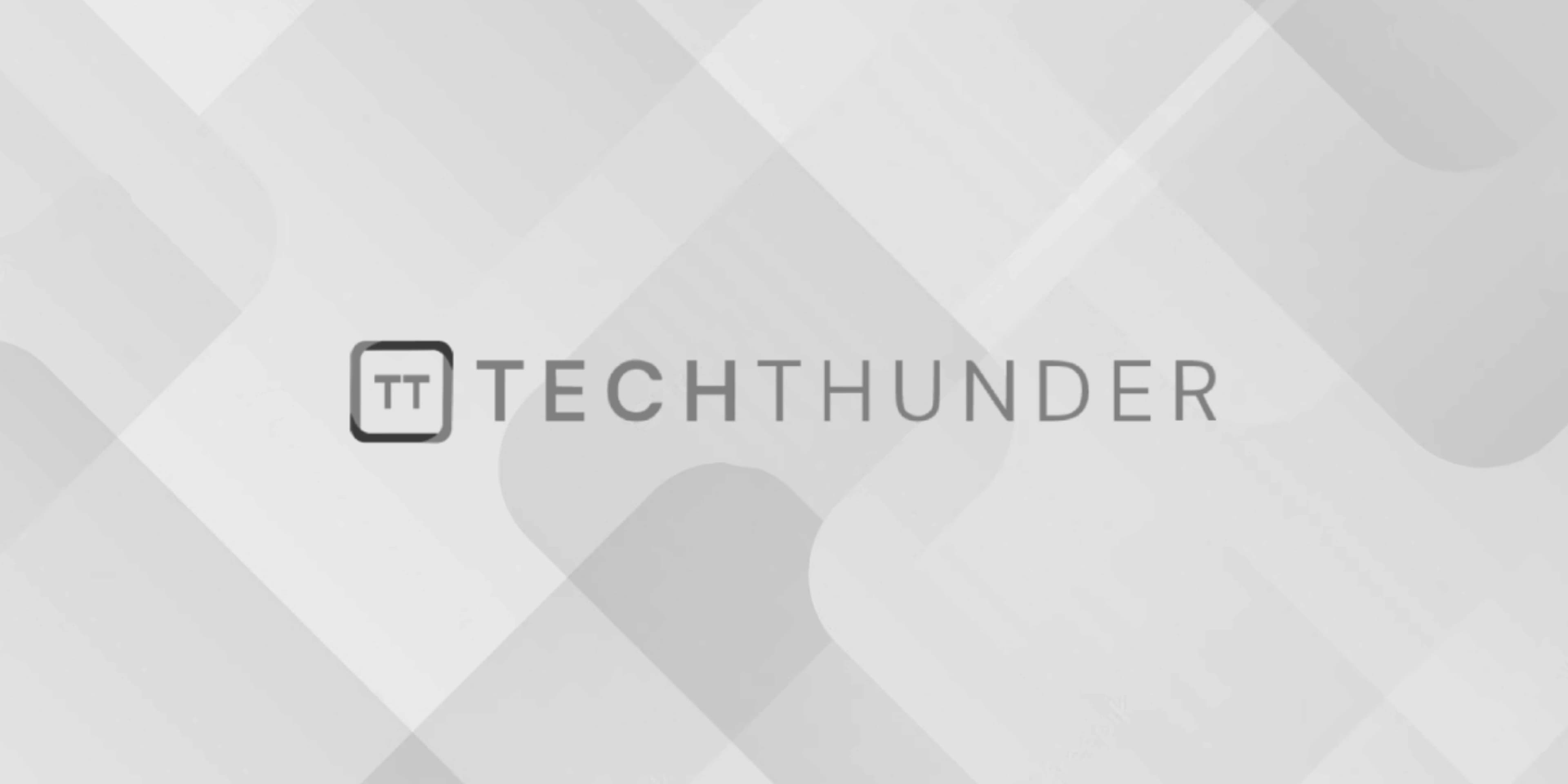
PHP Call By Reference
In PHP, function parameters are typically passed by value, which means that a copy of the variable’s value is passed to the function. However, you can also pass variables by reference, which allows the function to directly modify the original variable rather than a copy of it. This is known as “call by reference.”
To pass a variable by reference in PHP, you need to use the ampersand (&
) symbol before the variable name in both the function declaration and the function call.
Here’s an example to illustrate call by reference:
function multiplyByTwo(&$num) {
$num *= 2;
}
$number = 10;
multiplyByTwo($number);
echo $number; // Output: 20
In the example above, we define a function multiplyByTwo
that takes a parameter $num
by reference (indicated by &$num
). Inside the function, we multiply the value of $num
by 2. When we call the function multiplyByTwo($number)
, we pass the variable $number
by reference using the &
symbol. As a result, the function modifies the original value of $number
directly.
Important points to remember when using call by reference:
- The function definition must include the
&
symbol before the parameter name to indicate that it is passed by reference. - The variable being passed by reference must be a valid variable (i.e., it should already exist).
- Call by reference is usually used when you want to modify the original value of a variable inside a function and have the changes reflected outside the function.
Keep in mind that call by reference can lead to unexpected behavior and make your code harder to maintain. Use it judiciously and only when necessary, as it can make the program logic less clear and introduce unintended side effects.