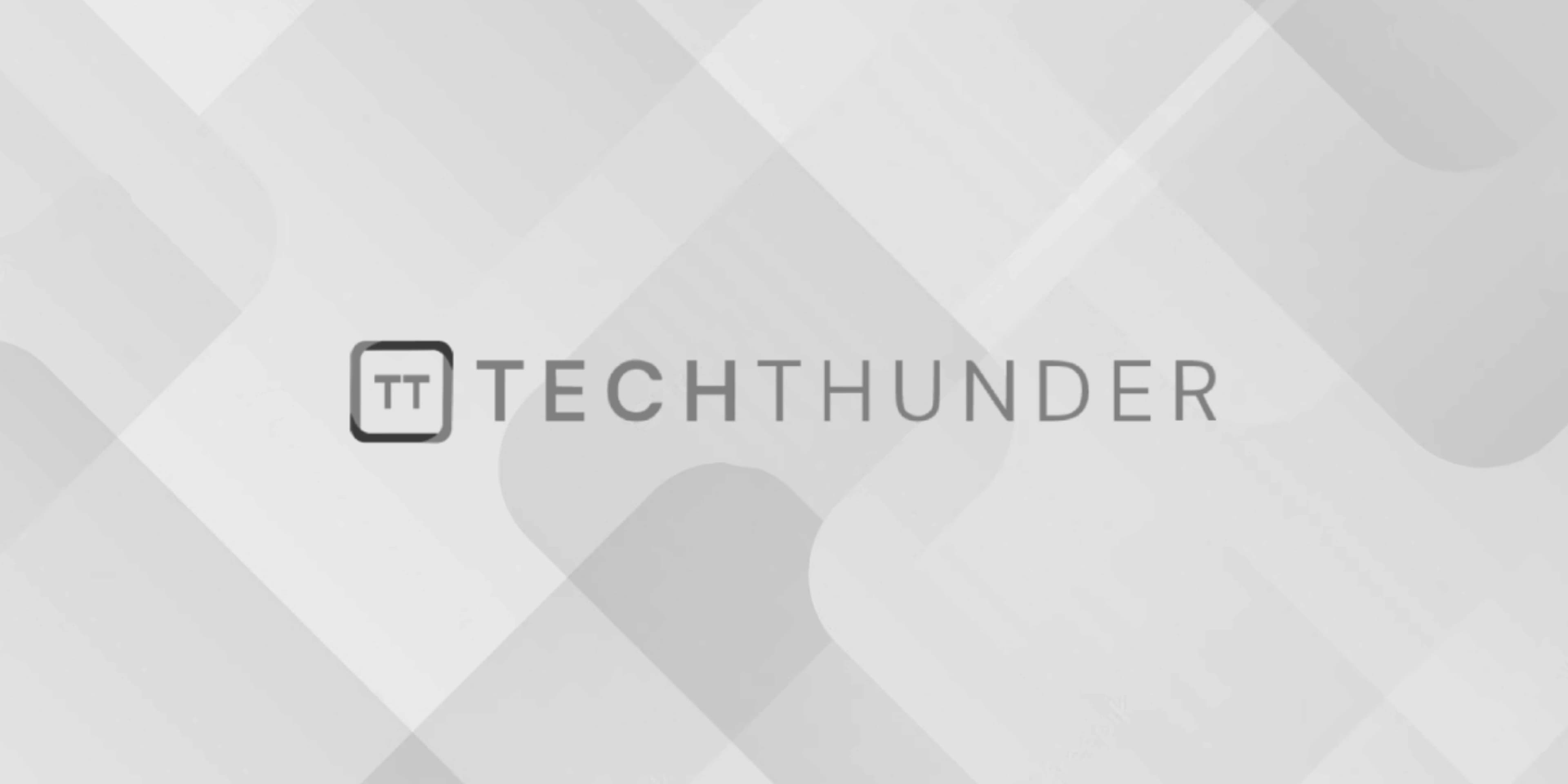
226 views
CRUD Operation using PHP & Mongodb
Performing CRUD (Create, Read, Update, Delete) operations using PHP and MongoDB involves connecting to the MongoDB server, selecting a database and a collection, and executing various MongoDB operations. Below is a basic example of how to perform CRUD operations using PHP and MongoDB:
- Install MongoDB PHP Extension:
Ensure that the MongoDB PHP extension is installed. You can install it using Composer:
composer require mongodb/mongodb
- Connect to MongoDB:
Create a PHP script (e.g.,connect.php
) to connect to the MongoDB server:
<?php
$mongoClient = new MongoDB\Client("mongodb://localhost:27017");
$database = $mongoClient->selectDatabase("mydatabase");
$collection = $database->selectCollection("mycollection");
?>
- Create (Insert) Operation:
<?php
require 'connect.php';
$data = [
'name' => 'John Doe',
'age' => 30,
'email' => '[email protected]'
];
$insertResult = $collection->insertOne($data);
if ($insertResult->getInsertedCount() === 1) {
echo "Document inserted successfully. Inserted ID: " . $insertResult->getInsertedId();
} else {
echo "Failed to insert document.";
}
?>
- Read Operation:
<?php
require 'connect.php';
$cursor = $collection->find([]);
foreach ($cursor as $document) {
echo "Name: " . $document['name'] . ", Age: " . $document['age'] . ", Email: " . $document['email'] . "<br>";
}
?>
- Update Operation:
<?php
require 'connect.php';
$filter = ['name' => 'John Doe'];
$updateData = ['$set' => ['age' => 31]];
$updateResult = $collection->updateOne($filter, $updateData);
if ($updateResult->getModifiedCount() === 1) {
echo "Document updated successfully.";
} else {
echo "Failed to update document.";
}
?>
- Delete Operation:
<?php
require 'connect.php';
$filter = ['name' => 'John Doe'];
$deleteResult = $collection->deleteOne($filter);
if ($deleteResult->getDeletedCount() === 1) {
echo "Document deleted successfully.";
} else {
echo "Failed to delete document.";
}
?>
Please make sure to replace “localhost:27017” with the appropriate MongoDB server address and port. Also, update “mydatabase” and “mycollection” with your desired database and collection names.
Ensure that you have MongoDB installed and running on your server before executing these PHP scripts. Additionally, consider implementing error handling and input validation to ensure the security and reliability of your application.