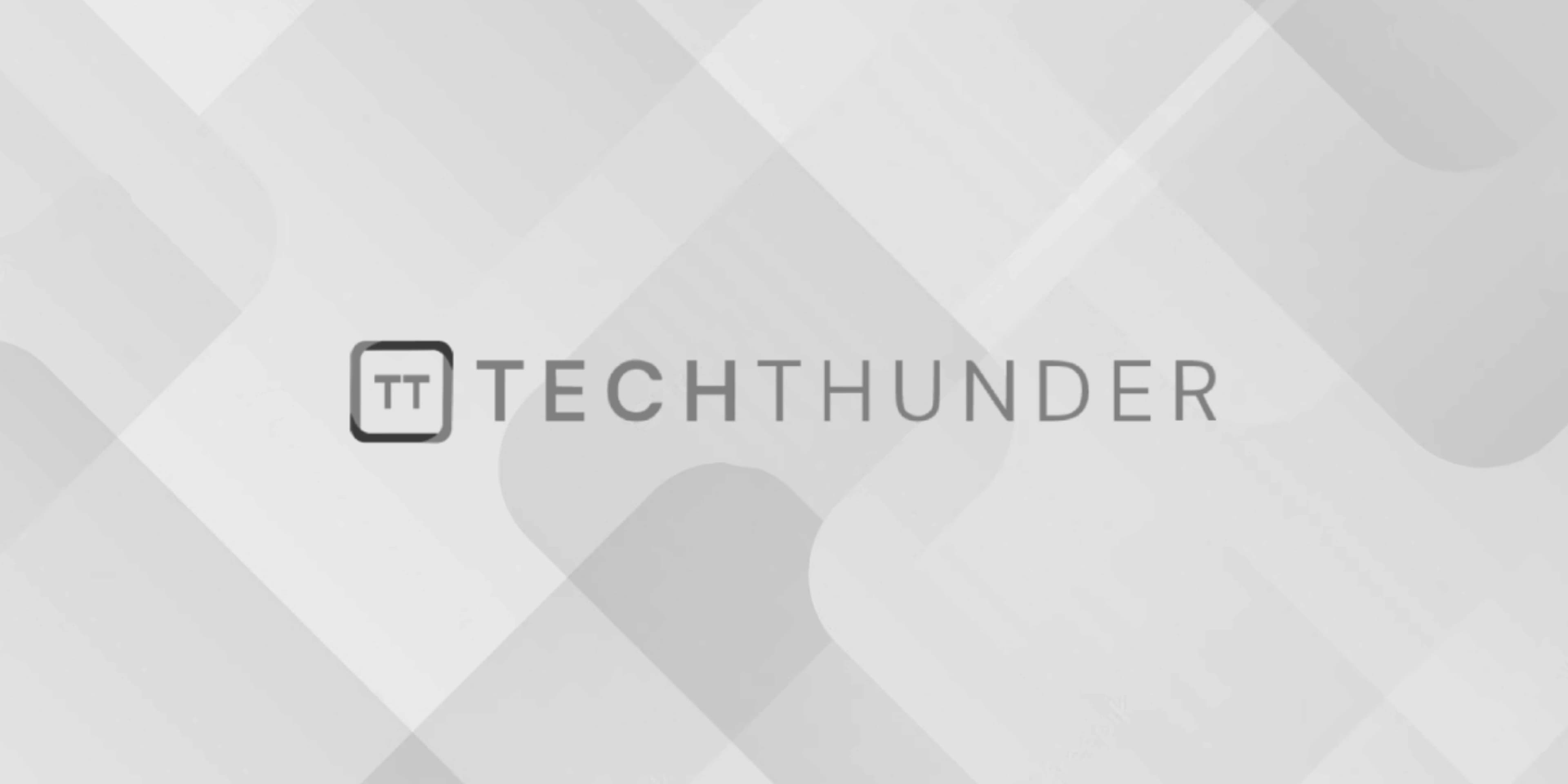
PHP Pagination
Pagination in PHP is a technique used to divide large sets of data into smaller, more manageable chunks or pages. It allows users to navigate through the data efficiently, reducing the load on the server and improving the user experience. Typically, pagination is used when displaying search results, lists, or tables with a large number of records.
Here’s a step-by-step guide on how to implement pagination in PHP:
- Retrieve Data from the Database:
First, you need to retrieve the data that you want to paginate from a database or any data source. You can use SQL queries (e.g., SELECT) with LIMIT and OFFSET clauses to fetch specific chunks of data. - Calculate Pagination Parameters:
Calculate the total number of records and the number of records per page. Also, determine the current page number based on user input or the URL parameter.
// Assuming you have retrieved the data from the database and stored it in the $data array
$totalRecords = count($data);
$recordsPerPage = 10; // Number of records to display on each page
$totalPages = ceil($totalRecords / $recordsPerPage);
// Get the current page number from the URL parameter
$currentPage = isset($_GET['page']) ? $_GET['page'] : 1;
// Validate the current page number to prevent invalid values
if ($currentPage < 1) {
$currentPage = 1;
} elseif ($currentPage > $totalPages) {
$currentPage = $totalPages;
}
// Calculate the starting index for the data slice
$startIndex = ($currentPage - 1) * $recordsPerPage;
// Get the subset of data for the current page
$dataSubset = array_slice($data, $startIndex, $recordsPerPage);
- Display Paginated Data:
Display the data subset ($dataSubset
) on the current page. Additionally, generate links or buttons for pagination navigation to allow users to navigate through different pages.
// Display the data on the current page
foreach ($dataSubset as $item) {
echo $item['name'] . '<br>';
}
// Generate pagination links
echo '<ul class="pagination">';
for ($page = 1; $page <= $totalPages; $page++) {
echo '<li';
if ($page === $currentPage) {
echo ' class="active"';
}
echo '><a href="?page=' . $page . '">' . $page . '</a></li>';
}
echo '</ul>';
In the above example, we display a simple list of data items and generate pagination links using an unordered list (<ul>
) with list items (<li>
). The current page number is passed as a URL parameter (?page=
), which allows users to navigate to different pages.
- CSS Styling (Optional):
Add CSS styles to the pagination links to make them visually appealing and indicate the active page.
That’s it! With these steps, you have implemented basic pagination in PHP. When users click on the pagination links, they will be directed to different pages, displaying the appropriate subset of data. Remember to adjust the $recordsPerPage
value according to your specific requirements, and consider using an appropriate front-end framework or library for more advanced pagination features and styling.