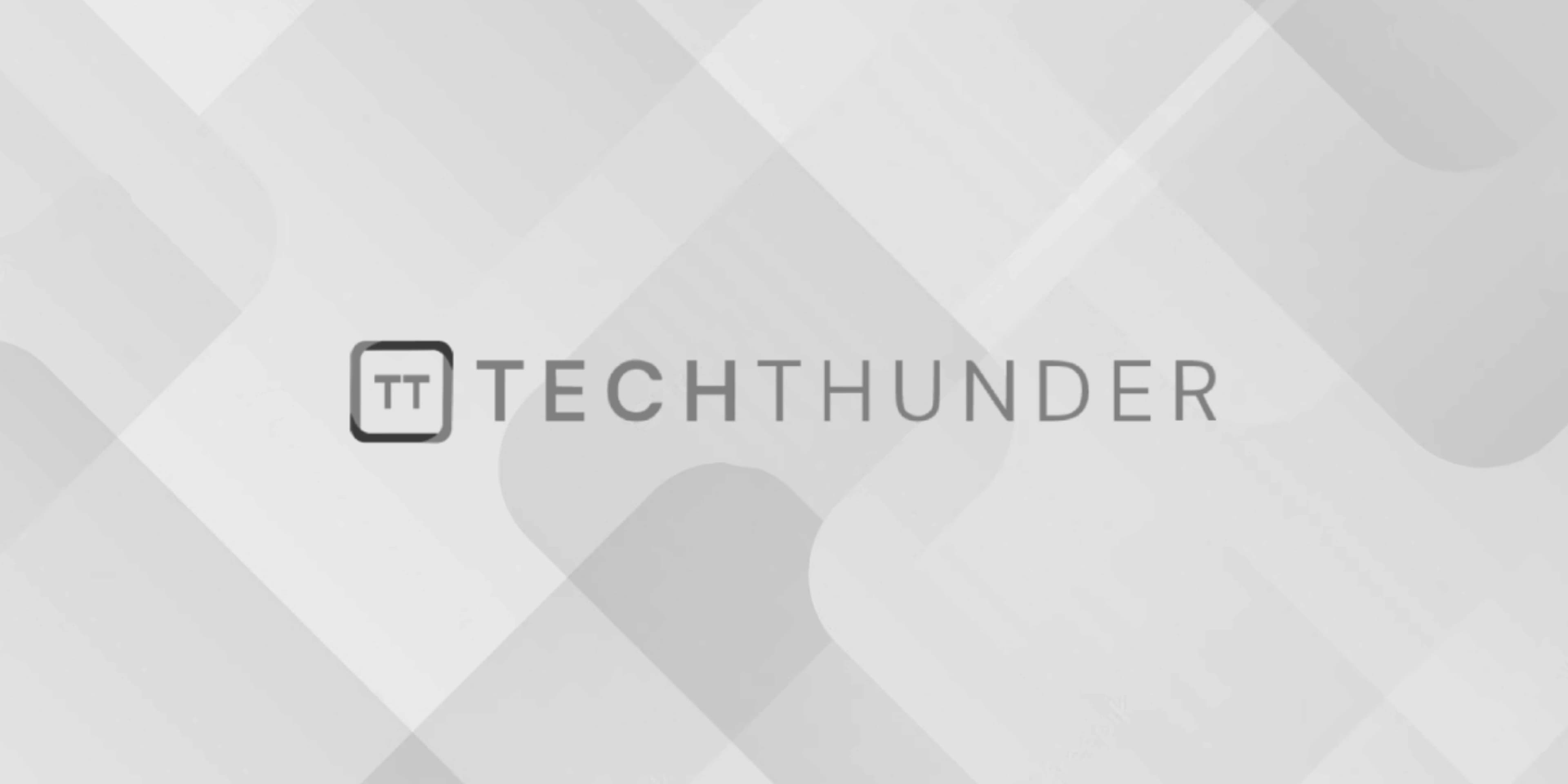
PHP Open File
In PHP, you can open a file using the fopen()
function. The fopen()
function is used to establish a file handle, which allows you to read, write, and manipulate the file. It takes two main parameters: the filename and the mode in which you want to open the file.
Here’s the syntax of the fopen()
function:
resource fopen(string $filename, string $mode, bool $use_include_path = false, resource $context = ?): resource|false
$filename
: The filename of the file you want to open. This can be a relative or absolute path to the file.$mode
: The mode in which you want to open the file. It specifies whether you want to read, write, append, or create the file.$use_include_path
(optional): If set totrue
, PHP will also search for the file in the include path.$context
(optional): A context resource created withstream_context_create()
, which can be used to set additional options for the stream.
The $mode
parameter can take various values depending on your requirements:
"r"
: Read mode. The file is opened for reading only. The file pointer is placed at the beginning of the file."w"
: Write mode. The file is opened for writing only. If the file does not exist, it is created. If it exists, its contents are truncated (cleared)."a"
: Append mode. The file is opened for writing only. If the file does not exist, it is created. The file pointer is placed at the end of the file, allowing you to append content."x"
: Exclusive creation mode. The file is opened for writing only. If the file already exists, thefopen()
call fails."r+"
: Read/write mode. The file is opened for both reading and writing. The file pointer is placed at the beginning of the file."w+"
: Read/write mode. The file is opened for both reading and writing. If the file does not exist, it is created. If it exists, its contents are truncated (cleared)."a+"
: Read/write mode. The file is opened for both reading and writing. If the file does not exist, it is created. The file pointer is placed at the end of the file, allowing you to read and append content.
Example (Opening a file for reading):
$filename = "example.txt";
$file_handle = fopen($filename, "r");
Example (Opening a file for writing):
$filename = "example.txt";
$file_handle = fopen($filename, "w");
Remember to always close the file handle using fclose()
after you are done working with the file to release system resources.
fclose($file_handle);
It’s essential to handle file operations carefully, validate user inputs, and sanitize file paths to prevent security vulnerabilities and ensure proper file handling in your PHP applications.