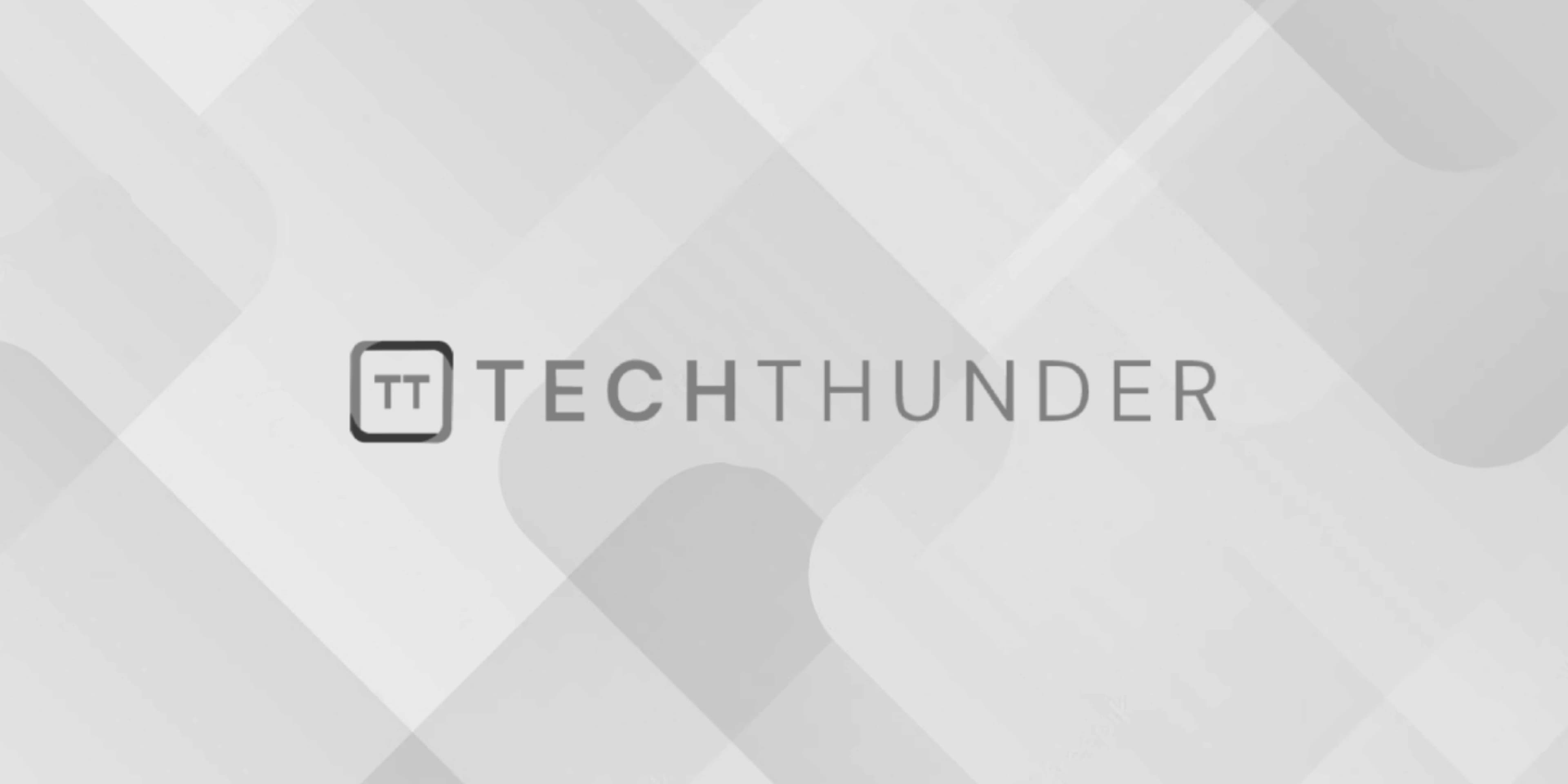
177 views
PHP String Functions
PHP provides a variety of built-in string functions that allow you to manipulate and process strings. Here are some commonly used PHP string functions:
strlen()
: Returns the length of a string.
$string = "Hello, world!";
echo strlen($string); // Output: 13
strpos()
: Finds the position of the first occurrence of a substring in a string.
$string = "Hello, world!";
$position = strpos($string, "world");
echo $position; // Output: 7 (index where "world" starts in the string)
substr()
: Extracts a substring from a string.
$string = "Hello, world!";
$substring = substr($string, 0, 5);
echo $substring; // Output: Hello
str_replace()
: Replaces all occurrences of a substring with another substring in a string.
$string = "Hello, world!";
$newString = str_replace("world", "everyone", $string);
echo $newString; // Output: Hello, everyone!
strtoupper()
: Converts a string to uppercase.
$string = "Hello, world!";
$uppercase = strtoupper($string);
echo $uppercase; // Output: HELLO, WORLD!
strtolower()
: Converts a string to lowercase.
$string = "Hello, world!";
$lowercase = strtolower($string);
echo $lowercase; // Output: hello, world!
ucfirst()
: Converts the first character of a string to uppercase.
$string = "hello, world!";
$ucfirst = ucfirst($string);
echo $ucfirst; // Output: Hello, world!
ucwords()
: Converts the first character of each word in a string to uppercase.
$string = "hello, world!";
$ucwords = ucwords($string);
echo $ucwords; // Output: Hello, World!
trim()
: Removes whitespace or other specified characters from the beginning and end of a string.
$string = " Hello, world! ";
$trimmedString = trim($string);
echo $trimmedString; // Output: "Hello, world!"
explode()
: Splits a string into an array by a specified delimiter.
$string = "apple,banana,orange";
$fruits = explode(",", $string);
print_r($fruits); // Output: Array ( [0] => apple [1] => banana [2] => orange )
These are just a few examples of the many useful string functions available in PHP. There are many more functions that can help you perform various string manipulations and text processing tasks. Always refer to the official PHP documentation for a complete list of string functions and their descriptions.