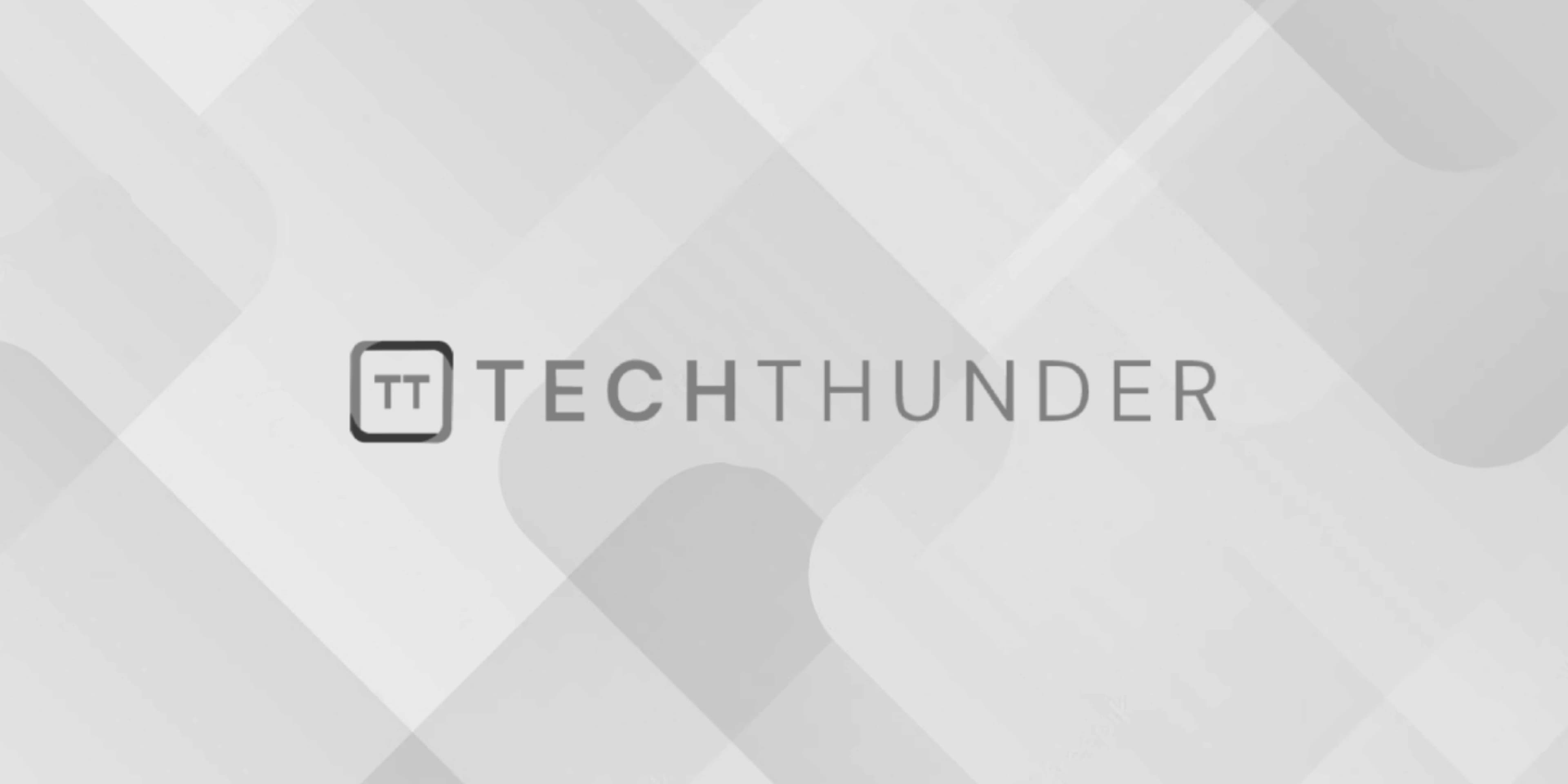
PHP Form: Get Post
In PHP, you can access form data submitted using the HTTP POST method by accessing the $_POST
superglobal array. When a form is submitted with the POST method, the form data is sent in the request body, and PHP automatically populates the $_POST
array with the submitted data. Here’s how you can retrieve form data using the POST method:
HTML Form (form.html):
<!DOCTYPE html>
<html>
<head>
<title>Sample Form</title>
</head>
<body>
<form action="process_form.php" method="post">
<label for="name">Name:</label>
<input type="text" name="name" id="name" required>
<label for="email">Email:</label>
<input type="email" name="email" id="email" required>
<input type="submit" value="Submit">
</form>
</body>
</html>
In the above HTML form, we have two input fields for the user’s name and email, and the form is set to submit the data to the “process_form.php” script using the POST method.
PHP Script (process_form.php):
<?php
if ($_SERVER["REQUEST_METHOD"] == "POST") {
// Retrieve form data using $_POST superglobal
$name = $_POST["name"];
$email = $_POST["email"];
// Do something with the form data, e.g., save it to a database, send an email, etc.
// Display the submitted data
echo "Name: " . $name . "<br>";
echo "Email: " . $email;
}
?>
In the PHP script, we check if the request method is POST ($_SERVER["REQUEST_METHOD"] == "POST"
). If it is, we retrieve the form data from the $_POST
superglobal array using the name
attribute of the form input fields as keys. In this example, the $_POST
array will contain the user’s name and email as submitted through the form.
You can then process the form data as needed, such as saving it to a database or sending an email. In this example, we simply echo the submitted data back to the user as a demonstration.
Remember to sanitize and validate user input before using it in your application to prevent security issues like SQL injection or cross-site scripting (XSS) attacks.