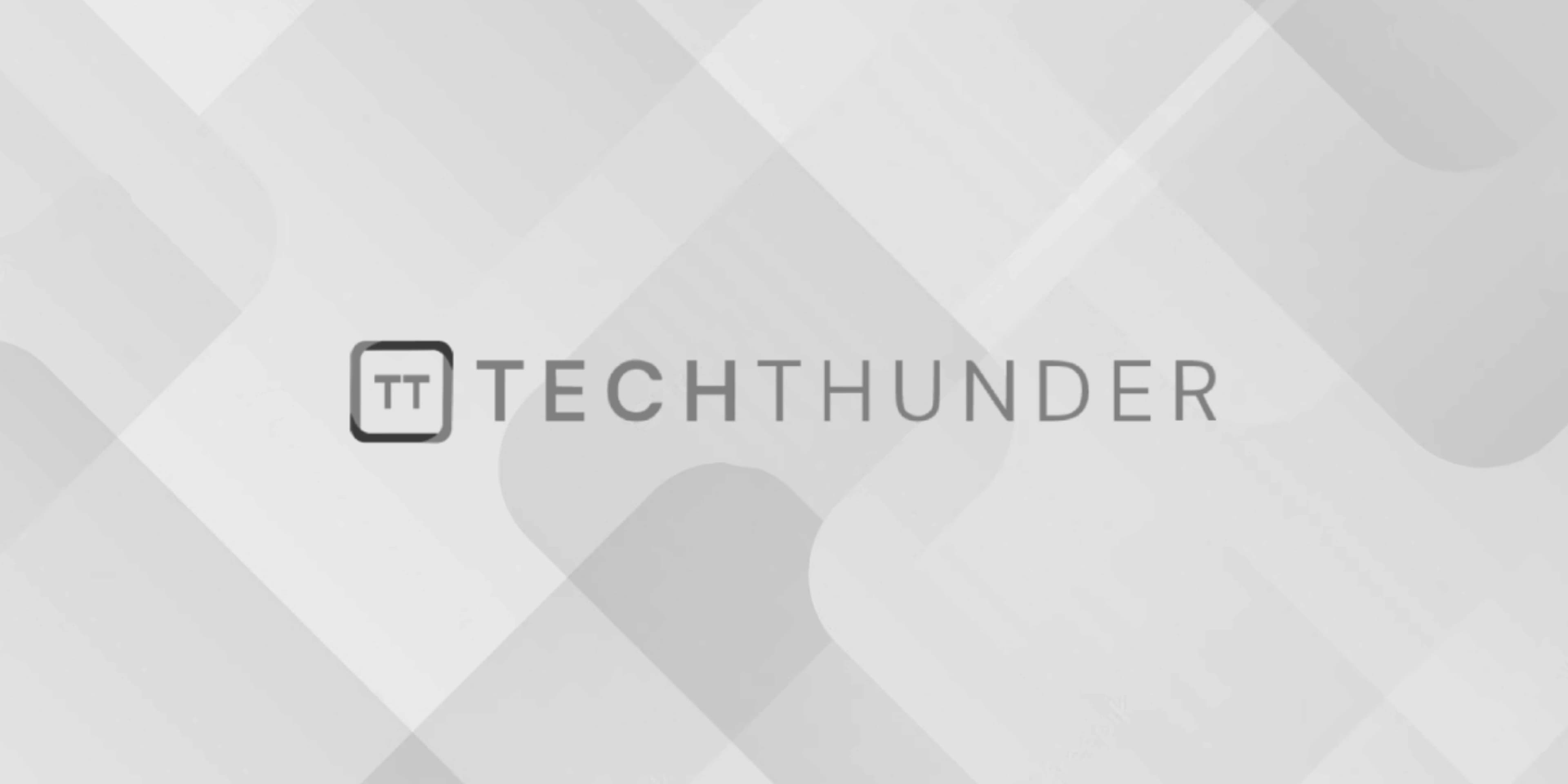
PHP foreach loop
In PHP, the foreach
loop is used to iterate over elements in an array or elements in an object. It provides an easy and convenient way to traverse through the items in a collection without the need to manually manage the index or iterator variable.
The basic syntax of the foreach
loop is as follows:
foreach ($arrayOrObject as $value) {
// Code to be executed for each element
}
Here’s how the foreach
loop works:
$arrayOrObject
: The array or object that you want to iterate over.$value
: A variable that represents the current value of the element in each iteration. You can choose any variable name you like for this.- Code Execution: The code block inside the
foreach
loop is executed for each element in the array or object. The$value
variable takes the value of each element in turn.
Example of a foreach
loop with an array:
$fruits = array("Apple", "Banana", "Orange", "Mango");
foreach ($fruits as $fruit) {
echo $fruit . " ";
}
Output:
Apple Banana Orange Mango
In this example, the foreach
loop iterates through each element in the $fruits
array, and in each iteration, the value of the current element is stored in the $fruit
variable, which is then echoed to the output.
Example of a foreach
loop with an associative array:
$studentScores = array(
"John" => 85,
"Alice" => 92,
"Bob" => 78,
"Eve" => 95
);
foreach ($studentScores as $name => $score) {
echo $name . ": " . $score . "\n";
}
Output:
John: 85
Alice: 92
Bob: 78
Eve: 95
In this example, the foreach
loop iterates through each key-value pair in the $studentScores
associative array. In each iteration, the key (student name) is stored in the $name
variable, and the value (student score) is stored in the $score
variable. The loop then echoes the name and score for each student.
The foreach
loop is especially useful for working with arrays and objects and is a cleaner and more concise way to iterate through their elements compared to using traditional for
or while
loops. It simplifies the process of iterating over collections and accessing their values.